JavaScript, being a versatile language, often encounters scenarios where data arrives in comma-separated strings. These strings might represent lists, items, or values that need to be processed individually. Here, we’ll learn about the techniques to convert comma separated strings to array in JavaScript.
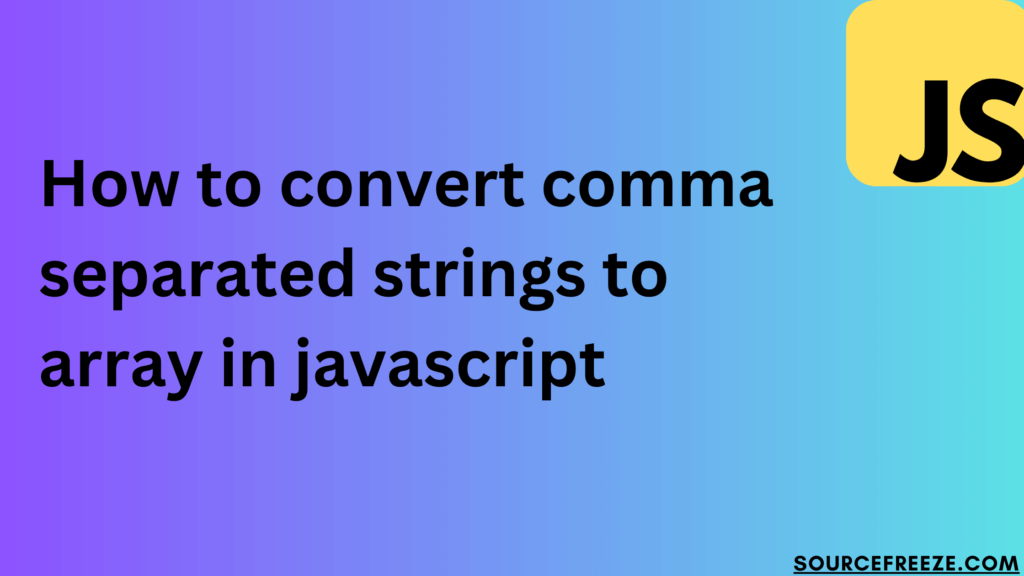
Let’s explore different approaches to accomplish this task:
Using the split() Method
The split()
method is a robust solution when dealing with simple comma-separated strings. It functions by breaking a string into substrings based on a specified separator and then returning an array containing these substrings.
Consider the string:
const commaSeparated = "apple,banana,orange";
To convert this string into an array of individual fruits, we employ the split() method:
const fruitsArray = commaSeparated.split(',');
console.log(fruitsArray); // Output: ['apple', 'banana', 'orange']
In this instance, split(‘,’) takes the comma as the separator and divides the string at each occurrence of the comma. This generates an array [‘apple’, ‘banana’, ‘orange’], allowing easy access to each fruit element.
An important consideration is handling cases where there might be empty elements between separators:
const mixedString = "apple,,orange";
const mixedArray = mixedString.split(',');
console.log(mixedArray); // Output: ['apple', '', 'orange']
In this scenario, split(‘,’) creates an array [‘apple’, ”, ‘orange’], including an empty string between the commas. It’s crucial to be aware of such occurrences when manipulating data.
Using Array.from() with split()
Array.from()
is a powerful method in JavaScript that creates a new array instance from an iterable object or array-like structure. When combined with the split()
method, it offers an alternative approach to handle comma-separated strings.
Consider the following scenario:
const commaSeparated = "apple,banana,orange";
Now, we can leverage Array.from() to convert this string into an array of individual fruits by first splitting it using split(‘,’):
const fruitsArray = Array.from(commaSeparated.split(','));
console.log(fruitsArray); // Output: ['apple', 'banana', 'orange']
Here’s the breakdown of this approach:
commaSeparated.split(‘,’) breaks the string at each comma, producing an array [‘apple’, ‘banana’, ‘orange’]. Array.from() then creates a new array from this array-like structure returned by split(‘,’), resulting in [‘apple’, ‘banana’, ‘orange’].
The versatility of Array.from()
extends beyond arrays. It can handle iterable objects, which means it can convert other iterable structures like strings into arrays.
Consider a scenario where we have a string containing characters that need to be separated:
const characters = "hello";
const charArray = Array.from(characters);
console.log(charArray); // Output: ['h', 'e', 'l', 'l', 'o']
Here, Array.from(characters) effortlessly converts the string “hello” into an array of individual characters, enabling easy manipulation or processing of each character.
Using slice() for String Manipulation
The slice() method in JavaScript is primarily used to extract a portion of an array and return it as a new array. While slice() is generally used with arrays, it can also be utilized in a unique way to handle comma-separated strings.
Let’s take a comma-separated string:
const commaSeparated = "apple,banana,orange";
By using slice() in combination with other string manipulation methods, such as split(), we can effectively convert this string into an array:
const fruitsArray = commaSeparated.slice().split(',');
console.log(fruitsArray); // Output: ['apple', 'banana', 'orange']
commaSeparated.slice()
returns a shallow copy of the original string. This step isn’t strictly necessary but demonstrates how slice() can be used in this context. split(',')
is then applied directly to the string. This function breaks the string into substrings at each comma, generating an array ['apple', 'banana', 'orange']
.
Conclusion
In this blog, we’ve explored various methods to convert comma-separated strings into arrays in JavaScript. Each method offers a unique approach to tackle this common task efficiently.
Throughout this discussion, we’ve covered:
- Using
split()
: The simplest and most straightforward method to split a string into an array based on a defined separator. - Leveraging
Array.from()
: A versatile approach to create arrays from iterable objects, such as the array returned bysplit()
. - Use of
slice()
: Demonstrating how slice() can assist in preliminary string manipulation before employing split() for array generation.
Recent Comments