JavaScript is a powerful language that enables dynamic manipulation of web pages. One of the fundamental tasks in front-end development involves modifying HTML elements dynamically. Adding classes to elements is crucial for styling and functionality. In this comprehensive guide, we’ll learn the process of adding class to an HTML element in javascript, exploring various methods and their applications.
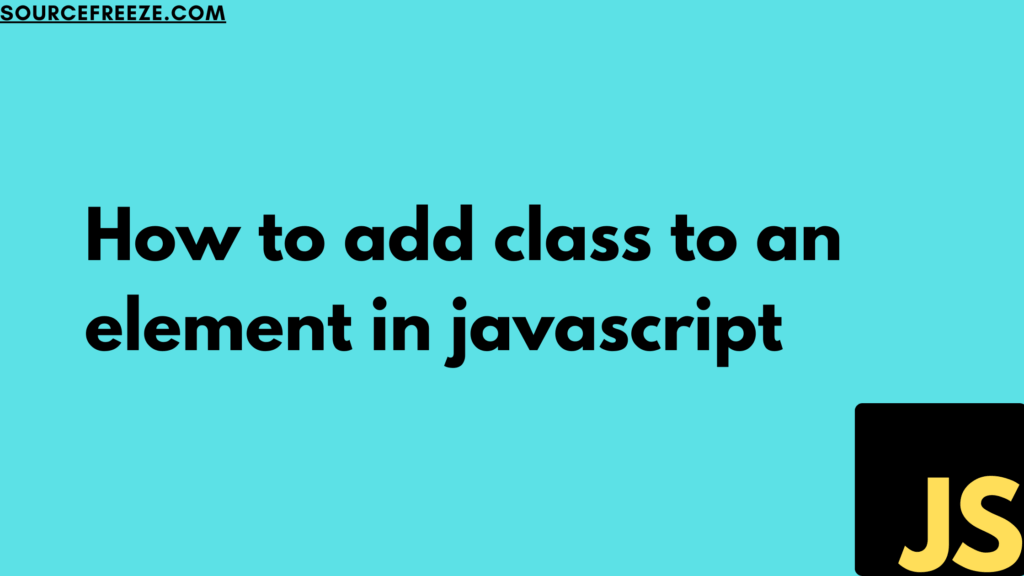
- Using classList Property: This method leverages the built-in
classList
property, offering a clean and straightforward approach to add classes to elements. - Using className Property: Another technique involves directly modifying the
className
property of an element, allowing concatenation of existing classes with new ones. - Using setAttribute Method: This method involves the
setAttribute
function, enabling the dynamic addition of a class to an element by explicitly setting its class attribute.
Throughout this blog, we’ll break down each method step-by-step, providing detailed code samples and explanations to help you grasp these concepts effectively. Starting with the first one:
Using classList Property
To add a class to an HTML element, one approach is leveraging the classList
property. This method is clean and straightforward, making it a popular choice among developers.
HTML Structure
Let’s start with a basic HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>Add Class to Element</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="targetElement">Some content here</div>
<script src="script.js"></script>
</body>
</html>
JavaScript Implementation
const element = document.getElementById('targetElement');
element.classList.add('newClass');
Explanation:
In the above code, we select the element by its ID using getElementById
. Then, the classList
property’s add()
method adds the specified class, ‘newClass’, to the element.
Detailed Code Breakdown
Let’s break this down further:
- const element = document.getElementById(‘targetElement’): Here, we’re selecting the HTML element with the ID ‘targetElement’ and storing it in the element variable.
- element.classList.add(‘newClass’): This line utilizes the classList property of the element, specifically the add() method, to add the class ‘newClass’ to the selected element.
Using className Property
Another approach to add a class is by directly modifying the className
property of the element.
HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>Add Class to Element</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="targetElement">Some content here</div>
<script src="script.js"></script>
</body>
</html>
JavaScript Implementation
const element = document.getElementById('targetElement');
element.className += ' newClass';
Explanation
In this method, we access the element using its ID and then concatenate the existing class with the new class, ‘newClass’, using the +=
operator.
Detailed Code Breakdown
- const element = document.getElementById(‘targetElement’);: This line selects the HTML element with the ID ‘targetElement’ and stores it in the element variable.
- element.className += ‘ newClass’;: Here, we append ‘ newClass’ to the existing classes of the element.
Using setAttribute Method
The setAttribute
method allows us to set an attribute for an HTML element. We can leverage this method to add a class dynamically.
HTML Structure (Same as Previous Examples)
<!DOCTYPE html>
<html>
<head>
<title>Add Class to Element</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="targetElement">Some content here</div>
<script src="script.js"></script>
</body>
</html>
JavaScript Implementation
const element = document.getElementById('targetElement');
element.setAttribute('class', 'newClass');
Explanation
In this method, setAttribute
is used to assign a class to the selected element. It takes two arguments: the attribute name (‘class’ in this case) and the value (‘newClass’).
Detailed Code Breakdown
- const element = document.getElementById(‘targetElement’);: Selects the HTML element with the ID ‘targetElement’ and stores it in the element variable.
- element.setAttribute(‘class’, ‘newClass’);: This line sets the ‘class’ attribute of the element to ‘newClass’.
Conclusion:
In this blog, we’ve explored three fundamental methods for adding classes to HTML elements using JavaScript. We’ve covered the usage of classList
, className
, and setAttribute
to dynamically apply classes to elements on a webpage.
By utilizing these methods, developers can enhance the interactivity and styling of web elements, ensuring a more efficient and seamless development process. Experimenting with these techniques will empower you to adding class to an HTML element in javascript.
Leave a Reply