In this guide, we’ll see various methods to get Scroll Position in React. From leveraging refs to target elements to scrolling based on specific coordinates, each method will explain to you the scroll behavior in JavaScript within your React applications.
Let’s explore these strategies in detail, understanding their implementation and applicability to create seamless scrolling experiences for your users:
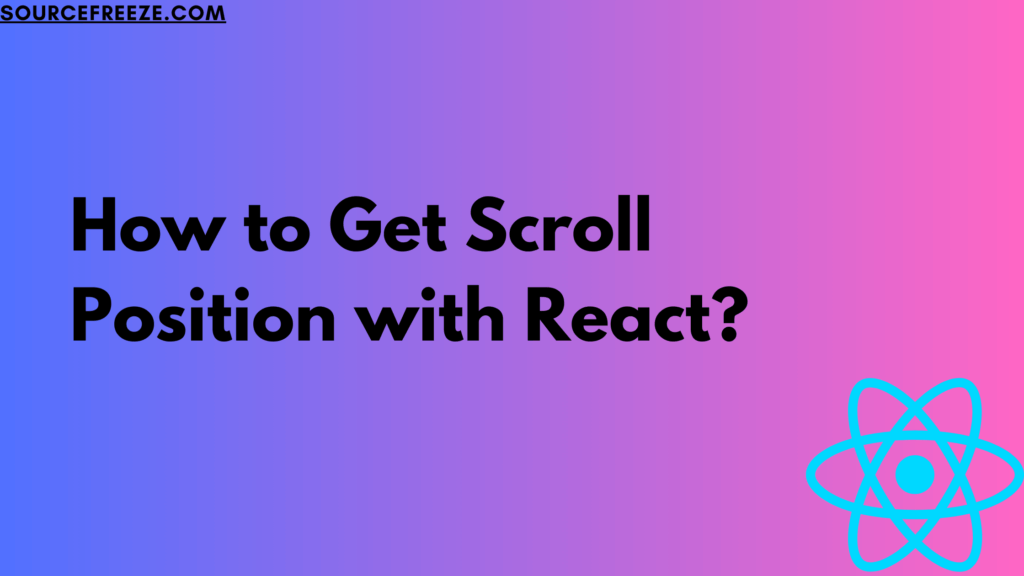
Let’s start by addressing the idea of setting scroll positions to specific elements.
Setting Scroll Position to Elements:
When it comes to setting scroll positions to particular elements in React, it’s crucial to establish a reference to that specific DOM element. In React, we utilize refs instead of the traditional getElementById()
from vanilla JavaScript.
Using Refs to Set Scroll Position
To target an element for scroll positioning, we’ll create a ref using the useRef() hook:
import { useRef } from 'react';
export default function App() {
const targetElement = useRef();
// ... rest of the component
}
In the JSX, assign this ref to the element you want to set the scroll position for:
<p ref={targetElement}>Target Element</p>
Now, this targetElement
ref holds a reference to the specified <p>
element, enabling us to manipulate its scroll position.
Scroll Position on Button Click
Let’s set up functionality to trigger scroll to this element upon a button click:
import { useRef } from 'react';
export default function App() {
const targetElement = useRef();
const scrollToElement = () => {
window.scrollTo({
top: targetElement.current.offsetTop,
behavior: 'smooth',
});
};
return (
<div className="App">
<button onClick={scrollToElement}>Scroll to Target Element</button>
<p ref={targetElement}>Target Element</p>
</div>
);
}
This code sets up a button that, when clicked, will smoothly scroll the viewport to the specified element.
Scrolling to Coordinates
Alternatively, we can scroll to specific coordinates within the window:
export default function App() {
const scrollToCoordinates = () => {
window.scrollTo(0, 1000); // Scroll to X: 0, Y: 1000
};
return (
<div className="App">
<button onClick={scrollToCoordinates}>Scroll to 1000px</button>
<h2 style={{ marginTop: 1000 }}>We've scrolled 1000 pixels</h2>
</div>
);
}
This code demonstrates scrolling to a specific Y coordinate when the button is clicked.
Conclusion
These methods showcase different ways to get Scroll Position in React, whether it’s targeting specific elements or scrolling to specific coordinates within the viewport.
From harnessing refs to target and smoothly scroll to specific elements to manipulating scroll coordinates directly, each technique offers a nuanced approach to controlling user scroll experiences.
Leave a Reply