In this tutorial, we will see how to redirect to another page in next js, there are three ways to redirect one page to another page in nextjs.
- Using Link in Next.js
- useRouter() Hook by using router.push
- config.next.js
- Middleware
Let’s see each option in a detailed way.
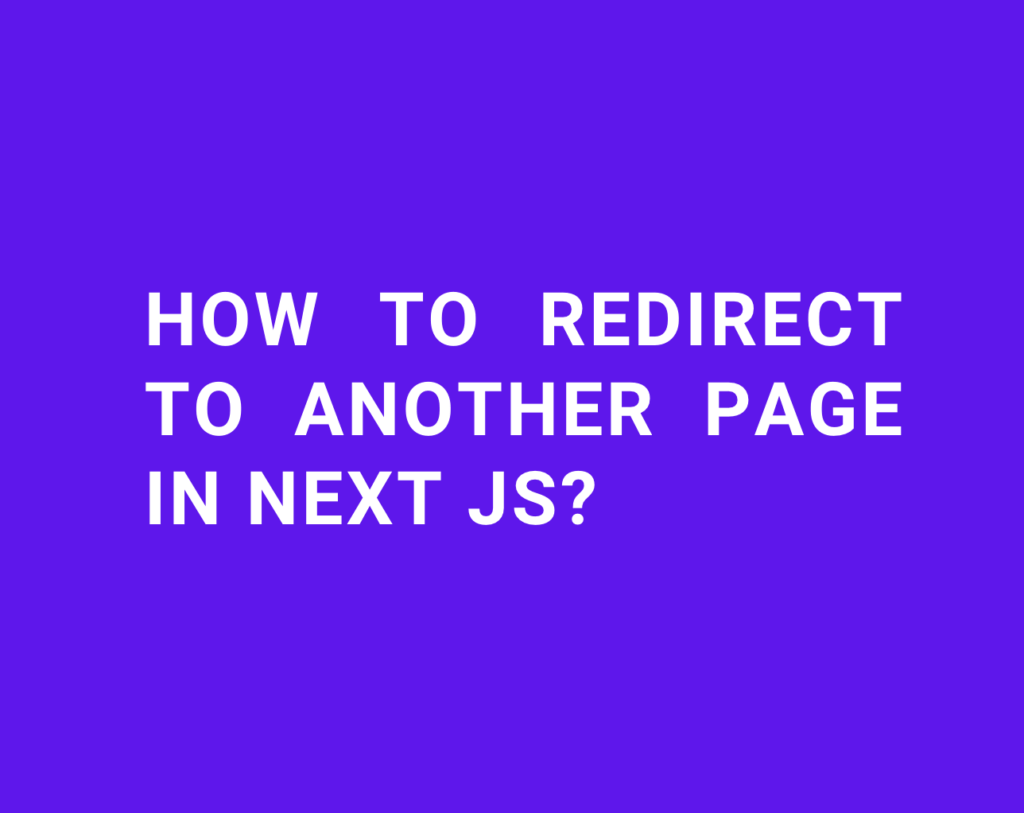
Using Link Component in Next.JS
Using Link component in nextjs it is like a href tag you can just use the href attribute and mention the redirect URL, please refer the below example.
First we need import the Link
from the next/link
module
import Link from 'next/link';
// ...
return (
<div>
<Link href="/new-url">
<a>Click here to redirect</a>
</Link>
</div>
);
How to redirect to another page using useRouter() Hook onclick
We can use the useRouter
hook from next/router
to get access to the router object in your functional component, and then we can use the push method to navigate to another page on the buttonn onclick event.
Let’s see an example using userRouter
in the below.
import { useRouter } from 'next/router';
function MyComponent() {
const router = useRouter();
const handleClick = () => {
router.push('/new-page');
};
return (
<button onClick={handleClick}>Go to new page</button>
);
}
While clicking the Go to new page button, it will navigate to the /new-page page.
We can also use the router.push
method to pass query parameters to the new page, as mentioned in the below example.
import { useRouter } from 'next/router';
function MyComponent() {
const router = useRouter();
const handleClick = () => {
router.push({
pathname: '/new-page',
query: {
name: 'Source Freeze',
count: 30,
},
});
};
return (
<button onClick={handleClick}>Go to new page</button>
);
}
This will navigate to the /new-page page and pass the query parameters name and count to it.
How to redirect to another page using config.next.js
We can redirect a one to another page using config.next.js but this is fixed redirect mostly it is used for ther permanent redirect it is not a logic based, in some cases you need to get the benefit SEO for the redirection or permanent redirection we will use this approach, please refer the below to redirect to another page using config.next.js
/** @type {import('next').NextConfig} */
const nextConfig = {
async redirects() {
return [
{
source: '/old-page',
destination: '/',
permanent: true,
},
]
},
}
module.exports = nextConfig
Middleware
Next we can achive the redirection using the Middleware, this middleware redirection we can use the conditional based redirection.
Please refer the beloe example.
import { NextResponse } from 'next/server'
export function middleware(request) {
if ( request.id === 1 ) {
return NextResponse.rewrite(new URL('/page-1', request.url))
} else if (request.id === 2) {
return NextResponse.redirect(new URL('/page-2', request.url))
} else {
return NextResponse.rewrite(new URL('/not-found', request.url))
}
return NextResponse.next()
}
Here in this tutorial we have seen all the options of redirect to another page in next js.
Thanks for reading also you can refer the below tutorial how to get the query params from the URL in NextJS
Leave a Reply