JavaScript, being a versatile language, often requires us to identify whether an object behaves like a Promise. Promises are essential for handling asynchronous operations, and recognizing them accurately becomes crucial in programming.
In this blog, we’ll delve into four simple methods that help us to check if object is a promise in javascript:
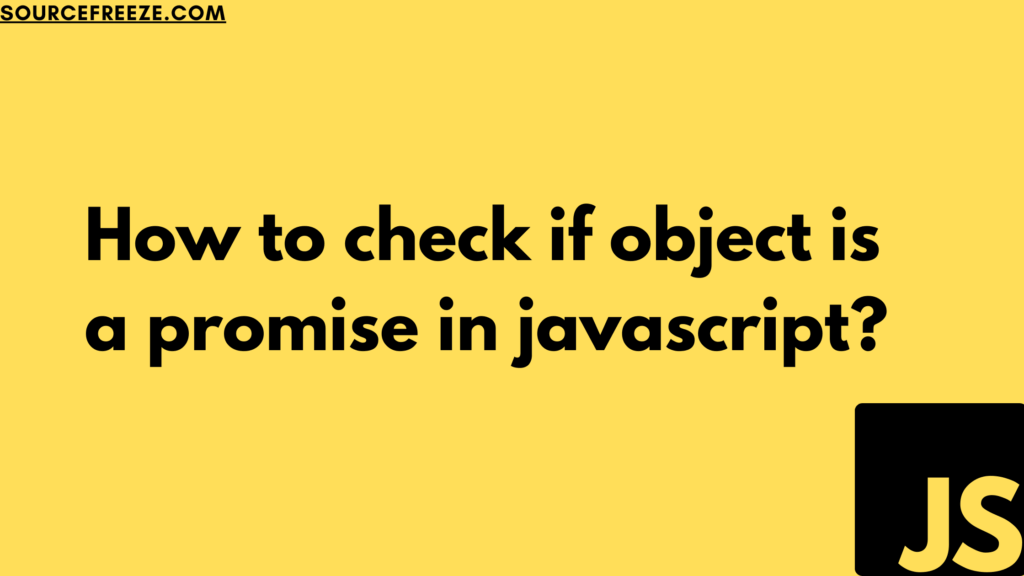
- Checking via typeof Function: This method involves examining whether the typeof the object’s .then property is a function.
- Utilizing
Object.prototype.toString.call()
: Here, we’ll explore how to use Object.prototype.toString.call() to check if the object’s type is “[object Promise]”. - Using Promise.resolve(): This method revolves around the Promise.resolve() function, attempting to resolve the given object into a Promise object for comparison.
- Checking Object Type and .then Property: This method combines checking the object’s type and its .then property to determine if it behaves like a Promise.
Checking via typeof Function:
In JavaScript, one method to determine if an object is a promise is by checking if the typeof
the object’s .then
property is a function. This method involves using the typeof
operator to ascertain the nature of the object’s .then
property.
To implement this, we will first use the typeof operator to check if the object has a .then property and if it’s a function. This approach is quite straightforward and widely used:
function isPromise(value) {
return typeof value.then === 'function';
}
// Usage:
const someObject = /* your object */;
if (isPromise(someObject)) {
// It's a Promise!
} else {
// It's not a Promise!
}
This method evaluates the type of the .then
property within the object to determine if it adheres to the expected behavior of a promise, specifically checking if it is a function.
Here’s an example illustrating this approach:
Consider a scenario where we’re fetching data from an API. We’ll use the typeof
function to check if the retrieved data behaves like a Promise:
function fetchDataFromAPI() {
return fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
if (typeof data.then === 'function') {
// Data is a Promise!
return data;
} else {
// Data is not a Promise!
// Handle non-promise data here...
}
});
}
Utilizing Object.prototype.toString.call()
Another effective way to ascertain whether an object is a Promise involves using Object.prototype.toString.call()
method. By calling this method on the object in question, we can check if the type is “[object Promise]”.
Here’s an example illustrating this approach:
Suppose we’re working with a function that returns a Promise. We’ll use Object.prototype.toString.call() to verify the returned value as a Promise:
function asyncOperation() {
return new Promise((resolve, reject) => {
// Asynchronous operations here...
resolve('Operation completed!');
});
}
const result = asyncOperation();
if (Object.prototype.toString.call(result) === '[object Promise]') {
// Result is a Promise!
} else {
// Result is not a Promise!
// Handle non-promise result here...
}
This method utilizes the Object.prototype.toString.call()
function, which provides a string representation of the object’s type. By comparing it against “[object Promise]”, we can effectively identify if the object is a Promise.
Using Promise.resolve():
Another way to verify if an object is a Promise involves the Promise.resolve()
function. This function attempts to resolve the given object into a Promise object. We can then compare the resolved object with the original to determine if they match.
Here’s an example illustrating this approach:
Imagine a scenario where we have an unknown value and need to check if it behaves like a Promise:
function processData(value) {
if (Promise.resolve(value) === value) {
// Value behaves like a Promise!
return value.then(data => {
// Process resolved data...
});
} else {
// Value is not a Promise!
// Handle non-promise value here...
}
}
In this method, Promise.resolve(value) tries to convert the value into a Promise. If the original object and the resolved object are the same, it suggests that the object was already a Promise, as the resolved Promise maintains its identity.
Checking Object Type and .then Property
Another approach involves checking if the object is an instance of the Object class and if it contains a .then
property that is a function. This combines checking the object’s type and its .then
property to ascertain if it behaves like a Promise.
function isPromise(obj) {
return obj instanceof Object && typeof obj.then === 'function';
}
// Usage:
const someObject = /* your object */;
if (isPromise(someObject)) {
// It's a Promise!
} else {
// It's not a Promise!
}
This method examines whether the given object is an instance of the Object class and simultaneously checks if it possesses a .then property of type function, resembling the behavior expected from a Promise.
Conclusion:
In this blog, we’ve explored four distinct methods to identify whether an object behaves like a Promise in JavaScript. By leveraging these methods:
- We’ve learned to check if an object’s .then property is a function using the typeof operator.
- Utilized Object.prototype.toString.call() to verify an object’s type as a Promise.
- Employed Promise.resolve() to resolve an object into a Promise for comparison.
- Combined object type and the presence of a .then property to identify Promise-like behavior.
These methods offer flexible approaches to determine Promise behavior, providing essential tools for handling asynchronous operations effectively in JavaScript.
Leave a Reply