Showing or Hide keyboard in react native is essential especially if you are working on the text input control, some times we are facing issues while dismissing or hiding the keyboard in react native this tutorial will explain how to dismiss the keyboard in react native, also will into how to hide or dismiss the keyboard button press or outside click or in the scroll view.
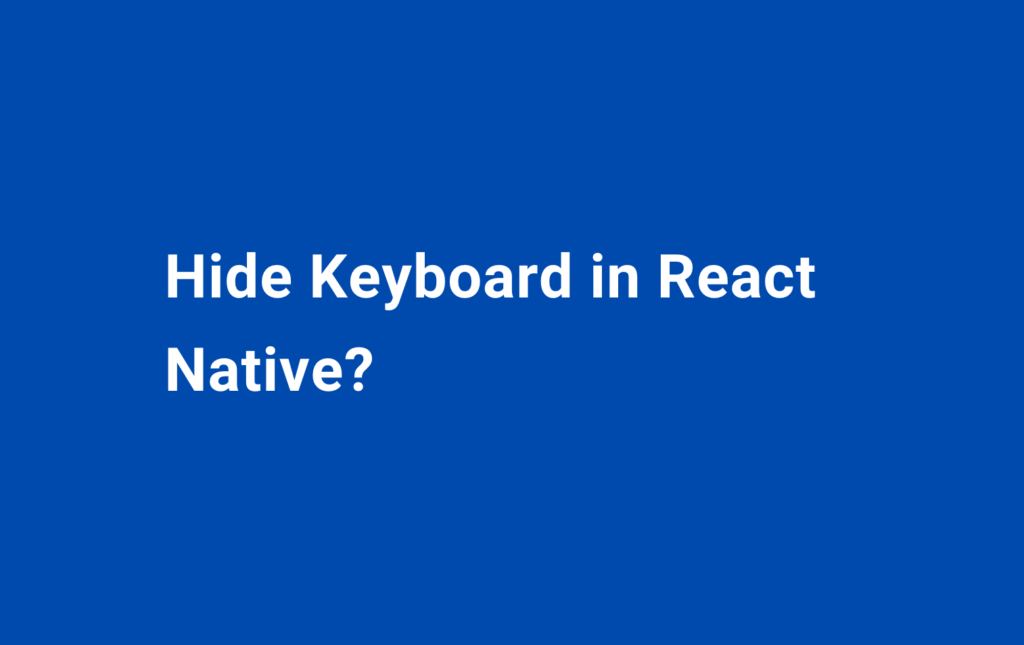
Hide Keyboard In React Native
To hide the keyboard in a React Native app, you can use the Keyboard component from the react-native library. Here’s an example of how to use it:
First, we need to import the Keyboard component like below.
import { Keyboard } from 'react-native';
Then, to hide or dismiss the keyboard, we can call the Keyboard.dismiss() method. This can be done in a number of ways, such as when a user clicks a button or any action click we can do it.
Here’s an example of how to hide the keyboard in react native when a button is clicked:
<Button
title="Hide Keyboard"
onPress={() => Keyboard.dismiss()}
/>
Alternatively, we can use the KeyboardAvoidingView
component to automatically adjust the layout of your app when the keyboard is visible. This can be useful if you want to ensure that certain UI elements, such as text input fields, are always visible while the keyboard is open
To use the KeyboardAvoidingView
, you’ll need to wrap the content that you want to adjust in a KeyboardAvoidingView
component and specify the behavior you want. For example:
<KeyboardAvoidingView behavior="padding" style={styles.container}>
<Text> Context Area </Text>
</KeyboardAvoidingView>
Hide or Dismiss the Keyboard In React Native while clicking outside of the view:
To hide or dismiss the keyboard in a React Native app when a user clicks outside of a specific view, you can use the TouchableWithoutFeedback
component from the react-native
library. This component allows you to specify an area of the screen that should not trigger touch events, such as dismissing the keyboard.
import the TouchableWithoutFeedback like below.
import { TouchableWithoutFeedback, Keyboard } from 'react-native';
Then, you can wrap the content that you want to be “touchable” in a TouchableWithoutFeedback
component, and specify the onPress
event to dismiss the keyboard. For example:
<TouchableWithoutFeedback onPress={() => Keyboard.dismiss()}>
<View style={styles.container}>
<View>
<Text> Content Area </Text>
<View>
</View>
</TouchableWithoutFeedback>
This will dismiss the keyboard whenever the user clicks anywhere within the View
component.
Also, you can use ScrollView
with keyboardShouldPersistTaps='handled
‘ to only hide or dismiss the keyboard.
I think we have covered all the aspects of how to dismiss the keyboard in react native, please let us know if any questions or clarifications in the comments below. Thanks.
Also, take a look at How to insert a line break into a Text component in React Native? in below.
Leave a Reply