In JavaScript, the colon (:) serves multiple purposes across different contexts, primarily as a separator and a syntax element within the language. Let’s dive into its key roles:
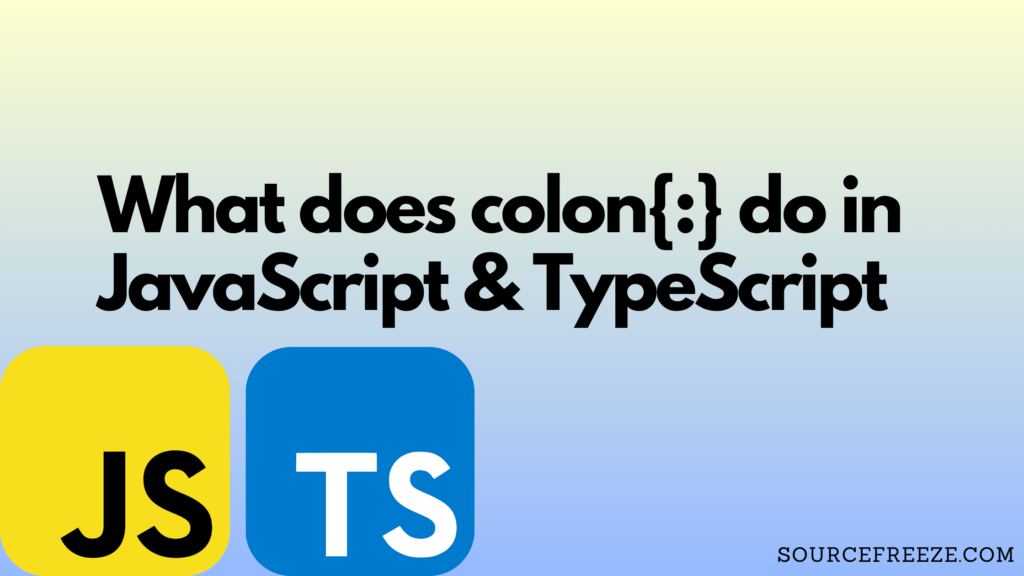
Here’s a list of the functionalities that a simple colon {:} provides us:
1. Using Colon in the Object Literal Syntax
2. Using Colon in the Ternary Operator
3. Using Colon in the Labeling Statements
4. Using Colon in the Object Destructuring
5. Using Colon in the switch Statement
6. Using Colon in the ES6 Method Shorthand
7. Using Colon in the TypeScript Type Definition
Let’s take a deeper dive into all these uses:
1. Colon in the Object Literal Syntax:
In JavaScript, the colon is instrumental in defining key-value pairs within object literals:
let person = {
name: "Jacob",
age: 30,
city: "Sydney"
};
Here, the colon acts as a separator, associating property keys (e.g., name) with their corresponding values (e.g., “Jacob”).
2. Colon in the Ternary Operator:
Within conditional statements, the colon is an integral part of the ternary operator, enabling concise conditional expressions:
let status = (age >= 18) ? "Adult" : "Minor";
The colon separates the true and false expressions. If the condition (age >= 18) holds true, status receives the value “Adult”; otherwise, it’s “Minor”.
Output: Depending on the value of age, the output for status will be either “Adult” or “Minor”.
3. Colon in the Labeling Statements:
Though less common, labels in conjunction with the colon (:) allow developers to mark specific statements, often used in complex nested loops, enabling control over loop flow.
Consider this example:
outerLoop: for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (i === 1 && j === 1) {
console.log("Break outer loop");
break outerLoop;
}
}
}
Here, the outer loop: acts as a label marking the outer loop. The break outer loop; statement within the nested loop structure signals to exit of both loops once a certain condition is met.
Two key things are happening here. Let’s understand better;
- Labeling Syntax:
- outer loop: marks the beginning of the outer loop. This label can be any valid identifier followed by a colon (:).
- break outer loop; is used to break out of the labeled loop when a specific condition (i === 1 && j === 1) is satisfied.
- Controlled Loop Termination: When the condition within the nested loops (i === 1 && j === 1) evaluates to true, the break outer loop; statement causes an immediate exit from the outer loop, bypassing further iterations. Without this labeled statement, breaking out nested loops simultaneously becomes challenging.
Output:
Break outer loop
4. Colon in the Object Destructuring:
During object destructuring, the colon facilitates property renaming:
let user = { firstName: 'Rachel', lastName: 'Green' };
let { firstName: fName, lastName: lName } = user;
Here, firstName: fName renames the property firstName to fName during the assignment.
Result: The renamed properties fName and lName will hold the values of firstName and lastName from the user object.
5. switch Statement
The switch statement in JavaScript employs the colon (:) in combination with the case and default keywords to execute specific code blocks based on the comparison of a given expression’s value.
switch (color) {
case 'red':
console.log('The color is red');
break;
case 'blue':
console.log('The color is blue');
break;
default:
console.log('The color is neither red nor blue');
}
Result: Based on the value of the expression within the switch statement, the corresponding case block or default block is executed, producing different output messages depending on the matched condition.
6. Colon in the ES6 Method Shorthand:
ES6 introduced concise object method shorthand using the colon:
let obj = {
name: "James",
greet() {
return `Hello, ${this.name}!`;
}
};
Here, greet() is a method defined using the concise ES6 syntax.
Result: The method greet() will output a personalized greeting based on the name property within the obj.
7. Colon in the TypeScript Type Definition:
In TypeScript, colon usage extends to type definitions during object initialization:
let user: { name: string, age: number } = {
name: "Monica",
age: 28
};
Here, the colon specifies the types for the name and age properties, ensuring strict typing for objects.
Now, the TypeScript compiler will ensure that the name should be a string and age should be a number for objects conforming to this structure.
To Sum it all:
The colon in JavaScript exhibits incredible versatility, serving as a critical syntax element in defining object properties, conditional operations, label statements, object destructuring, ES6 method shorthand, and TypeScript type definitions. Its multifaceted applications significantly impact JavaScript’s readability, structure, and functionality, empowering developers with a diverse set of tools for effective coding practices.
Checkout more from Source Freeze here!
Recent Comments