In web development, manipulating styles dynamically using JavaScript can be really handy. Let’s explore how to create a style tag using JavaScript in a web page to add styles using 2 different methods:
- Using document.createElement()
- Using insertAdjacentHTML()
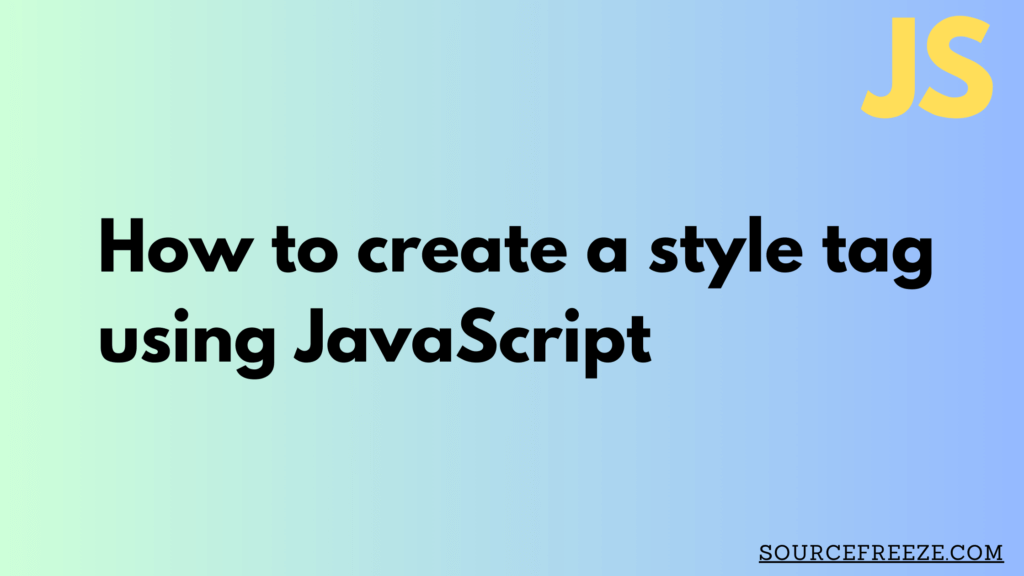
Creating a Style Tag in JavaScript using document.createElement():
Creating a style tag dynamically using JavaScript grants us the power to alter CSS properties programmatically.
// Step 1: Create a new style element
const styleTag = document.createElement('style');
// Step 2: Add CSS rules to the style element
styleTag.innerHTML = `
.classname {
color: blue;
font-size: 18px;
/* Add more CSS rules here */
}
`;
// Step 3: Append the style tag to the document's head
document.head.appendChild(styleTag);
This code snippet showcases a simple way to generate a style tag dynamically. Let’s break down each step:
- Creating the Style Element: We use
document.createElement()
to generate a new style element.
- Adding CSS Rules: The
innerHTML
property is utilized to define CSS rules within the<style>
tags. In this example, we’re setting text color to blue and font size to 18 pixels. You can include any CSS rules needed.
- Appending to the Document’s Head: To apply these styles, the newly created style tag is appended to the
<head>
of the HTML document usingappendChild()
.
By following the steps outlined above, we can manipulate styles programmatically, let’s dive deeper into the insertion of HTML adjacent to an element using the insertAdjacentHTML()
method in JavaScript.
Adding a Style Tag with Styles using insertAdjacentHTML()
The insertAdjacentHTML()
method comes in handy when we need to dynamically inject HTML content adjacent to an existing element. This method offers four different positions for insertion:
'beforebegin'
: Inserts content before the targeted element.'afterbegin'
: Inserts content at the beginning of the targeted element, within it.'beforeend'
: Inserts content at the end of the targeted element, within it.'afterend'
: Inserts content after the targeted element.
Let’s explore how we can use this method in conjunction with JavaScript:
Step 1: Selecting the Target Element
Firstly, we need to identify the element after which we want to insert the style tag. This can be done by selecting an element using its ID, class, or any other selector.
const targetElement = document.getElementById('target');
Replace 'target'
with the ID of the element where you want to insert the style tag.
Step 2: Defining CSS Rules
Next, we create the CSS rules that we want to apply dynamically. These rules will define the appearance or behavior of elements on the webpage.
const cssRules = `
.classname {
color: red;
font-size: 20px;
/* Add more CSS rules here */
}
`;
You can modify these rules by changing properties like color, font size, or any other CSS properties you want to apply.
Step 3: Creating the Style Tag
Now, let’s create the <style>
tag dynamically by combining the CSS rules within it.
const styleHTML = `<style>${cssRules}</style>`;
This code combines the CSS rules inside <style>
tags, creating a complete style tag with the defined styles.
Step 4: Inserting the Style Tag
Finally, we’ll use the insertAdjacentHTML()
method to insert the newly created style tag with styles after the selected target element.
targetElement.insertAdjacentHTML('afterend', styleHTML);
This line of code tells the browser to place the generated <style>
tag containing our CSS rules just after the target element.
This approach allows us to dynamically inject CSS rules into our webpage, letting us change the appearance or behavior of elements on the fly without modifying the HTML or CSS files directly.
Conclusion:
In this exploration, we’ve delved into two powerful methods in JavaScript for dynamically manipulating elements within a webpage: creating a style tag using document.createElement() and the insertAdjacentHTML()
method.
Recent Comments