If you’re new to JavaScript and see the “Function is not defined” message while using the onclick event in JavaScript, don’t worry—it’s common and fixable! This error pops up when trying to use a function that either doesn’t exist or can’t be found where it is needed.
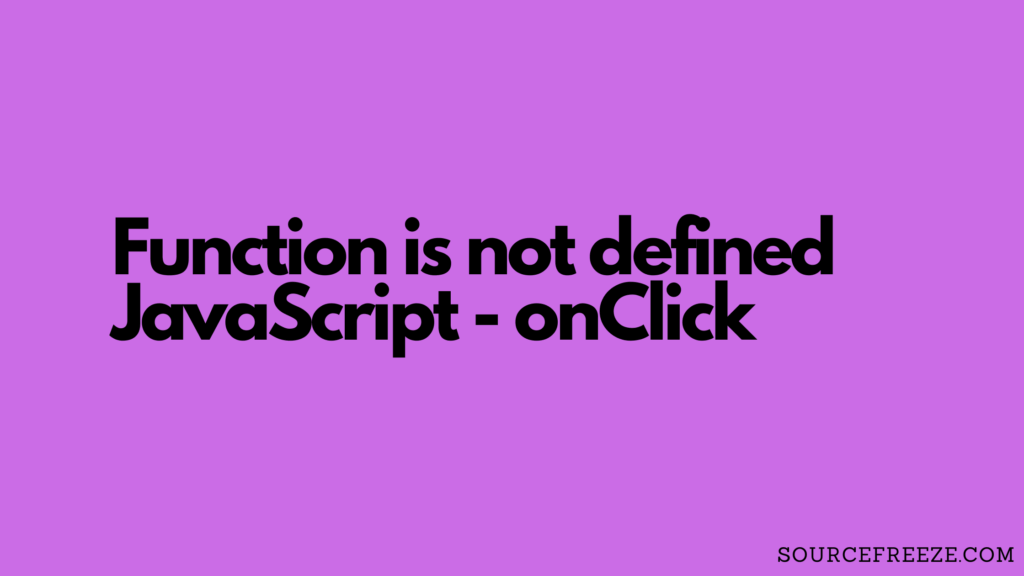
Understanding the Problem
Let’s break it down: when you click a button (thanks to the onclick attribute), JavaScript looks for a function to execute. But if it can’t find that function, it throws the “Function is not defined” error.
Identifying the cause:
Checking Function Names:
Ensure consistency in your function names—JavaScript distinguishes between uppercase and lowercase letters. Make certain that your function names are accurately spelled and consistently referenced throughout your codebase.
Function Placement:
Place your functions appropriately within your code structure. If they’re nested within other functions or hidden away in scopes, they might not be accessible when required. Position them where your entire codebase can access them without constraints.
Timing of the function definition:
For JavaScript to recognize your functions when needed, it is essential the functions defined before usage. If your functions reside in an external JavaScript file, ensure that this file is loaded before invoking the functions. In the case of functions in HTML, position them before the sections where they’re called upon.
Separate Files Verification:
If your functions exist in a separate JavaScript file, conduct a thorough check to ensure its proper linkage within your HTML document. Review the file’s location and inspect it for any potential mistakes that could impede its connection.
HTML Reminder:
When utilizing the onclick attribute, only specify the function name without parentheses or arguments. Maintain the format like this: onclick=”firstFunction()”.
Example Code Walkthrough:
Here’s a simple example that triggers the “Function is not defined” error:
<!DOCTYPE html>
<html>
<head>
<title>Resolving Function is not defined</title>
<script>
function firstFunction() {
alert('Clicked First Button');
}
</script>
</head>
<body>
<button onclick="firstFunction()">First Button</button>
<button onclick="secondFunction()">Second Button</button>
</body>
</html>
I’m sure this is the message you’d be seeing in the console (Click Ctrl + Shift + J to view console):
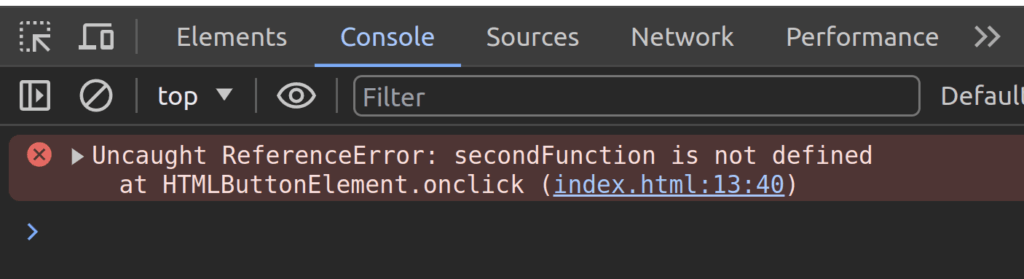
Solving the Issue:
Adding the missing function resolves the error. Here’s the fixed code:
<script>
function firstFunction() {
alert('Clicked First Button');
}
function secondFunction() {
alert('Clicked Second Button');
}
</script>
In Conclusion:
The “Function is not defined” error is a common issue in JavaScript, especially when handling onclick events. By following these steps and making sure your functions are in the right place, you’ll solve this issue like a pro!
Feel free to ask any questions or share thoughts on this topic!
Check out more from Source Freeze here!
Recent Comments