JavaScript offers versatile tools to handle dates and times. Converting milliseconds to a date is essential in various applications, from data processing to user interface interactions. We’ll explore two primary approaches to convert Milliseconds to a Date using JavaScript:
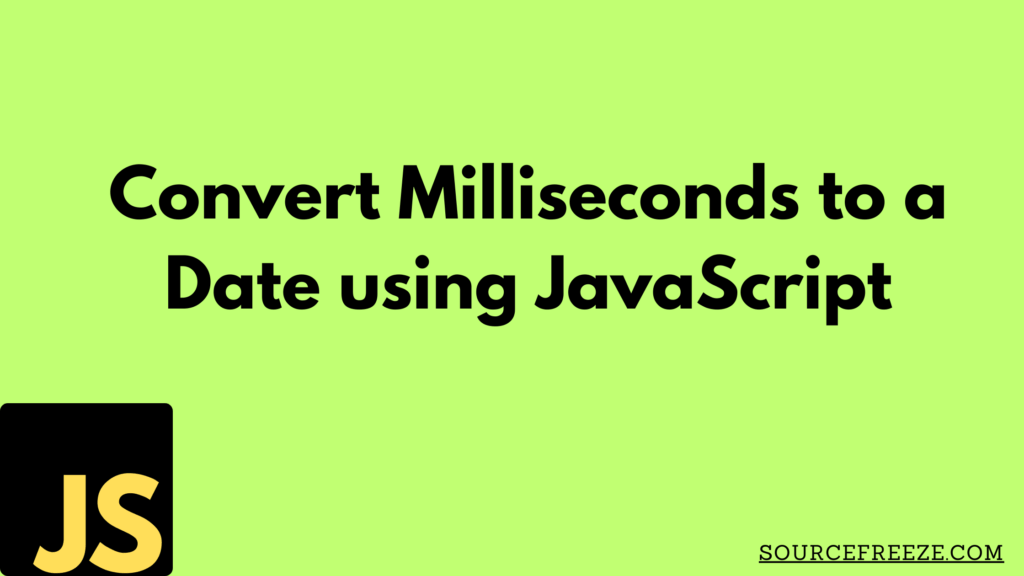
Let’s start by using the new Date() method to convert milliseconds to a date representation.
Exploring new Date() Method:
const milliseconds = 729459245971; // Example millisecond value
const date = new Date(milliseconds);
console.log(date);
Output:
1993-02-11T19:34:05.971Z
In this example, we assign the number of milliseconds to the milliseconds variable and then use new Date() to create a Date object.
We can convert the date in a human-readable string format using toString()
:
const milliseconds = 729459245971; // Example millisecond value
const date = new Date(milliseconds);
console.log(date.toString());
Output
Thu Feb 11 1993 19:34:05 GMT+0000 (Coordinated Universal Time)
Extracting specific components:
The new Date()
method offers simplicity and efficiency in converting milliseconds to a date. Additionally, by employing methods like getMonth()
, getDate()
, and getFullYear()
, we can extract specific date components from the resultant Date object.
const milliseconds = 729459245971; // Example millisecond value
// Accessing specific date components
const date = new Date(milliseconds);
const year = date.getFullYear();
const month = date.getMonth() + 1; // Adding 1 because months are zero-indexed
const day = date.getDate();
console.log(`${day}-${month}-${year}`);
Output:
11-2-1993
By using getFullYear(), getMonth(), and getDate(), we retrieve the year, month, and day from the Date object, respectively. Adjustments are made to the month value (+1) to match the conventional numbering of months.
Manipulating Date Elements:
Let’s explore an example utilizing HTML and JavaScript to convert a predefined millisecond value into a readable date format upon a button click:
<!DOCTYPE html>
<html>
<head>
<title>Convert Milliseconds to Date</title>
</head>
<body>
<h2>Convert Predefined Milliseconds to Date</h2>
<button onclick="displayDate()">Click to get date</button>
<p id="milliseconds" style="font-size: 15px;"></p>
<p id="convertedDate" style="font-size: 15px;"></p>
<script>
var milliseconds = 729459245971; // Predefined milliseconds value
function displayDate() {
var date = new Date(milliseconds);
document.getElementById('convertedDate').innerHTML = date.toString();
}
// Display milliseconds value
document.getElementById('milliseconds').innerHTML = "Milliseconds = " + milliseconds;
</script>
</body>
</html>
This HTML document includes a button triggering the displayDate()
function when clicked. The function uses the predefined millisecond value to generate the corresponding date and displays it on the webpage.
Output:
Here’s how the HTML page would look like:
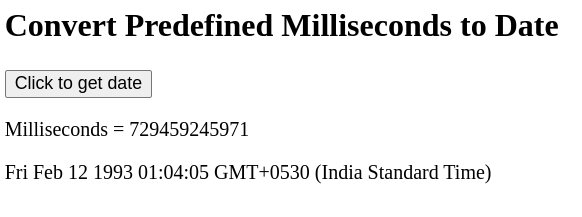
Conclusion:
In conclusion, we’ve explored two straightforward methods in JavaScript to effortlessly convert milliseconds into easily readable date formats. By using the new Date()
method and crafting custom functions with HTML, we’ve learned practical ways to handle date conversions, empowering developers to manage time-related data seamlessly. These approaches offer flexibility and simplicity, catering to various needs while enhancing proficiency in JavaScript’s date manipulation capabilities.
Recent Comments