In JavaScript, generating random numbers is a common requirement for various tasks, ranging from game development to statistical simulations. The ability to create random numbers is crucial, and JavaScript provides us with methods to accomplish this efficiently. In this blog, we’ll learn how to generate random numbers in JavaScript
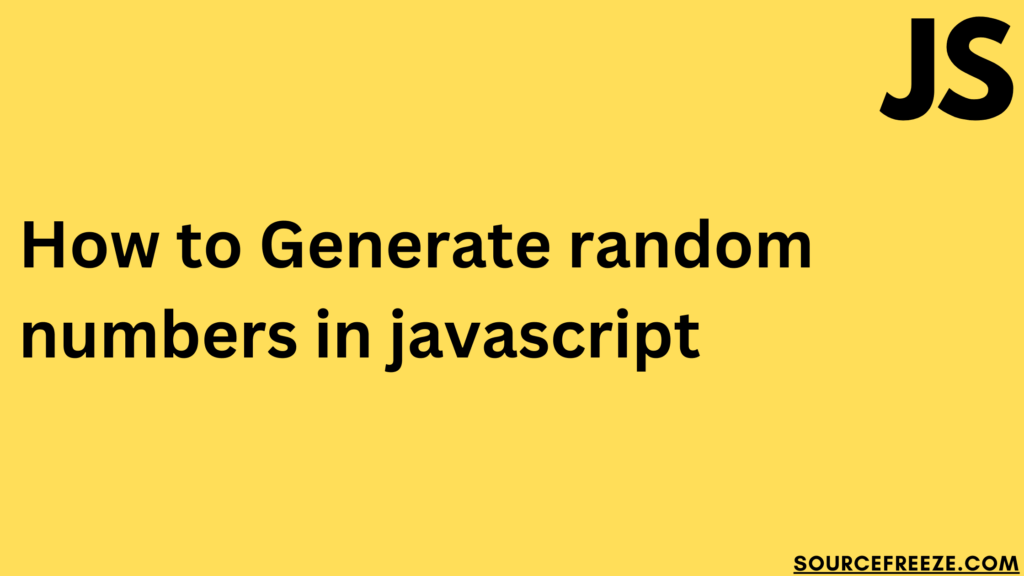
Math.random()
The Math.random() function in JavaScript is foundational for generating pseudo-random floating-point numbers. It returns a floating-point number between 0 (inclusive) and 1 (exclusive), where 0 is inclusive and 1 is exclusive.
Consider this basic example:
const randomNumber = Math.random();
console.log(randomNumber);
Here, Math.random()
provides us with a random decimal number. It’s crucial to understand that this method doesn’t accept any arguments; it solely generates a random decimal between 0 (inclusive) and 1 (exclusive) every time it’s called.
Using Math.random() to Generate Specific Ranges
Now, if we want to create random numbers within a particular range, such as between 10 and 50, we need to manipulate the output of Math.random() using simple arithmetic.
For instance:
function getRandomInRange(min, max) {
return Math.random() * (max - min) + min;
}
const randomInRange = getRandomInRange(10, 50);
console.log(randomInRange);
In this example, getRandomInRange()
accepts a min
and max
value and generates a random number within that range.
Here’s some of the outputs we’ll get in the range on 10 & 50:
23.236899532520656
32.790891513808575
When generating random numbers in JavaScript, one common issue is the output of numbers with numerous decimal places, making the result less manageable or suitable for specific applications. For instance, using Math.random()
to create a range might produce lengthy decimal values.
Consider this code:
function getRandomInRange(min, max) {
return (Math.random() * (max - min) + min).toFixed(2);
}
const randomInRange = getRandomInRange(10, 50);
console.log(randomInRange);
Here, we utilize the toFixed() method to limit the output to two decimal places. This action addresses the problem of excessive decimals, ensuring that the generated random number will always have precisely two decimal points.
Here’s the output:
35.88
44.97
Conclusion
In this blog, we explored JavaScript’s capabilities in generating random numbers. We learned the foundational method, Math.random()
, which allows us to produce unpredictability in our applications.
Alongside we also learnt about the toFixed() method to round up the unnecessary decimal places.
Recent Comments