Sorting a Set in JavaScript involves a few steps but follows a different approach compared to arrays. Sets don’t have a built-in sort() method like arrays, but we can convert them to arrays, sort the arrays, and then convert them back to Sets.
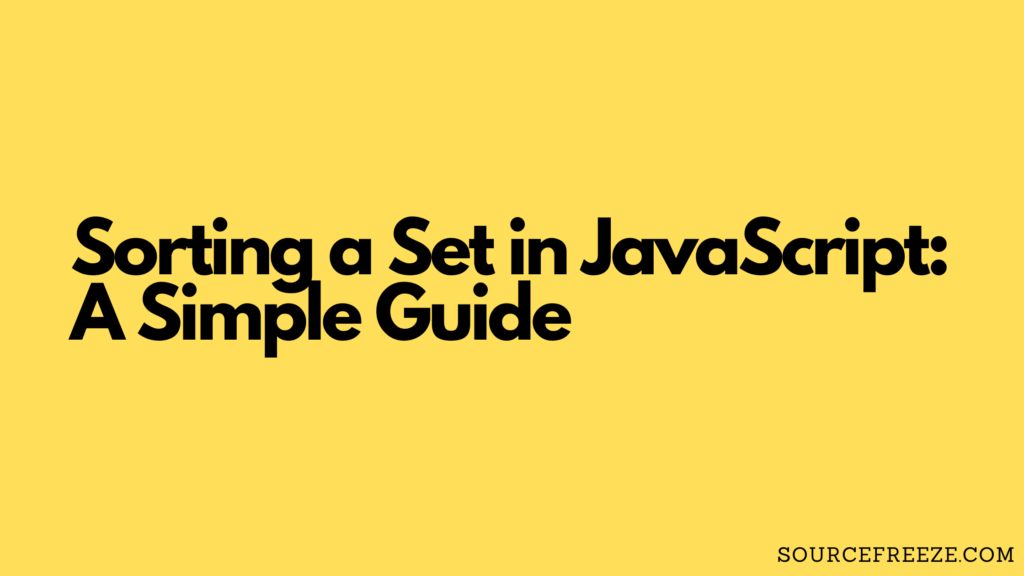
Method 1: Creating an Array from Set Elements
Step 1: Creating and Populating a Set
Create a Set and add elements to it:
let mySet = new Set();
mySet.add(30);
mySet.add(10);
mySet.add(50);
mySet.add(20);
Step 2: Creating an Array from Set Elements
Create an empty array and add elements from the Set using a for loop:
let elementsArray = [];
for (let item of mySet) {
elementsArray.push(item);
}
Step 3: Sorting the Array
Sort the array using sort():
elementsArray.sort();
Step 4: Converting Sorted Array back to Set
Now that the array is sorted, we can convert it back to a Set:
let sortedSet = new Set(elementsArray);
Step 5: Displaying the Sorted Set
Display the sorted Set using a for loop:
console.log("Sorted Set Method 1:");
for (let item of sortedSet) {
console.log(item);
}
The output will be:
Sorted Set Method 1:
10
20
30
50
Method 2: Using Array.from():
Step 1: Creating and Populating a Set
Let’s start by creating a Set and adding elements to it:
let mySet = new Set();
mySet.add(30);
mySet.add(10);
mySet.add(50);
mySet.add(20);
Step 2: Converting Set to Array with Array.from()
Here’s what is different, we will convert the Set to an array using Array.from():
let sortedArray = Array.from(mySet).sort();
Step 3: Converting Sorted Array back to Set
Now we’ll be following the same steps as before i.e. to convert the sorted array back to a Set:
let sortedSet = new Set(sortedArray);
Step 4: Displaying the Sorted Set
Display the sorted Set using a for loop:
console.log("Sorted Set Method 2:");
for (let item of sortedSet) {
console.log(item);
}
The output will still be the same:
Sorted Set Method 2:
10
20
30
50
Conclusion:
Both methods provide a sorted Set. The first approach uses Array.from() to convert the Set to an array, while the second approach involves iterating through the Set to build an array.
Feel free to explore and try out these methods to familiarize yourself with sorting Sets using different JavaScript approaches!
Check out more from Source Freeze here!
Leave a Reply