Sorting arrays of strings in JavaScript is a fundamental operation, and achieving a descending order can be done using various methods. In this blog, we’ll explore two effective approaches to sort an array strings in descending order in JavaScript:
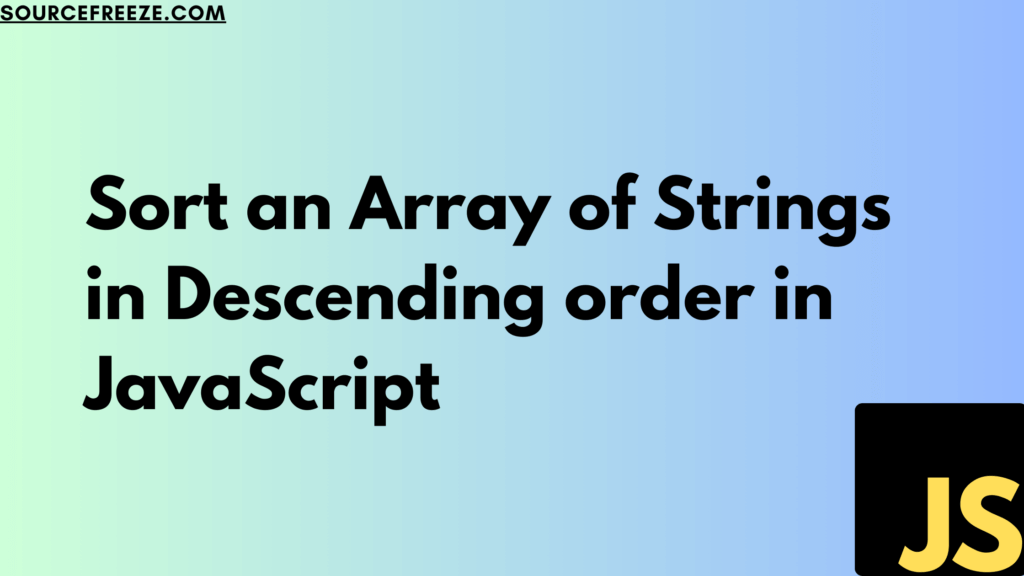
- Sort and Reverse Method: Utilizing the sort() method in conjunction with reverse() to arrange elements in reverse alphabetical order.
- Sort with Comparator Function: Using a custom comparator function within the sort() method to define sorting criteria for descending order.
These methods provide developers with versatile options to efficiently organize string arrays in JavaScript starting with the first one:
Using sort() and reverse()
In JavaScript, sorting an array of strings in descending order involves leveraging the sort()
method in conjunction with reverse()
. This approach enables us to arrange elements in reverse alphabetical order efficiently.
Let’s dive into the step-by-step process:
1. Initialization:
We begin by initializing an array containing strings that need sorting.
let stringArray = ['apple', 'banana', 'orange', 'grape', 'kiwi'];
2. Sorting in Descending Order:
To achieve a descending order, we utilize the sort()
method. This method arranges the elements in ascending order by default.
stringArray.sort();
However, to attain the desired descending order, we need to apply the reverse()
method after sorting:
stringArray.reverse();
3. Result:
Now, stringArray
holds the strings sorted in descending order:
console.log(stringArray);
Output:
['orange', 'kiwi', 'grape', 'banana', 'apple']
This method sorts the elements in ascending order using sort()
and then reverses the sorted array using reverse()
to achieve the desired descending order.
This method is straightforward and effective for sorting arrays of strings in descending order. However, there’s another approach involving a comparator function (sort() with a custom comparison function) that provides more flexibility. Let’s explore that next!
Using sort() and a Comparator Function
1. Initialization:
As before, we start by initializing an array containing strings:
let stringArray = ['apple', 'banana', 'orange', 'grape', 'kiwi'];
2. Sorting with Comparator Function:
To achieve descending order, we’ll use a custom comparator function inside the sort()
method. This function compares elements and rearranges them based on specific criteria.
The comparator function takes two parameters (typically named a
and b
) representing elements being compared. It should return a positive number if a
should come before b
, a negative number if b
should come before a
, or zero if their positions remain unchanged.
stringArray.sort((a, b) => {
if (a > b) {
return -1; // b comes before a (for descending order)
} else if (b > a) {
return 1; // a comes before b
} else {
return 0; // positions unchanged
}
});
3. Result:
After applying the comparator function within sort(), stringArray now contains strings sorted in descending order:
console.log(stringArray);
Output:
['orange', 'kiwi', 'grape', 'banana', 'apple']
This approach utilizes a comparator function within the sort()
method. The function compares elements based on the defined criteria, allowing us to sort the array in descending order by altering the default sorting behavior.
Using a comparator function with sort() grants greater control over sorting criteria, making it versatile when sorting complex data structures or when needing specialized sorting rules.
Conclusion:
In this blog, we explored two powerful techniques for sorting arrays of strings in descending order in JavaScript. We began by employing the sort() method combined with reverse() to achieve a straightforward yet effective sorting mechanism. Additionally, we dove into using a custom comparator function within sort(), offering greater control and flexibility in defining specific sorting criteria.
Leave a Reply