In this tutorial, we will more about how to parse the float with 2 decimals in JavaScript.
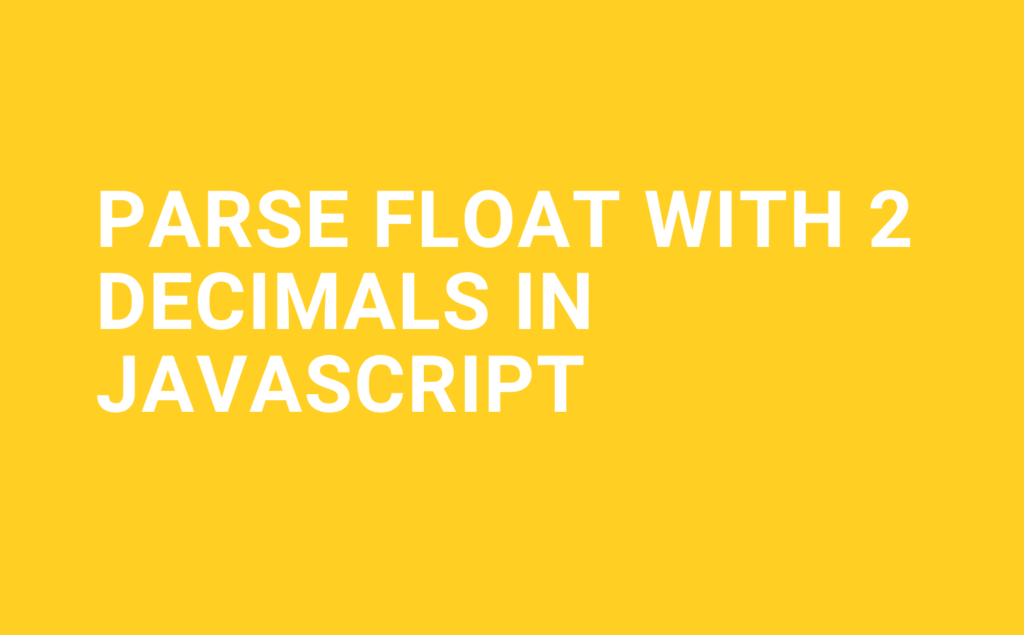
Parse Float with 2 Decimals in JavaScript
To parse float with 2 decimal places:
- First, use the
toFixed(
) method to specify the number of decimal places you want to round the number to. This method rounds the number to the nearest decimal place and AlsotoFixed()
will return the result as a string. - toFixed() method rounds the number up or down, depending on the next decimal place. If you want to always round down, you can use the
Math.floor()
or round up you can useMath.ceil()
- Finally, to convert the parsed number back to a number and not a string, use the
parseFloat()
, this will convert the string to float.
let us see parse float with 2 decimals by example.
First, assign a value that holds the floating point number which we want to parse.
let num = 6.16659265;
Then use toFixed() a
method like below also mentions the number of decimal places we want to round the number for example in the below case I have mentioned 2 decimal places.
let parsedNum = num.toFixed(2);
The toFixed() method rounds the number up or down, depending on the next decimal place. If we want to always round down, we can use the Math.floor()
function. if you want nearest integer please use Math.round() function see the last example.
let parsedNum = Math.floor(num * 100) / 100;
If we want to always round up, we can use the Math.ceil()
function.
let parsedNum = Math.ceil(num * 100) / 100;
Finally, If we need to convert the parsed number to a string, use
the parseFloat()
else you can you the value from the last step directly, like the
below example.
let finalNumber = parseFloat(parsedNum);
Also, we can use the Math.round()
function to method returns the value of a number rounded to the nearest integer.
let parsedNum = Math.round(num * 100) / 100;
Thanks, hope this tutorial is helpful Parse Float with 2 decimals in javascript.
Please let us know if any help is needed. Thanks.
Also please check our other javascript tutorials.
โ How to convert array to space separated strings in javascript
โ How to convert array to comma separated strings in javascript
Leave a Reply