Validating dates will help to ensure users are entering the correct date, In this tutorial will see how to validate date in JavaScript, making your applications reliable and user-friendly.
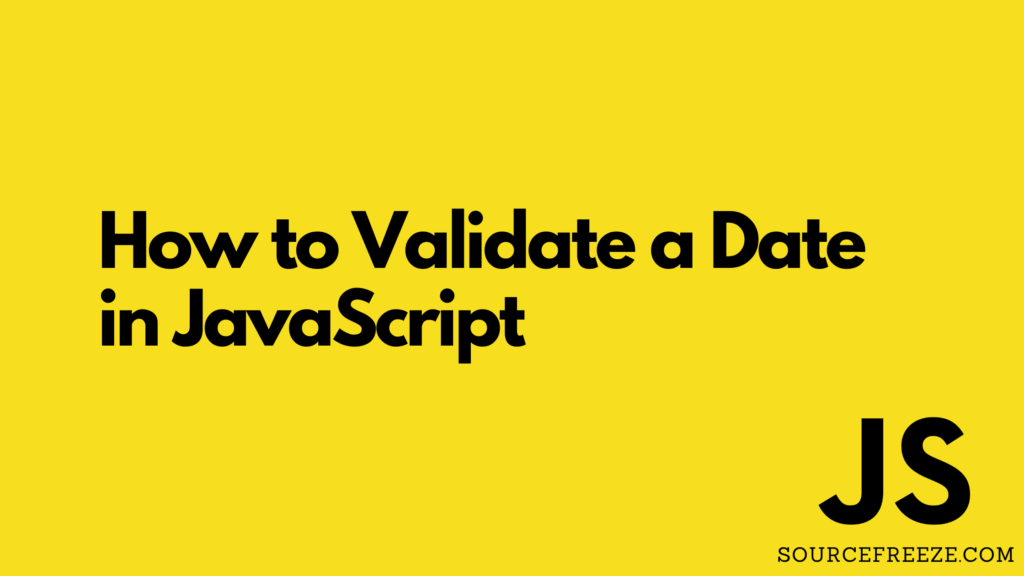
Understanding the Fundamentals
Before we dive into specific techniques, it’s crucial to grasp some basic concepts related to date validation in JavaScript:
- Date Object: The built-in Date object serves as the cornerstone of date manipulation and validation in JavaScript. It provides various methods for parsing date strings, extracting specific information (e.g., year, month, day), and performing calculations.
- Date Formats: Dates can be represented in various formats, each with its own set of conventions. Common formats include YYYY-MM-DD (ISO 8601), MM/DD/YYYY (US format), and DD/MM/YYYY (European format).
- Valid Date Range: Dates must fall within a specific range, typically between January 1, 1970 (Unix epoch) and the maximum representable date. Understanding these limitations is crucial for preventing invalid entries and potential errors.
Moment.js for Date Validation in JavaScript
Dates are an integral part of countless applications, from booking flights to managing finances. But how do we ensure that the dates users enter are accurate and valid? Here, we’ll explore the power of Moment.js, a popular JavaScript library that simplifies and accelerates date validation and manipulation.
The moment() method within Moment.js is pivotal for validating dates. This function requires three parameters:
- Date String: This is the date we want to validate, like “2023-12-06”.
- Date Format: We need to tell Moment.js how the date is formatted. For “2023-12-06”, the format would be “YYYY-MM-DD”.
- Strict Parsing: If we set this to true, Moment.js will be extra picky. The date string must exactly match the format.
Now, we’ll use the isValid() function to see if the date is valid. It’s like asking Moment.js, “Hey, is this date legit?”
Example 1: Validate Date in JavaScript
const moment = require('moment');
let dateString = "2023-12-06";
let dateFormat = "YYYY-MM-DD";
let isDateValid = moment(dateString, dateFormat).isValid();
console.log(isDateValid);
Output:
true
Example 2: Validate Date in JavaScript
const moment = require('moment');
let dateString = "2024-13-32";
let dateFormat = "YYYY-MM-DD";
let isDateValid = moment(dateString, dateFormat).isValid();
console.log(isDateValid); // Output: false
Output:
false
Using the built-in Date Object:
When working with the Date object, we’ll walk through a simple process to check if a date is valid or not. First, let’s create a date object by specifying the date we need to validate.
Our Three-Step Validation Process:
Date Extraction: We’ll retrieve the user-provided date from a form field, API response, or any other source.
Creating a Date Object: We’ll leverage the Date constructor to transform the extracted date string into a dedicated Date object for further processing.
Validation Magic: Here’s where the magic happens! We’ll utilize the getTime() method to examine the internal representation of the Date object. If it returns a valid number, the date is deemed valid. Otherwise, it’s flagged as invalid.
Let’s put this into action with some code examples:
Example 1: Validating a Date String (YYYY/MM/DD):
function isDateValidYYYYMMDD(dateString) {
// Create a `Date` object from the string
const dateObject = new Date(dateString);
// Check if the object is valid
return !isNaN(dateObject.getTime());
}
const userDate = "2023-12-06";
const isValid = isDateValidYYYYMMDD(userDate);
console.log(isValid); // Output: true
Explanation:
- We create a Date object using the user-provided date string.
- The getTime() method returns a valid number if the date is valid, and NaN otherwise.
- We use the ! operator to negate the result because we want true for valid dates.
Example 2: Validating a Date String (MM/DD/YYYY):
function isDateValidMMDDYYYY(dateString) {
// Split the date string into an array of month, day, and year
const dateParts = dateString.split("/");
// Convert the month to a zero-based index
const month = parseInt(dateParts[0]) - 1;
// Convert all values to integers
const year = parseInt(dateParts[2]);
const day = parseInt(dateParts[1]);
// Create a `Date` object with the converted values
const dateObject = new Date(year, month, day);
// Check if the object is valid
return !isNaN(dateObject.getTime());
}
const userDate = "12/06/2023";
const isValid = isDateValidMMDDYYYY(userDate);
console.log(isValid); // Output: true
Explanation:
- We split the date string into separate components using the / character.
- We convert the month to a zero-based index because JavaScript months start at 0.
- We convert all values to integers to ensure proper parsing.
- We create a Date object with the converted year, month, and day.
- We use the same getTime() method and negation as in the previous example.
Conclusion:
While the Date object offers a strong foundation, the path to mastering validating date in javascript extends beyond it. Several other techniques await exploration, each with unique strengths and applications. Consider venturing into the realms of:
- Regular Expressions: These powerful patterns allow for precise matching of specific date formats, ensuring adherence to custom or international standards.
- Comparison Operators: Empower yourself to define desired date ranges and compare user-provided dates against them, enabling validation within specific boundaries and time periods.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply