Scrolling to specific elements within a React component is a common requirement in web development. Whether it’s navigating to a particular section of a webpage or focusing on an element after an action, understanding the methods to achieve this within a React context is essential. In this guide, we’ll learn how to Scroll to an Element in a React Component?
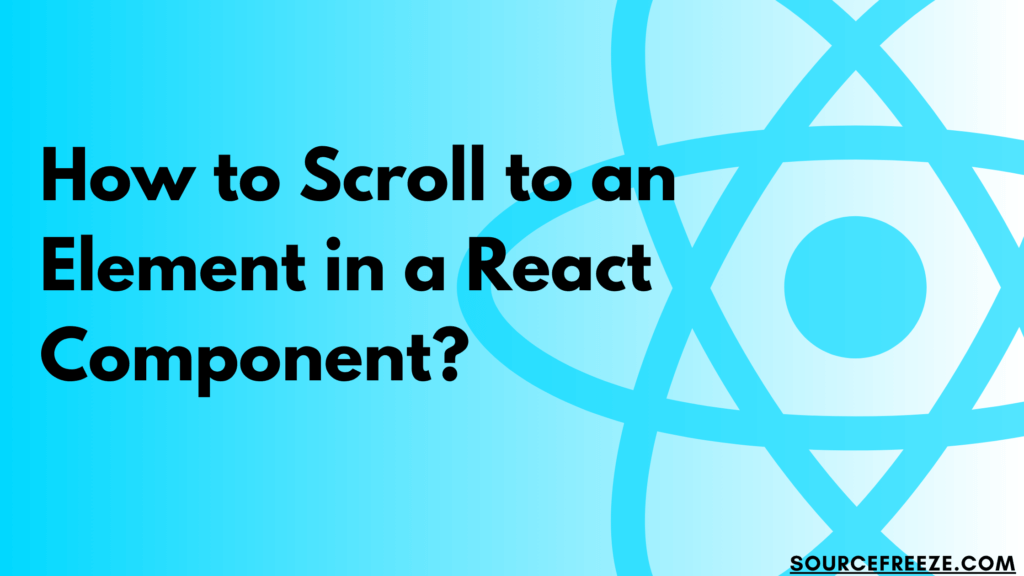
Using scrollIntoView()
The scrollIntoView() method is a native browser API that smoothly scrolls the specified element into the viewport. In React, we can access this method using the ref attribute to reference the DOM element.
Let’s assume we have a React component with a target element we want to scroll to:
import React, { useRef } from 'react';
const ScrollComponent = () => {
const elementRef = useRef(null);
const scrollToElement = () => {
if (elementRef.current) {
elementRef.current.scrollIntoView({ behavior: 'smooth' });
}
};
return (
<div>
<button onClick={scrollToElement}>Scroll to Element</button>
<div ref={elementRef}>Target Element to Scroll To</div>
{/* Other content */}
</div>
);
};
export default ScrollComponent;
We use the useRef() hook to create a reference to the target element (elementRef).Upon clicking the button, the scrollToElement() function is triggered.Inside scrollToElement(), we check if the reference to the element exists (elementRef.current). If it does, we invoke scrollIntoView() on that element with the option { behavior: ‘smooth’ }, ensuring a smooth scrolling effect.
Using scroll(x, y)
The scroll()
method is a straightforward way to scroll an element to a particular position, specified by coordinates (x, y)
. In a React context, we can employ this method by obtaining a reference to the parent container or the window
object and then utilizing scroll()
to navigate to a specific position.
Consider a scenario where we want to scroll a container to a particular position:
import React, { useRef } from 'react';
const ScrollComponent = () => {
const containerRef = useRef(null);
const scrollToPosition = () => {
if (containerRef.current) {
const { current: container } = containerRef;
container.scrollTo({ top: 200, behavior: 'smooth' });
}
};
return (
<div ref={containerRef} style={{ height: '300px', overflowY: 'scroll' }}>
<button onClick={scrollToPosition}>Scroll to Position</button>
<div style={{ height: '600px' }}>Scrolling Container</div>
{/* Other content */}
</div>
);
};
export default ScrollComponent;
We create a reference to the container element (containerRef) using the useRef() hook.Upon clicking the button, the scrollToPosition() function is triggered.Inside scrollToPosition(), we retrieve the DOM element using the reference (containerRef.current). Then, we use the scrollTo() method with the desired position (top: 200) and a smooth scrolling behavior.
Here’s how it looks like:
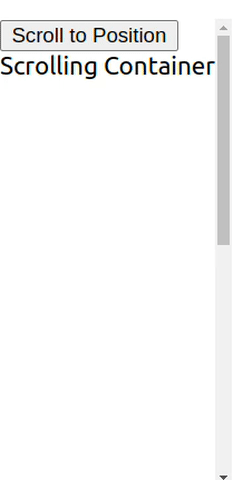
Conclusion
In this guide, we explored various techniques to smoothly scroll to elements within React components, enhancing user interaction and navigation on web pages. We learnt two prominent methods:
scrollIntoView()
Method:- This method smoothly scrolls the specified element into the viewport.
- Utilizes the ref attribute to reference the DOM element and triggers scrollIntoView() for smooth scrolling behavior.
scroll(x, y)
Method:- Allows scrolling to specific coordinates within an element or the window.
- Utilizes the scroll() method with precise positioning coordinates (e.g., top, left) for targeted scrolling.
Leave a Reply