In nextjs we can easily get the query params with the use of useRouter()
that we can import from 'next/router'
then assign userRouter()
to the router variable after that using router.query we can get the exact query string values. if you are passing multiple queries also we can get the values from router.query.
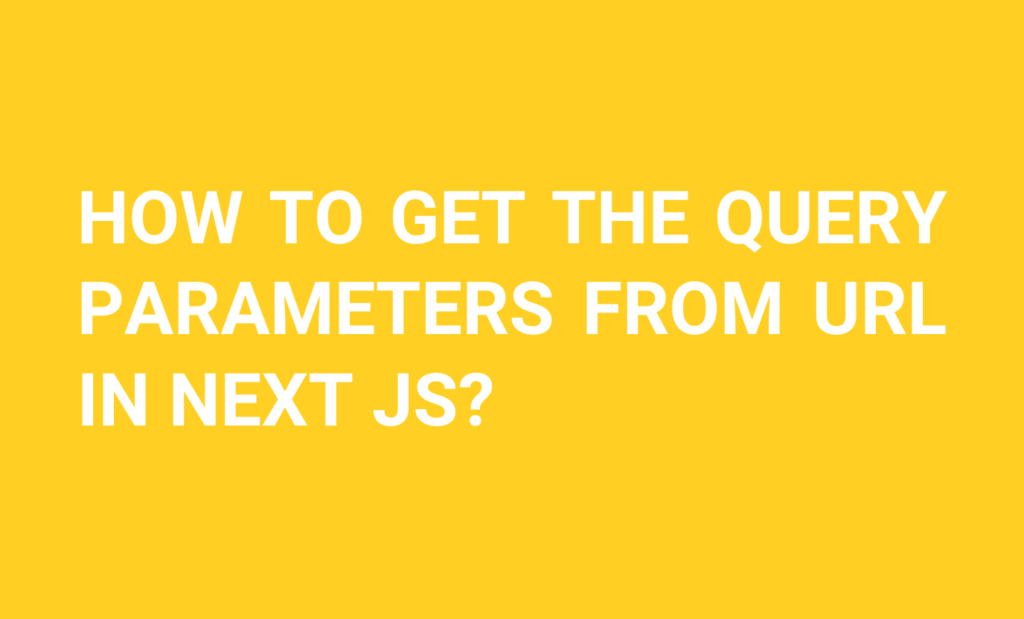
Here in the example, we can see the detailed way, how to get the query params from the URL in NextJS it is applicable for single query params or multiple query params.
First, we can see how to pass the query params to the link to the next calling page, refer to the below code.
import Link from "next/link";
<Link href={{ pathname: '/search', query: { keyword: 'source freeze' } }}>
<a>Search</a>
</Link>
Then next we need to get the query params from the URL, here we are three options to get the query params, please refer to the below options.
- In Component
- Using getServerSideProps Function
- In API Routes
First, we can see the option to get query params in the component
Access Query Params in the Component
To get the query params in the component first we need to import the useRouter from the ‘next/router’, then create a variable router and assign the useRouter to that variable, then using router.query we can able to get the exact params, used anywhere on the page.
import { useRouter } from 'next/router';
function Search(props) {
const router = useRouter();
// Get the query parameter from the URL
const { keyword } = router.query;
return (
<div>
The Search Keyword {keyword}.
</div>
);
}
export default Search;
Also if you have passed multiple keywords we can easily access them like the one below.
import { useRouter } from 'next/router';
function Search(props) {
const router = useRouter();
// Get the query parameter from the URL
const { keyword1, keyword2 } = router.query;
return (
<div>
The Search Keyword {keyword1} {keyword2}.
</div>
);
}
export default Search;
Next, we can see the option how to get the query params using getServerSideProps
function
How to Get Query Parameters In a getServerSideProps
Using getServerSideProps
function in Next.js we can generate server-side rendered pages, also it receives a context object as an argument, which contains a query property that holds the query parameters for that page.
We can access the query parameters by accessing the context value below.
export async function getServerSideProps({ context }) {
// context value contains the query params
const param = context.paramName;
}
If we want to access multiple query parameters, we can access them like the one below.
export async function getServerSideProps({ context }) {
const queryparam1 = context.param1;
const queryparam2 = context.param2;
const queryparam3 = context.param3;
}
Accessing Query Params In API Routes
We can also access query params by API route, we will get the req object property by destructuring the request object we can access the query params.
Here’s an example to access the query params in the API routes
import { NextApiRequest, NextApiResponse } from 'next';
export default (req: NextApiRequest, res: NextApiResponse) => {
const { query } = req;
const queryParams = query.paramName;
res.status(200).json({ queryParams });
};
Conclusion:
So there are three methods we can access the query params in NextJS
- In the component, we can
useRouter()
hook to access query parameters. - In a server-side rendered page using
getServerSideProps
, we can access query parameters by destructuring the query property from the context. - In an API route, we can access query parameters by destructuring the query property from the req object.
Leave a Reply