In React, obtaining the current year may seem like a simple task, yet it plays a crucial role in various applications, especially when displaying dynamic content or creating time-sensitive features. We’ll explore two practical methods to achieve this: utilizing JavaScript’s Date object and leveraging React’s state management to update the year dynamically.
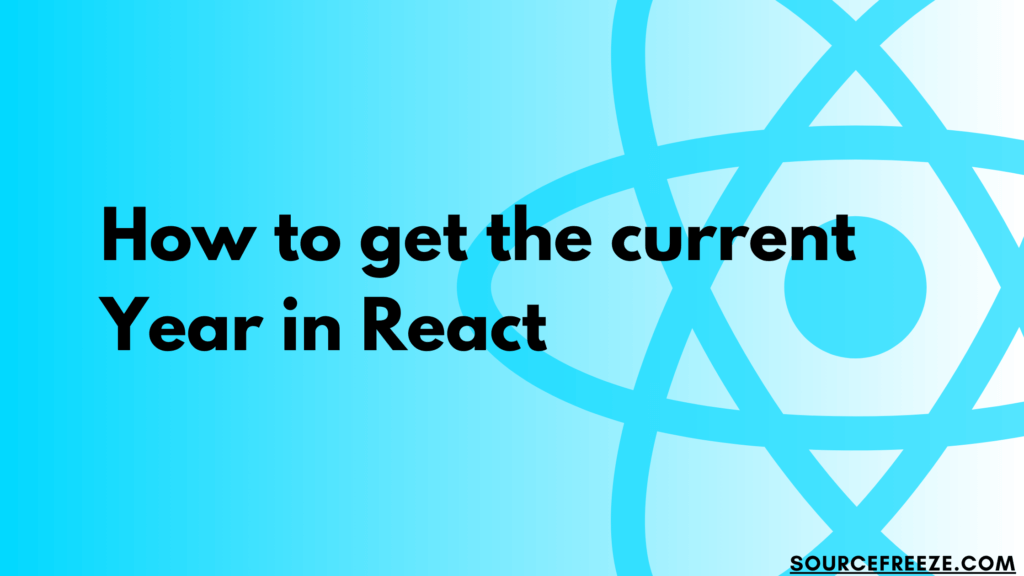
Using JavaScript’s Date Object
We’ll begin by defining a method, getCurrentYear
, which utilizes the Date
object to fetch the current year.
const getCurrentYear = () => {
const currentDate = new Date();
const currentYear = currentDate.getFullYear();
return currentYear;
};
Obtaining the Current Month
Similarly, we’ll create a function, getCurrentMonth
, to retrieve the current month. Note that JavaScript’s getMonth()
method returns the month starting from 0, hence the addition of 1 to display the correct month number.
const getCurrentMonth = () => {
const currentDate = new Date();
const currentMonth = currentDate.getMonth() + 1;
return currentMonth;
};
Integrating Methods in a React Component
Now, let’s integrate these functions into a React component. This component will display the current year and month, updating them dynamically upon button clicks.
import React, { useState } from 'react';
const App = () => {
const getCurrentYear = () => {
const currentDate = new Date();
const currentYear = currentDate.getFullYear();
return currentYear;
};
const getCurrentMonth = () => {
const currentDate = new Date();
const currentMonth = currentDate.getMonth() + 1;
return currentMonth;
};
const [year, setYear] = useState(0);
const [month, setMonth] = useState(0);
const updateYear = () => {
const updatedYear = getCurrentYear();
setYear(updatedYear);
};
const updateMonth = () => {
const updatedMonth = getCurrentMonth();
setMonth(updatedMonth);
};
return (
<div>
<p>Current Year: {year}</p>
<button onClick={updateYear}>Update Year</button>
<p>Current Month: {month}</p>
<button onClick={updateMonth}>Update Month</button>
</div>
);
};
export default App;
Result:
Here’s how the page looks like before we click the buttons:
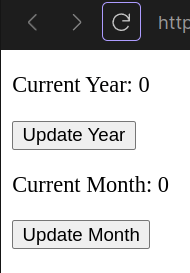
After clicking the buttons and getting updated values(It’s December 2023 for me while writing):
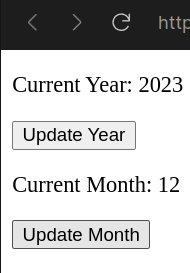
Code Explanation:
Let’s break down the code and explanation into concise points to delve deeper into howthe App component operates:
State Initialization:
- const [year, setYear] = useState(0);
- Initializes the
year
state usinguseState
hook with an initial value of0
. setYear
is the function used to update theyear
state.
- Initializes the
- const [month, setMonth] = useState(0);
- Initializes the
month
state usinguseState
hook with an initial value of0
. setMonth
is the function used to update themonth
state.
- Initializes the
Getting the year using const getCurrentYear = () => { … }
- Defines a function
getCurrentYear
that uses theDate
object to retrieve the current year. getCurrentYear()
returns the current year value.
Getting the month const getCurrentMonth = () => { … }
- Defines a function
getCurrentMonth
that uses theDate
object to retrieve the current month. - The month retrieved is incremented by 1 to match standard month numbers (January as 1, February as 2, and so on).
Updating year using const updateYear = () => { … }
- Defines a function
updateYear
to update theyear
state. - Calls
getCurrentYear()
to fetch the current year and usessetYear
to update the state.
Updating month const updateMonth = () => { … }
- Defines a function
updateMonth
to update themonth
state. - Calls
getCurrentMonth()
to fetch the current month and usessetMonth
to update the state.
Return Statement:
- JSX Structure:
- Renders a <div> containing:
<p>
displaying the current year:Current Year: {year}
<button>
triggeringupdateYear
function on click:Update Year
<p>
displaying the current month:Current Month: {month}
<button>
triggeringupdateMonth
function on click:Update Month
- Renders a <div> containing:
Event Handling:
- onClick Events:
- When the
Update Year
button is clicked, it triggers theupdateYear
function, updating theyear
state with the current year. - When the
Update Month
button is clicked, it triggers theupdateMonth
function, updating themonth
state with the current month.
- When the
Conclusion
In this blog, we explored practical methods using JavaScript’s Date object and React’s state management to dynamically retrieve and display the current year and month in a React application. Leveraging these methods provides a seamless way to showcase real-time date information and enables easy updates through user interactions.
Leave a Reply