JavaScript helps us figure out what type of file from the URL. Knowing how to detect file types programmatically—be it images, documents, or multimedia—is fundamental in various web applications. In this blog, we’ll see how to get a file type from URL in JavaScript.
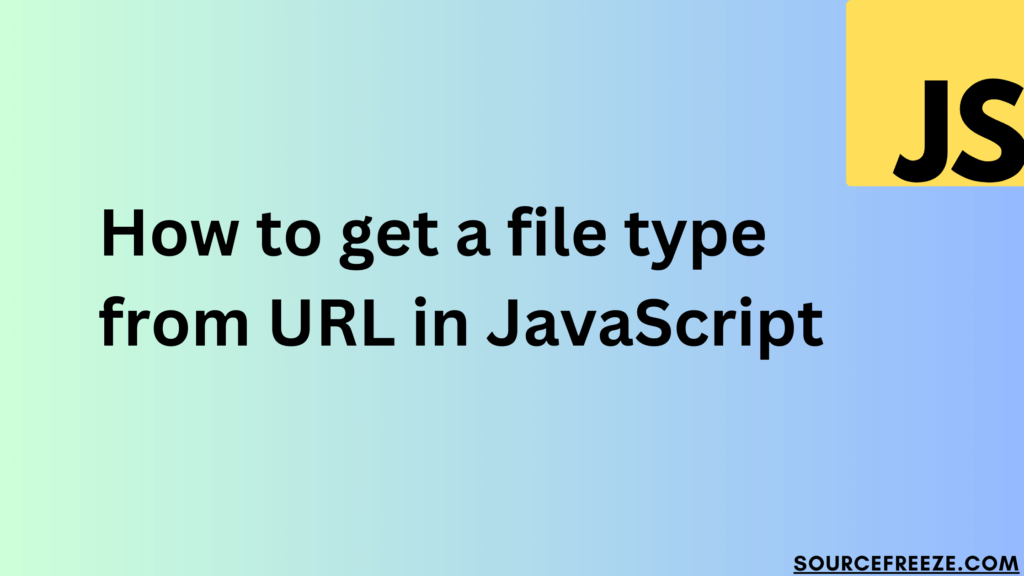
Using JavaScript’s split() Function
JavaScript’s split() function proves handy in parsing URLs. We can use this function to isolate the file extension—the part after the last period in a URL—to determine the file type.
Consider this sample code snippet:
function getFileTypeFromURL(url) {
const segments = url.split('.');
const extension = segments[segments.length - 1];
return extension.toLowerCase(); // Convert to lowercase for consistency
}
Example usage:
const url = 'https://example.com/images/image.jpg';
const fileType = getFileTypeFromURL(url);
console.log('File type:', fileType);
This function getFileTypeFromURL() splits the URL based on the periods (.), extracting the file extension from the last segment. This simplistic approach, while effective for most cases, might not cover URLs without extensions or with unconventional naming conventions.
Output:
File type: jpg
Utilizing Regular Expressions
Regular expressions offer a robust way to extract file extensions from URLs, accommodating various naming conventions and handling more complex scenarios.
Consider this updated code:
function getFileTypeWithRegex(url) {
const regex = /(?:\.([^.]+))?$/; // Regular expression to capture file extension
const extension = regex.exec(url)[1]; // Extract extension from URL
return extension ? extension.toLowerCase() : 'No extension found';
}
Example usage:
const url = 'https://example.com/files/document.pdf';
const fileType = getFileTypeWithRegex(url);
console.log('File type:', fileType);
This function getFileTypeFromURL()
splits the URL based on the periods (.
), extracting the file extension from the last segment. This simplistic approach, while effective for most cases, might not cover URLs without extensions or with unconventional naming conventions.
Output:
File type: pdf
Implementation of split() Technique:
The split() method dissects a string based on a specified separator. In our case, we use the period (.) as the separator to extract the file extension from a URL.
Consider this detailed code example:
function getFileTypeFromURL(url) {
const segments = url.split('/'); // Split the URL by '/'
const lastSegment = segments[segments.length - 1]; // Extract the last segment (file name)
const fileParts = lastSegment.split('.'); // Split the file name by '.'
if (fileParts.length === 1) {
return 'No file extension found';
} else {
const extension = fileParts[fileParts.length - 1]; // Retrieve the extension
return extension.toLowerCase(); // Return the extension in lowercase for consistency
}
}
// Example usage:
const imageURL = 'https://example.com/images/image.jpg';
const documentURL = 'https://example.com/files/document.pdf';
const imageFileType = getFileTypeFromURL(imageURL);
const documentFileType = getFileTypeFromURL(documentURL);
console.log('Image file type:', imageFileType);
console.log('Document file type:', documentFileType);
This getFileTypeFromURL() function splits the URL into segments, retrieves the last segment (presumed to be the file name), and further splits the file name to extract the file extension. It then returns the extracted extension in lowercase, providing insight into the file type.
Here’s the output:
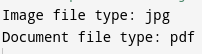
Conclusion:
JavaScript makes it easy to know what type of file we’re dealing with, just by checking its web address. With these methods, handling different files becomes simpler for websites and apps.
Leave a Reply