JavaScript, the language of the web, helping us to work with dates effortlessly. When handling dates, sometimes we only need the date without the time component. This could be for displaying events, scheduling, or organizing tasks. Fortunately, JavaScript offers various methods to extract the date without the time, making it simpler for developers to manipulate and display dates accurately. In this guide, we’ll explore several methods to get a Date without the Time in JavaScript.
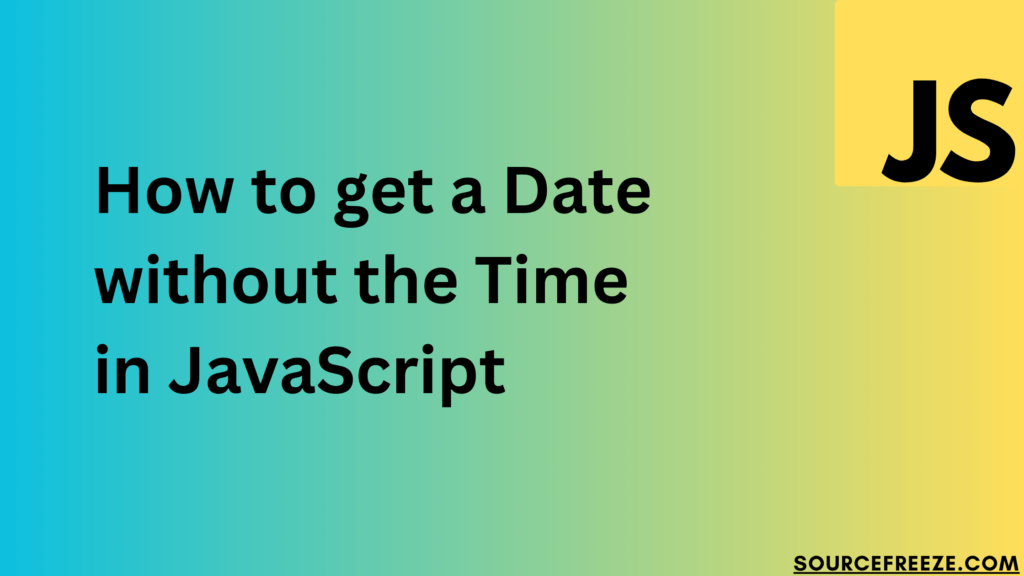
Using the Date Object Methods
The Date
object in JavaScript comes with several methods that allow us to manipulate dates. One of these methods is toISOString()
, which returns the date in the format of a string representing the date and time. To extract only the date part from this string, we can utilize the split()
method along with string manipulation.
// Get the current date
const currentDate = new Date();
// Convert the date to ISO string format
const dateISOString = currentDate.toISOString();
// Extract only the date part
const dateWithoutTime = dateISOString.split('T')[0];
console.log('Date without time:', dateWithoutTime);
This code creates a Date
object named currentDate
, representing the current date and time when this code executes. This object holds both the date and time components.
The toISOString() method converts the currentDate object to a string representation in the ISO 8601 format (YYYY-MM-DDTHH:mm:ss.sssZ). This format includes the date, time, and timezone information, separated by the ‘T’ character.
Here, split(‘T’)[0] is used to separate the date and time components in the ISO string. It splits the string at the ‘T’ character, resulting in an array with two parts. The [0] index retrieves the first part, which contains only the date information. The final variable dateWithoutTime stores the extracted date without the time component.
Output:
Date without time: 2023-12-19
Using setHours() Method
The setHours()
method allows us to manipulate the hour component of a date object. By setting the hours, minutes, seconds, and milliseconds to zero, we effectively eliminate the time portion, retaining only the date.
Let’s explore this method through a code example:
// Function to extract date without time using setHours() with padding
function getDateWithoutTime(date) {
// Create a new date object to avoid modifying the original date
const dateWithoutTime = new Date(date);
// Set hours, minutes, seconds, and milliseconds to zero
dateWithoutTime.setHours(0, 0, 0, 0);
// Format date components with padding
const year = dateWithoutTime.getFullYear();
const month = String(dateWithoutTime.getMonth() + 1).padStart(2, '0');
const day = String(dateWithoutTime.getDate()).padStart(2, '0');
return `${year}-${month}-${day}`;
}
// Get the current date
const currentDate = new Date();
// Obtain the date without time and format it with padding
const dateNoTime = getDateWithoutTime(currentDate);
console.log('Date without time:', dateNoTime);
In this code snippet, the getDateWithoutTime() function serves the purpose of isolating the date component without any time-related information. To achieve this, the setHours(0, 0, 0, 0) method is used, resetting the hours, minutes, seconds, and milliseconds to zero within a new Date object created from the provided date.
Furthermore, to ensure uniformity and a standardized format when displaying the date, the function proceeds to format the year, month, and day components. The getFullYear(), getMonth(), and getDate() methods retrieve these components, while padStart() ensures that single-digit months and days are padded with a leading zero, resulting in a consistent format like ‘YYYY-MM-DD’.
Output:
Date without time: 2023-12-19
Using the setSeconds() Method:
The getSeconds() method within the JavaScript Date object retrieves the seconds component from a given date. Though getSeconds() doesn’t directly help in extracting a date without the time, we can still employ it alongside other methods to ensure consistent formatting when displaying a date without the time component.
Here’s an example demonstrating the usage of getSeconds()
in conjunction with other methods to format the date without time:
// Function to extract date without time using getSeconds() with padding
function getDateWithoutTime(date) {
// Create a new date object to avoid modifying the original date
const dateWithoutTime = new Date(date);
// Set seconds and milliseconds to zero
dateWithoutTime.setSeconds(0, 0);
// Format date components with padding
const year = dateWithoutTime.getFullYear();
const month = String(dateWithoutTime.getMonth() + 1).padStart(2, '0');
const day = String(dateWithoutTime.getDate()).padStart(2, '0');
return `${year}-${month}-${day}`;
}
// Get the current date
const currentDate = new Date();
// Obtain the date without time and format it with padding
const dateNoTime = getDateWithoutTime(currentDate);
console.log('Date without time:', dateNoTime);
This code performs similar operations as before, creating a function getDateWithoutTime() to extract a date without the time component. Despite using setSeconds() to reset the seconds and milliseconds to zero, it’s important to note that the seconds value itself isn’t utilized in the final output.
The getSeconds() method is used indirectly, ensuring that the time is nullified by setting the seconds and milliseconds to zero. The subsequent formatting of year, month, and day components with padding, similar to the previous example, guarantees a consistent ‘YYYY-MM-DD’ format when presenting the date without the time component.
Output will still be the same:
Date without time: 2023-12-19
Conclusion
In this comprehensive guide, we’ve explored various methods in JavaScript to extract a date without its accompanying time component. Through practical code examples and explanations, we’ve gained insights into different approaches:
- Leveraging
toISOString()
and String Manipulation: By converting the date to an ISO string format and extracting only the date portion through string manipulation methods likesplit()
, we can isolate the date component. - Using
setHours()
andsetSeconds()
: These methods within theDate
object allow us to reset specific time components to zero, effectively removing the time element and leaving us solely with the date.
Leave a Reply