Maps are powerful data structures in JavaScript, storing key value pairs. But sometimes, we need to extract specific information from this wealth of data. That’s where filtering comes in, allowing us to focus on the key-value pairs that meet our criteria. in this tutorial, we will see how to filter a map in javascript
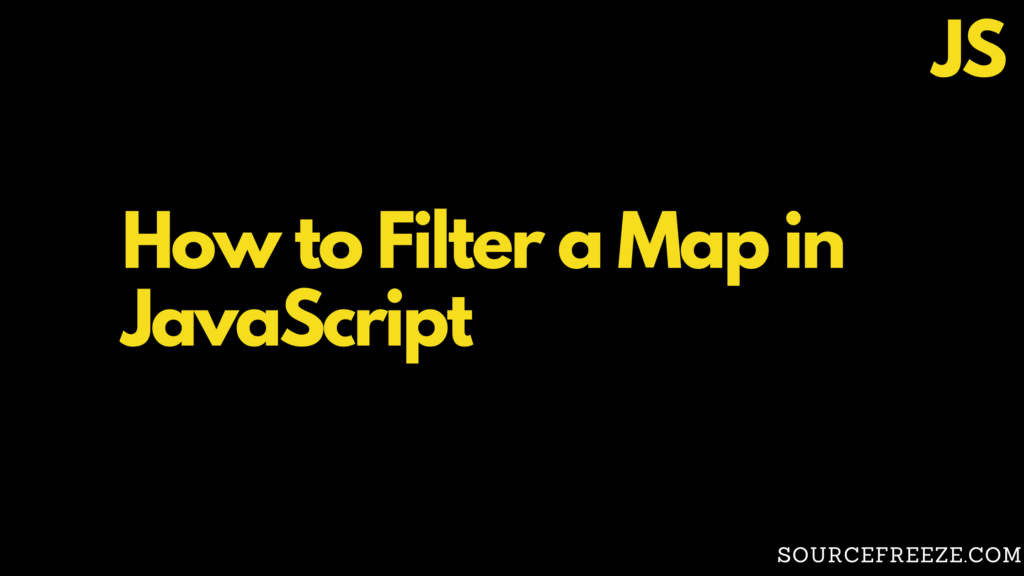
Here, we’ll explore two effective methods for filter a map in JavaScript:
Method 1: Utilizing Array.from() and filter():
Step 1: Create a Sample Map
Let’s start by creating a Map with some key-value pairs:
let myMap = new Map();
myMap.set('John', 35);
myMap.set('Alice', 29);
myMap.set('Bob', 42);
Step 2: Convert Map Entries to Array and Apply Filtering Logic
We’ll use Array.from() to convert the Map’s entries into an array and then apply the filter() function to select elements that meet the filtering condition:
let filteredEntries = Array.from(myMap).filter(([key, value]) => {
// Define your filtering condition here
return value < 30; // Example: Filter elements with value less than 30
});
Step 3: Reconstruct a Filtered Map
Reconstruct a Map from the filtered array of entries using the Map constructor:
let filteredMap = new Map(filteredEntries);
Step 4: Display the Filtered Map
Loop through the filteredMap and display the key-value pairs:
for (let [key, value] of filteredMap) {
console.log(`${key}: ${value}`);
}
Output:
Alice: 29
Method 2: Using Map.prototype.forEach()
Step 1:
We’ll use the same map as before:
let myMap = new Map();
myMap.set('John', 35);
myMap.set('Alice', 29);
myMap.set('Bob', 42);
Step 2: Initialize an Empty Map for Filtered Entries
Create an empty Map to hold the filtered entries:
let filteredMap = new Map();
Step 3: Use forEach to Filter the Map
Utilize the forEach() method on the original Map to filter entries based on a specified condition:
myMap.forEach((value, key) => {
// Define your filtering condition here
if (value < 30) {
filteredMap.set(key, value);
}
});
Step 4: Display the Filtered Map
Loop through the filteredMap and display the key-value pairs:
filteredMap.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
Output:
We get the same output as before:
Alice: 29
Ultimately, the best method depends on your specific needs, coding preferences, and the complexity of your filtering criteria.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply