In the world of JavaScript, tracking user actions is crucial. Understanding when a user stops typing can enhance user experience, enabling applications to respond effectively. When we talk about detecting typing pauses, we’re essentially interested in capturing the moment when the user finishes entering text. There are several methods to detect when the user stops typing in JavaScript:
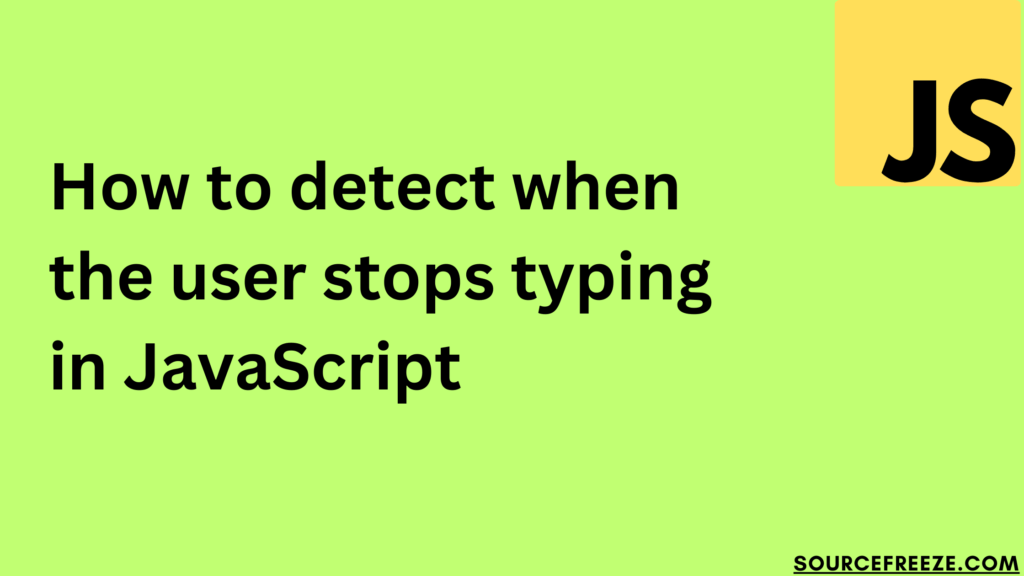
The first method to detect when a user stops typing in JavaScript involves using the input event and a timer. This technique monitors the input field and triggers an action when the user pauses typing.
Using input Event and Timer
This technique involves leveraging the input
event, which is fired whenever the value of an input field changes. When a user types in an input field, this event continuously triggers, indicating ongoing activity. However, to pinpoint when the user stops typing, we incorporate a timer mechanism.
const inputField = document.getElementById('yourInputField');
let typingTimer;
inputField.addEventListener('input', function() {
// Clear previous timeout to avoid premature triggers
clearTimeout(typingTimer);
// Set a new timeout of 800 milliseconds (adjustable)
typingTimer = setTimeout(function() {
// Perform action after typing pause
actionAfterTypingPause();
}, 800); // Adjust time duration as needed
});
Here’s how it works: Upon detecting the input
event, we initialize a timer. If the user continues typing, this timer keeps getting reset, preventing premature triggers. However, when the user pauses typing, the timer finally elapses after a specified duration (here, set at 800 milliseconds). It’s at this point that we consider the typing paused and execute the intended action.
Using Event Debouncing
Debouncing involves optimizing event handling by delaying the execution of a function until after a certain time period has passed since the last fired event. This method helps in handling rapid, successive events and detecting pauses effectively.
Debounce Function: We’ll create a debounce function that delays the execution of a function until a specified time has elapsed since the last event. Here’s a simple implementation:
function debounce(func, delay) {
let timeoutId;
return function() {
clearTimeout(timeoutId);
timeoutId = setTimeout(func, delay);
};
}
Implementing the Debounce Function: Now, let’s apply this debounce function to our input field event listener:
const inputField = document.getElementById('yourInputField');
inputField.addEventListener('input', debounce(function() {
// Perform action after typing pause
actionAfterTypingPause();
}, 800)); // Set the delay duration as needed (800 milliseconds in this case)
Action on Typing Pause: Replace actionAfterTypingPause() with the function or action you wish to execute when the typing pause occurs. This could include form validation, sending HTTP requests, or updating suggestions.
Here’s how it works: The debounce function we create acts as a wrapper around the actual function we want to execute. When the input event fires (i.e., when the user types), this function postpones the execution of our target action until a specified time (here, set at 800 milliseconds) has passed since the last input event.
This delay gives users a chance to finish typing before any action takes place. If another input event occurs within this time frame, the previous timer gets cleared, effectively resetting the countdown. Only when the user pauses typing for the entire specified duration does the action finally execute.
Utilizing the keyup Event Listener
Attach the keyup Event Listener: We’ll add an keyup
event listener to the input field, capturing each key release action.
const inputField = document.getElementById('yourInputField');
inputField.addEventListener('keyup', function() {
// Perform action after typing pause
actionAfterTypingPause();
});
Perform Action on Typing Pause: Similar to previous methods, replace actionAfterTypingPause()
with the function or action you intend to execute when the typing pause occurs. This action could involve validation checks, auto-completion suggestions, or any relevant updates based on user input.
Observing Key Releases: The keyup
event fires each time a key is released within the input field. By listening to these events, we can effectively track the user’s typing activity and identify pauses between keystrokes.
Here’s an HTML example incorporating an input field and the JavaScript code utilizing the keyup
event listener to detect typing pauses:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Typing Pause Detection</title>
</head>
<body>
<input type="text" id="textInput" placeholder="Start typing...">
<script>
// JavaScript code
const inputField = document.getElementById('textInput');
inputField.addEventListener('keyup', function() {
// Replace this with your intended action after typing pause
// For demonstration purposes, log a message
console.log('Detected typing pause!');
// You can call a function or execute specific actions here
});
</script>
</body>
</html>
This HTML file includes a simple input field (<input type="text">
) with an ID of “textInput” where users can input text. The JavaScript code snippet within the <script>
tags listens for keyup
events on this input field.
Upon detecting a keyup
event (when a key is released after being pressed), the code executes the specified action. In this example, a message is logged to the console to indicate a detected typing pause. You can replace the console.log
statement with any desired action or function to be performed when the user pauses typing. As you type in the input field and release keys, the console should log the message
Detected typing pause!
Conclusion:
In this blog, we explored various methods to detect typing pauses in JavaScript. We delved into three distinct techniques:
- Using the
input
Event and Timer: This method involves setting up aninput
event listener combined with a timer to execute actions after a typing pause. - Utilizing Event Debouncing: Debouncing optimizes event handling by delaying function execution until a specified time has passed since the last fired event, effectively detecting typing pauses.
- Leveraging the
keyup
Event Listener: By attaching a keyup event listener to an input field, we monitored key releases to identify typing pauses.
Leave a Reply