Generally, we navigate to different pages of a website by clicking the provided buttons on the web page. But, sometimes there is no back button provided on the web pages. In that case, we use the browser back and forward buttons to navigate through the different pages of the website. In this tutorial, we are going to learn about the different ways in which we can detect the browser’s back and forward button clicks while navigating the website.
The different ways of detect the browser back button event in JavaScript
- By using the
hashchange
event - Using the
popstate
event - Using
popstate
with the history.pushState() method
Let us now discuss all the above listed methods in details.
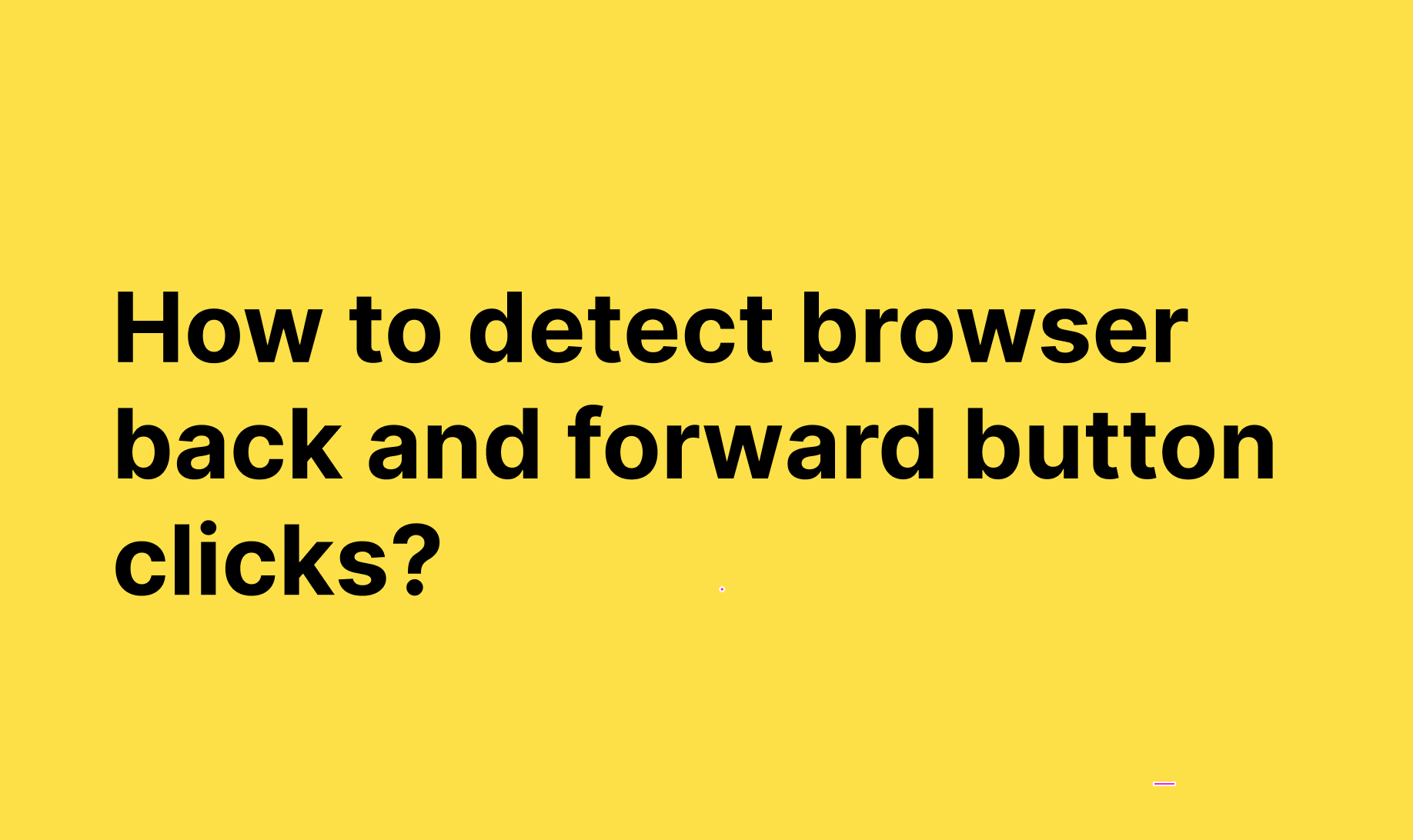
Using the hashchange
event:
The hashchange
event in JavaScript is a window event that occurs whenever there is a change in the history of the navigation state of the website. This property of the hashchange
event makes it able to detect the backward or the forward button click. We can implement this approach as shown in the below code example.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Document</title>
</head>
<body>
<button id="btn"> <a href="#title">Click the link to change the URL</a></button>
<h2 id="title">How to detect browser back and forward button clicks</h2>
<p class="result"></p>
<script>
window.addEventListener('hashchange', function(){
console.log("User clicked the browser buttons. Detected using hashchange event.");
});
</script>
</body>
</html>
Using the popstate
event:
The popstate event is an event that is triggered by the forward or
backward click or whenever there is a change in the session history. The popstate
event can help us detect the browser button clicks by using the below code.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Document</title>
</head>
<body>
<button id="btn"> <a href="#title">Click the link to change the URL</a></button>
<h2 id="title">How to detect browser back and forward button clicks</h2>
<p class="result"></p>
<script>
window.addEventListener('popstate', function(event){
if(event.state){
console.log("User clicked the browser buttons. Detected using popstate event.");
}
});
</script>
</body>
</html>
Using popstate
event with the history.pushState() method:
The purpose of using the pushState with the popstate
event is that we will manually push the new entry to the session history by clicking on a button or link on the webpage. It makes the popstate event trigger even if we are on the same page. Because of the entry changes in the session history that we made using the history.pushState() method.
The below example will help you understand the practical implementation of this approach.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Document</title>
</head>
<body>
<button id="btn"> <a href="#title">Click the link to change the URL</a></button>
<h2 id="title">How to detect browser back and forward button clicks</h2>
<p class="result"></p>
<script>
var btn = document.getElementById('btn');
btn.addEventListener('click', function(){
window.history.pushState({}, null, null);
})
window.addEventListener('popstate', function(){
console.log("User clicked the browser buttons popstate");
});
</script>
</body>
</html>
In this tutorial, we have learned about the three different ways of detecting the forward and the backward button clicks in the browser window.
Also please check our other javascript tutorials.
☞ How to convert array to space separated strings in javascript
☞ How to convert array to comma separated strings in javascript
Leave a Reply