In this tutorial, we’ll explore how to dynamically create an image element using JavaScript, enabling us to manipulate and control images on a webpage. This process allows for the seamless addition of images to a webpage through scripting, giving us more flexibility and control. We’ll see the step-by-step process of creating, modifying, and incorporating these image elements into your web projects:
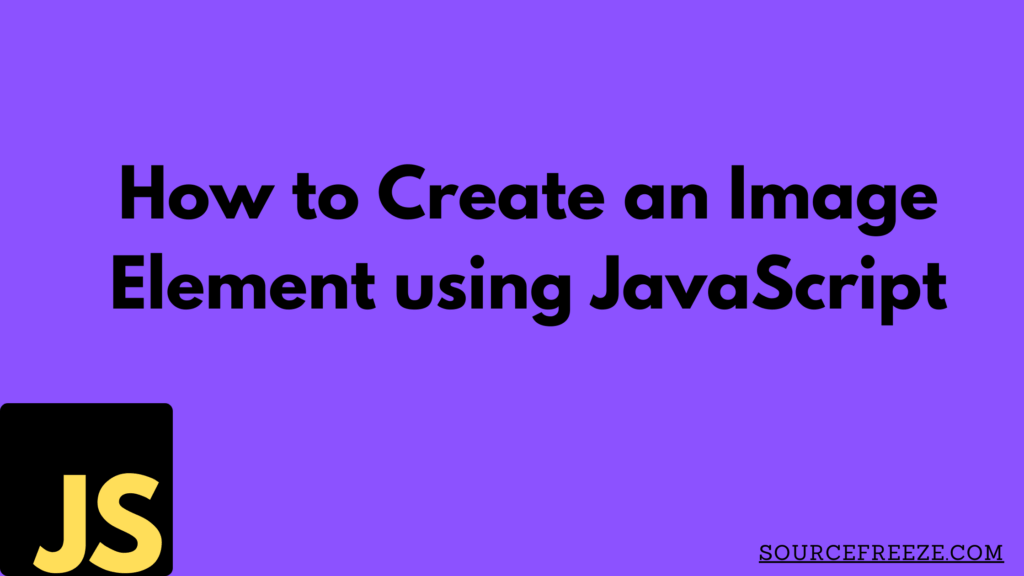
What is an Image Element?
An image element, represented in HTML by the <img>
tag is a crucial component for displaying images on web pages. It allows the seamless integration of visual content into a webpage’s layout. This element enables the inclusion of images from various sources, such as local files or URLs, enhancing the user experience and visual appeal of a website. Let’s start with the first method:
I. Create Image Element using JavaScript
A. Using createElement() Method:
In JavaScript, the createElement()
method is employed to dynamically generate HTML elements, including image elements. We can create an image element using this method and then further customize its attributes and properties as needed.
// Create a new image element
const imgElement = document.createElement('img');
B. Setting Attributes with setAttribute()
Once the image element is created, we can set its attributes such as src
, alt
, width
, and height
using the setAttribute()
method.
// Set attributes for the image element
imgElement.setAttribute('src', 'path/to/image.jpg');
imgElement.setAttribute('alt', 'Description of the image');
imgElement.setAttribute('width', '300');
imgElement.setAttribute('height', '200');
This allows us to dynamically assign various properties to the image element before appending it to the webpage’s Document Object Model (DOM).
Let’s move on to the next section, “Manipulating Image Elements,” to explore how to incorporate these dynamically created images into the webpage and make further modifications.
II. Manipulating Image Element using JavaScript
A. Appending Images to the DOM:
To display the dynamically created image on a webpage, we need to append it to the Document Object Model (DOM). This involves selecting an existing HTML element where the image will be placed.
// Select the target element where the image will be appended
const targetElement = document.getElementById('imageContainer');
// Append the image element to the target element
targetElement.appendChild(imgElement);
By appending the image element to a designated container in the DOM, we ensure its display within the web page’s structure.
B. Modifying Image Properties Dynamically
JavaScript allows us to dynamically modify image properties based on user interactions or specific events. For instance, changing the image source or altering its dimensions on the fly.
// Change the image source dynamically
imgElement.setAttribute('src', 'new/path/to/updated-image.jpg');
// Modify image dimensions
imgElement.setAttribute('width', '400');
imgElement.setAttribute('height', '300');
These dynamic modifications enable us to adapt images in real time without requiring manual HTML adjustments.
Conclusion
In this tutorial, we’ve explored how to dynamically create and manipulate image elements using JavaScript. Understanding the image element’s role in web development and utilizing JavaScript’s capabilities to create, customize, and integrate images into webpages empowers developers to enhance user experiences.
Leave a Reply