In the world of web development, JavaScript serves as the backbone for interactivity. Integrating video content seamlessly into web pages enhances user experience. Today, we’ll delve into the process of creating a video element using JavaScript. This involves using JavaScript to dynamically add and control a video player on a web page.
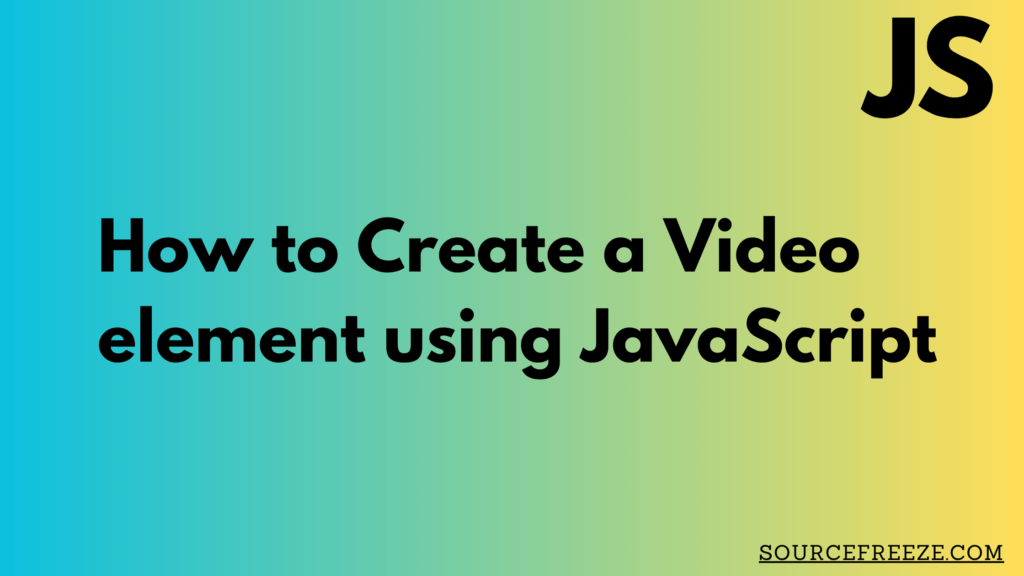
Adding the Video Element to HTML:
To begin, let’s set up our HTML file. We’ll create a container in the body where our video element will reside:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Video Element Creation</title>
</head>
<body>
<div id="video-container"></div>
<script src="your-javascript-file.js"></script>
</body>
</html>
JavaScript Code:
Now, we’ll dive into the JavaScript code that will dynamically create and configure the video element within our designated container:
// Select the container element
const videoContainer = document.getElementById('video-container');
// Create the video element
const video = document.createElement('video');
// Set video attributes
video.src = '/media/Huberman Lab.mp4';
video.controls = true;
video.width = '1920';
video.height = '1080';
// Append the video element to the container
videoContainer.appendChild(video);
Explanation of the Code:
- Selecting the Container: We use
document.getElementById
to select the container where the video will be added. - Creating the Video Element:
document.createElement('video')
generates the video element. - Setting Attributes: Configuring essential attributes like
src
(the video file’s path),controls
(to display player controls), and dimensions (width
andheight
) for the video element. - Appending to the Container: Finally, using
appendChild
, we add the video element to the designated container.
This sets up a basic structure for adding a video element to a webpage using JavaScript. In the next segment, we’ll explore more advanced functionalities and controls for the video element.
Here’s how an embedded video looks like with the basic controls
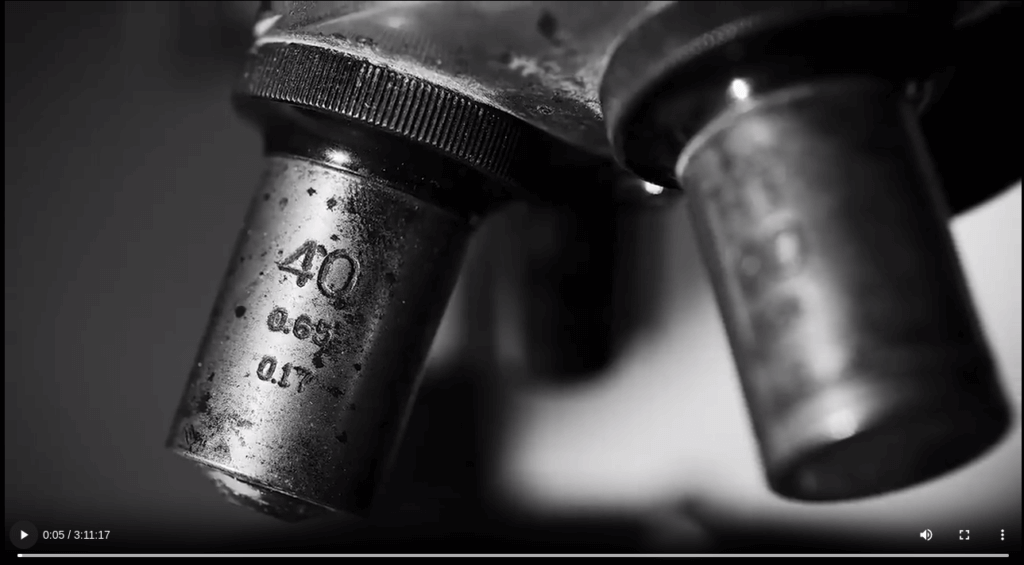
Exploring Video Element Attributes:
There’s a multitude of additional attributes and options that can enhance the video player’s functionality and appearance. Some of these attributes include:
- Autoplay: Allows the video to play automatically when the page loads.
- Loop: Enables continuous playback of the video.
- Poster: Defines an image to display while the video is downloading or until the user hits play.
- Preload: Specifies whether the video should be loaded when the page loads.
- Muted: Allows the video to play without sound.
These attributes, among others, offer developers flexibility in customizing the behavior of the video element according to specific requirements. To explore a comprehensive list of attributes and their usage, check out the Mozilla Developer Network’s documentation on the <video> element.
Conclusion
In this article, we’ve delved into creating dynamic video elements using JavaScript. By exploring attributes like autoplay, loop, and more, developers can tailor video experiences for enhanced engagement.
Thanks for stopping by! Check out more from Source FreezeĀ here!
Leave a Reply