To Convert array to comma separated strings in Javascript first need to pass the array to the String object like String(arr), then the String(arr) will return the comma separated strings as an output.
Please refer to the below example here we have passed the arrValue=[“javascript”,”typescript”,”react”,”angular”] to the String object, then the String object will return the values as comma separated output like ‘javascript,typescript,react,angular’
The String object is used to represent and manipulate a sequence of characters.
let arrValue = ['javascript', 'typescript', 'react', 'angular'];
let strValue = String(arrValue);
console.log(strValue); // 'javascript,typescript,react,angular'
console.log(typeof strValue); // string
Alternatively, we can use the Array.prototype
.join()
method, this Array.join()
method is used to join(
) the all the array with the specified characters.
To convert array to comma separated strings in javascript we can use the join()
method of an array, by passing the comma as a parameter. the Array.join()
method returned the comma separated strings as an output.
Please refer to the below example we have passed the arrValue=[“javascript”,”typescript”,”react”,”angular”] to the arrValue to join()
method of array, then join() method will return the values as comma separated output. e.g ‘javascript,typescript,react,angular’
The join()
method creates and returns a new string by concatenating all of the elements in an array
let arrValue = ['javascript', 'typescript', 'react', 'angular'];
const strValue = arrValue.join(',');
console.log(strValue); // 'javascript,typescript,react,angular'
console.log(typeof strValue); // string
the Array.prototype
.join()
will take the specified separator as the parameter in our case we have used a comma as a separator but if want to use a different separator we can use it it will be separated based on the specified separator character for example space, #, or whatever you want.
**************
Related Post: Convert Array to Space Separated Strings
*************
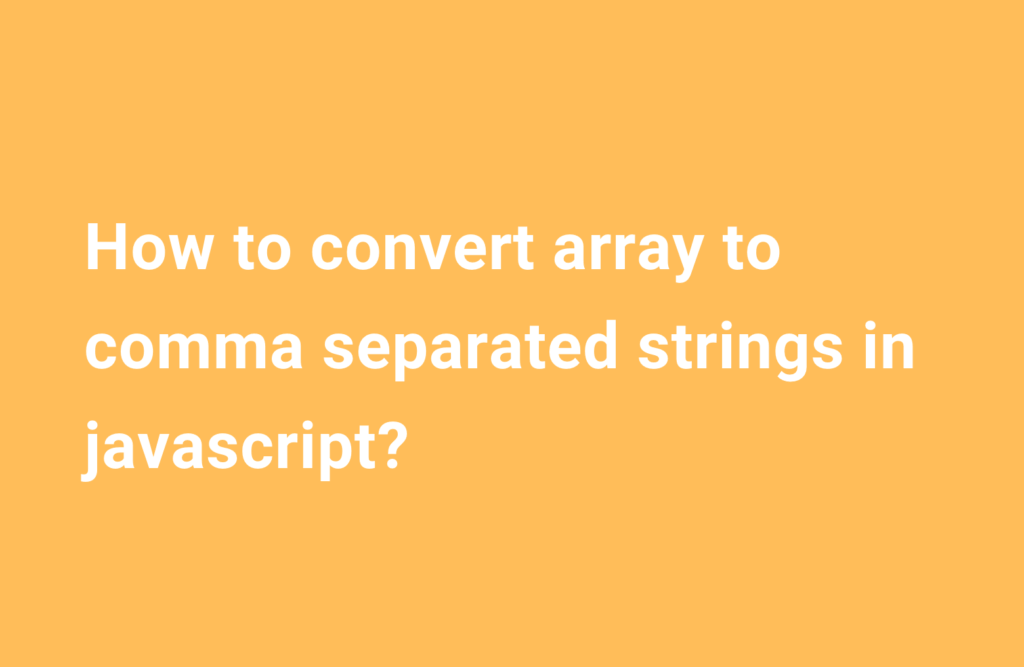
Leave a Reply