This article provides you with a comprehensive guide on how to convert an array to a map in JavaScript. We will explore different methods and their benefits, ensuring you have the right tools for your specific needs.
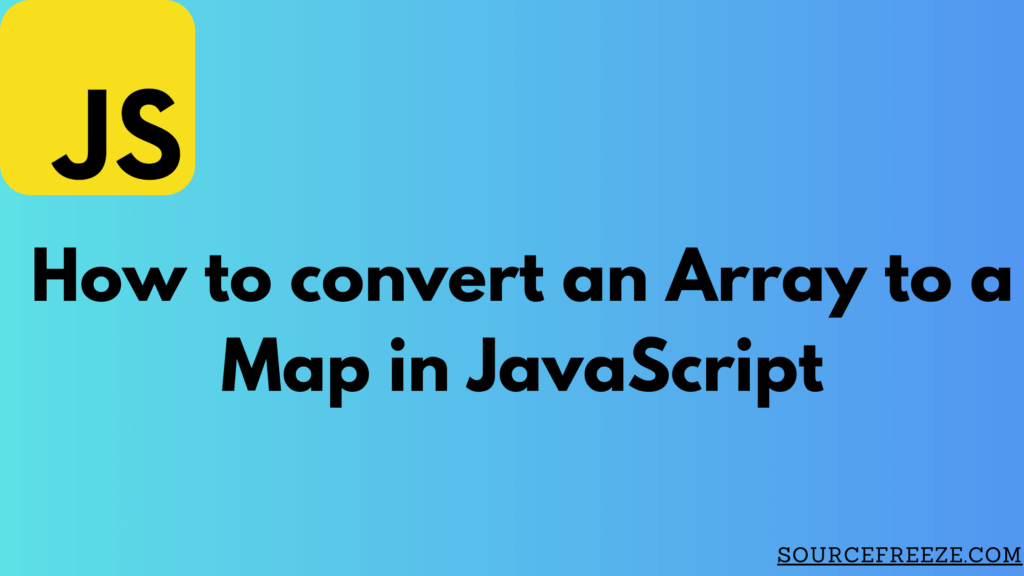
Methods for Conversion:
- Using the Map Constructor
- Using Array.prototype.reduce()
- Utilizing Array.prototype.forEach()
- Leveraging for…of Loop
JavaScript offers multiple ways to convert an array to a map in JavaScript. Let us see the first method in detail.
Using the Map Constructor
One of the most direct methods to convert an array into a map in JavaScript involves the Map constructor. This constructor allows us to create a new Map instance from an array.
Here’s a step-by-step breakdown of how we can achieve this:
const myArray = [['key1', 'value1'], ['key2', 'value2']];
const myMap = new Map(myArray);
Explanation:
- Create an Array: First, we have an array, myArray, which contains sub-arrays, each representing a key-value pair.
- Use the Map Constructor: We utilize the Map constructor and pass myArray as an argument. The constructor accepts an iterable of key-value pairs ([key, value]) where each pair becomes an entry in the map.
Example:
const fruits = [['apple', 5], ['orange', 8], ['banana', 3]];
const fruitMap = new Map(fruits);
console.log(fruitMap);
// Output: Map(3) {"apple" => 5, "orange" => 8, "banana" => 3}
Here we’ll get 2 advantages:
- Preservation of Order: The Map object retains the order of the original array
- Unique Keys: Keys within a map are unique; if duplicate keys are found, the last key-value pair overwrites previous entries.
Using Array.prototype.reduce()
The reduce() method in JavaScript is powerful for transforming data structures. Here’s how we can use it to convert an array into a map in javascript:
const myArray = [['key1', 'value1'], ['key2', 'value2']];
const myMap = myArray.reduce((acc, [key, value]) => {
acc.set(key, value);
return acc;
}, new Map());
Explanation:
- Initialize with new Map(): We start by initializing an empty Map.
- Iterate Using reduce(): The reduce() method operates on each element of the array.
- Set Key-Value Pairs: Within the reducer function, we use acc.set(key, value) to set key-value pairs in the map.
Example:
const animals = [['lion', 'roar'], ['elephant', 'trumpet'], ['giraffe', 'bleat']];
const soundMap = animals.reduce((acc, [animal, sound]) => {
acc.set(animal, sound);
return acc;
}, new Map());
console.log(soundMap);
// Output: Map(3) {"lion" => "roar", "elephant" => "trumpet", "giraffe" => "bleat"}
The use of reduce() offers a flexible way to convert arrays into maps, especially when custom logic or complex transformations are needed.
Utilizing Array.prototype.forEach()
The forEach() method in JavaScript provides a way to iterate through elements in an array. Although it doesn’t directly produce a map, we can use it to create one:
const myArray = [['key1', 'value1'], ['key2', 'value2']];
const myMap = new Map();
myArray.forEach(([key, value]) => {
myMap.set(key, value);
});
Explanation:
- Initialize a Map: We start by creating an empty Map.
- Iterate with forEach(): Loop through each element of the array.
- Set Key-Value Pairs: Inside the forEach loop, use myMap.set(key, value) to add elements to the map.
Example:
const cities = [['NY', 'New York'], ['SF', 'San Francisco'], ['LA', 'Los Angeles']];
const cityMap = new Map();
cities.forEach(([code, name]) => {
cityMap.set(code, name);
});
console.log(cityMap);
// Output: Map(3) {"NY" => "New York", "SF" => "San Francisco", "LA" => "Los Angeles"}
The advantage we’ll get for using forEach() is that it is explicit and easy to understand for simple transformations.
Leveraging for…of Loop
The for…of loop in JavaScript provides an elegant way to iterate through elements in an array and create a map:
const myArray = [['key1', 'value1'], ['key2', 'value2']];
const myMap = new Map();
for (const [key, value] of myArray) {
myMap.set(key, value);
}
Explanation:
- Initialize a Map: We again start by creating an empty Map.
- Use for…of Loop: The loop iterates through the elements of the array.
- Set Key-Value Pairs: Inside the loop, myMap.set(key, value) adds elements to the map.
Example:
const colors = [['red', '#FF0000'], ['green', '#00FF00'], ['blue', '#0000FF']];
const colorMap = new Map();
for (const [name, hexCode] of colors) {
colorMap.set(name, hexCode);
}
console.log(colorMap);
// Output: Map(3) {"red" => "#FF0000", "green" => "#00FF00", "blue" => "#0000FF"}
Advantages:
- Readable Iteration: for…of offers a concise and readable way to loop through arrays.
- Manual Map Population: Similar to forEach(), this method requires explicit setting of key-value pairs.
Conclusion:
Each method has its advantages, offering flexibility and readability based on specific use cases. The resulting maps retain key-value associations, allowing for easy access and manipulation of data.
By understanding these methods, we can efficiently convert an array to a map in JavaScript, leveraging the chosen technique based on the complexity and requirements of their tasks in JavaScript programming.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply