JavaScript, as a versatile language, offers various methods to manipulate and combine numbers effortlessly. When it comes to concatenating or merging two numbers together, understanding the right approach can make a significant difference. In this guide, we’ll learn how to concatenate two Numbers in JavaScript
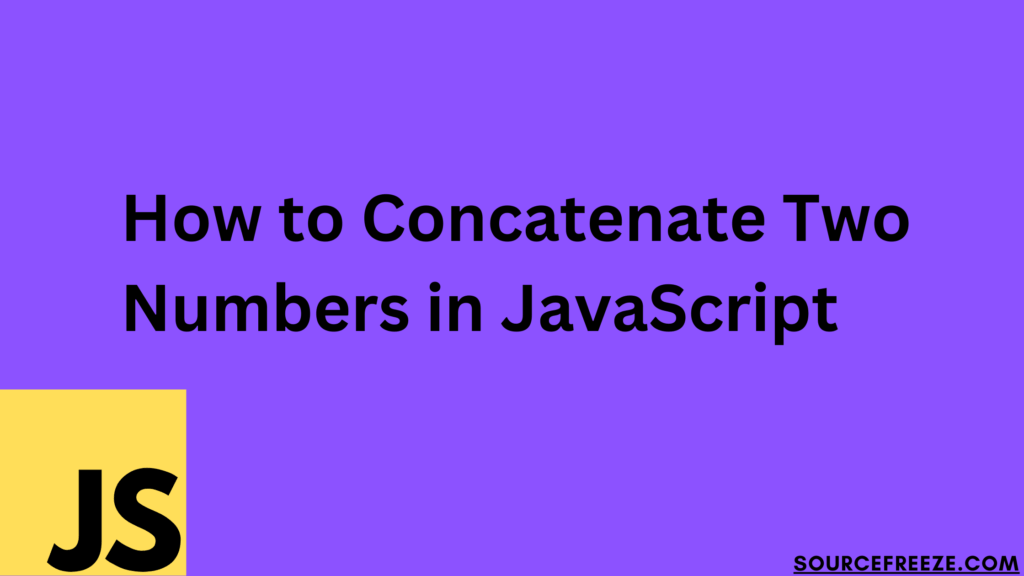
Using String Conversion for Concatenation
One of the most straightforward ways to concatenate numbers in JavaScript involves converting them to strings and then merging these strings. Here’s a practical example:.
let num1 = 23;
let num2 = 5;
let concatenated = num1.toString() + num2.toString();
console.log(concatenated);
Output:
"235"
Conversion using ‘+’
When you use the `+
` operator between strings and numbers, it implicitly convert the numbers into strings before concatenation:
let a = 30;
let b = 70;
let result = "The sum is: " + a + b;
console.log(result);
In this scenario, JavaScript automatically converts a
and b
into strings before appending them to the string “The sum is: “. The final output becomes:
The sum is: 3070
Template Literals for Concatenation
Template literals offer a modern and concise approach to concatenating numbers within strings. They provide a more readable and flexible way to embed variables into strings.
Consider this example:
let x = 15;
let y = 35;
let message = `The concatenated result is: ${x}${y}`;
console.log(message);
Here, ${x}
and ${y}
within the backticks are placeholders for the variables x
and y
. These placeholders directly embed the values of x
and y
into the resulting string, creating the output as:
The concatenated result is: 1535
Template literals help when you need to create complex strings with embedded variables or expressions. They are especially handy for generating dynamic strings in functions, error messages, or any context where string interpolation is beneficial.
Array Join Method for Number Concatenation
JavaScript’s Array.join()
method provides an alternative way to concatenate numbers by leveraging arrays.
Consider the following example:
let numberArray = [1, 2, 3];
let concatenatedString = numberArray.join('');
console.log(concatenatedString);
In this example, numberArray
contains three numerical values: 1, 2, and 3. The join('')
method concatenates these numbers by converting them into strings and joining them together with an empty string as the separator. The resulting string is:
123
Conclusion:
In this blog, we explored various methods for concatenating numbers in JavaScript. We learned about:
- Using toString() for straightforward conversions,
- Conversion using ‘+’,
- Leveraging template literals for readable embedding, and
- Applying Array.join() to concatenate arrays efficiently.
Leave a Reply