In this blog, we will explore the topic of retrieving the current URL in Next.js, a powerful React framework. Understanding how to obtain the current URL is crucial for various web development scenarios. We’ll explore the steps to achieve this and provide real life code examples to get the current url in Nextjs.
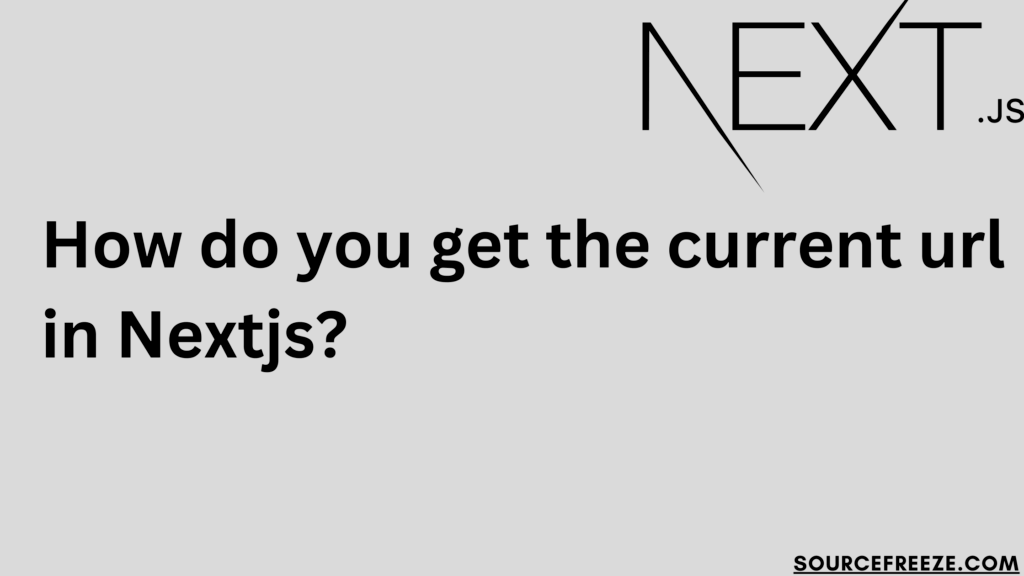
Getting Started:
To kick things off, let’s understand why obtaining the current URL is important in web development. Often, we encounter situations where we need to dynamically update content based on the URL or perform specific actions. In Next.js, achieving this is straightforward, and we will guide you through the process.
Using useRouter Hook for Client-Side Access
The useRouter
hook is a cornerstone for client-side URL access in Next.js. Import it using import { useRouter } from 'next/router'
to utilize its functionalities within your components.
import { useRouter } from 'next/router'
function YourComponent() {
const { asPath, pathname } = useRouter();
// Your component logic
return <h2>Your Component</h2>
}
Here, asPath
holds the full page path, while pathname
encapsulates the page’s specific path, even with dynamic routing parameters.
The useRouter hook serves as a fundamental tool for accessing URL details on the client side within Next.js components.
Breakdown:
asPath
: Represents the complete URL path, including query parameters and hash fragments. It’s valuable for capturing the entire route a user is visiting.pathname
: Indicates the specific page path, even with dynamic routing parameters. This property is useful for targeting specific routes within your application.
Practical Use:
- Dynamic Page Rendering: Determine the specific route or page being accessed by extracting data from
asPath
orpathname
. - Conditional Rendering: Implement conditional logic based on the current URL path or query parameters using these properties.
Unveiling URL Details on the Server
In scenarios requiring Server-Side Rendering (SSR) through getServerSideProps
, accessing URL information involves different approaches. Leveraging req.url
or query
within the getServerSideProps
the function offers insights into the URL structure. Moreover, the resolvedUrl
property provides the URL without the _next/data
prefix, vital for client transitions.
export async function getServerSideProps({ req, query, resolvedUrl }) {
console.log(req, query, resolvedUrl)
return { props: {} }
}
Breakdown:
req.url
: Provides the path, similar to theasPath
on the client side.query
: Contains query strings and path parameters.resolvedUrl
: Offers the URL without the_next/data
prefix, enabling smoother client transitions.
Practical Use:
- Server-Side Data Fetching: Fetch data based on the URL parameters or path accessed during server-side rendering.
- URL Transformation: Manipulate the URL for specific server-side functionalities or API interactions based on the accessed route.
Static Site Generation and URL Retrieval
For Static Site Generation (SSG) via getStaticProps
, the params
object holds the defined parameters from the path. This method grants access to crucial parameters, allowing precise handling of page generation.
export async function getStaticProps({ params }) {
console.log(params)
return { props: {} }
}
Breakdown:
params
: Holds parameters defined in the URL path, aiding in precise page generation.
Practical Use:
- Dynamic Page Generation: Generate static pages based on URL parameters, ensuring dynamic content for specific routes.
- Custom Page Rendering: Craft pages with dynamic content fetched based on URL parameters for a personalized user experience.
Conclusion
In this blog, we delved into comprehensive methods of obtaining the current URL in Next.js, covering:
- Using useRouter Hook for Client-Side Access
- Unveiling URL Details on the Server
- Static Site Generation and URL Retrieval
Understanding these distinct methods for obtaining URLs in Next.js empowers developers to employ diverse strategies for client-side interactivity, server-side data retrieval, and dynamic page generation.
Leave a Reply