In the world of JavaScript and web development, accessing elements using specific classes is a common task. We’ll explore how to find these elements that have more than one class and how to spot those with one class but not another. Let’s learn the simple yet powerful methods to get Elements by multiple Class Names using JavaScript
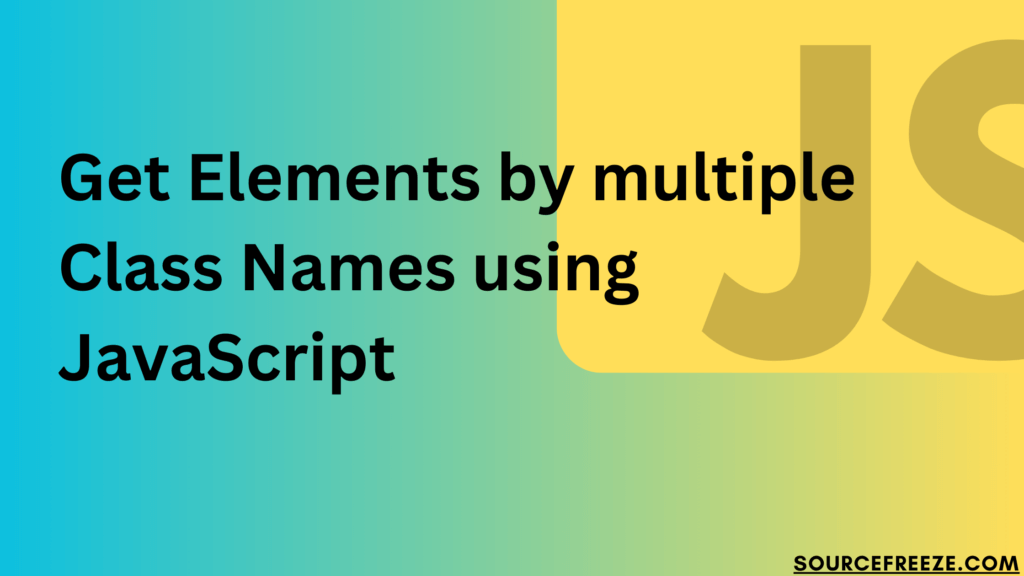
Converting Elements to an Array
To begin, when we select elements by multiple class names, the retrieved result isn’t an array but rather a collection or NodeList. This collection might resemble an array, but it lacks the extensive functionality and methods native to arrays.
How to Convert NodeList to Array
The process is straightforward. We’ll use the Array.from()
method, a powerful utility in JavaScript that allows us to convert an array-like object (such as a NodeList) into a true array.
// Selecting elements by multiple class names
const elements = document.querySelectorAll('.class1.class2');
// Converting NodeList to an array
const elementsArray = Array.from(elements);
Utilizing querySelectorAll
JavaScript’s querySelectorAll
method is a powerful tool for selecting elements based on multiple class names directly without converting them into an array. This method also returns a NodeList, an array-like object, containing all the elements that match the specified selectors.
// Selecting elements by multiple class names
const elements = document.querySelectorAll('.class1.class2');
// Elements are accessible in a NodeList
The querySelectorAll method streamlines the selection process by accepting a CSS-style selector as an argument, allowing us to target elements with specific classes comprehensively.
To manipulate elements within the NodeList directly, we utilize methods like forEach
, item
, or array-like indexing.Here’s an example:
// Iterating through elements in the NodeList
elements.forEach(element => {
// Perform operations on each element
});
getElementsByClassName
The getElementsByClassName
method is a native JavaScript function that allows us to fetch elements by their class names directly without the need for complex selectors.
// Selecting elements by multiple class names
const elements = document.getElementsByClassName('class1 class2');
// Elements are accessible in an HTMLCollection
This method directly fetches elements with the specified class names, returning an HTMLCollection, another array-like object similar to a NodeList.
Utilizing HTMLCollection Properties
Similar to NodeLists, HTMLCollections have their unique properties. They’re live collections, updating as the DOM changes, and can be accessed using methods like item
or array-like indexing.
example code for accessing elements within the HTMLCollection.
// Accessing elements within the HTMLCollection
const firstElement = elements[0]; // Using array-like indexing
const secondElement = elements.item(1); // Using item method
Let’s continue exploring ways to identify elements based on multiple classes, including scenarios where an element has at least one of multiple classes or has one class but not the other.
Finding the First Element with Multiple Classes
To locate the first element with multiple classes, we can leverage array methods in conjunction with querySelector
. For instance, to find the first element with both class1
and class2
:
// Finding the first element with both class1 and class2
const firstElement = document.querySelector('.class1.class2');
This method selects the first element that encompasses both specified classes, granting direct access to that particular element.
Selecting Elements with One Class but Not the Other
To target elements with one class while excluding another, we can use the :not()
CSS pseudo-class within the querySelectorAll
method.
// Selecting elements with class1 but not class2
const elementsWithClass1 = document.querySelectorAll('.class1:not(.class2)');
This query retrieves all elements with class1
but excludes those that also have class2
.
Conclusion
In this blog, we’ve navigated JavaScript’s powerful tools for finding elements by class names. We’ve explored methods like:
- Converting to Array
- Using querySelectorAll
- getElementsByClassName
Alongside we also learned their applications, these methods allow us to precisely target elements with multiple classes or specific class combinations.
Leave a Reply