When working with JavaScript, there are various methods to convert map keys and values into an array, in this tutorial we will how to convert map keys and values to an array in javascript
The primary techniques which we will explore in this blog include:
- Using Array.from()
- Using forEach() method
- Using for…of loop
- Using spread operator
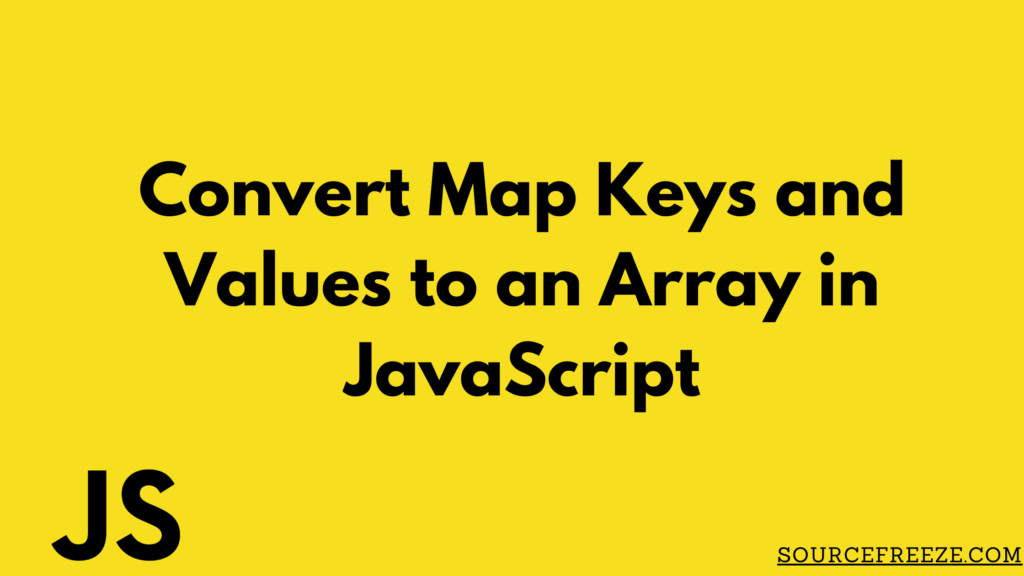
Let’s see the details of the first method, which involves the Array.from() approach to convert map keys and values into an array.
Method 1: Using Array.from()
The Array.from() method in JavaScript creates a new, shallow-copied array instance from an array-like or iterable object. When applied to a Map object, it extracts the keys, values, or both, and constructs an array accordingly.
Syntax of Array.from():
Array.from(map.keys());
Array.from(map.values());
Array.from(map.entries());
Example:
Suppose we have a Map containing some values for the keys:
let myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
Now, we’ll use Array.from() to extract keys and values:
// Extracting keys from map
let keysArray = Array.from(myMap.keys());
console.log(keysArray); // Output: ['key1', 'key2', 'key3']
// Extracting values
let valuesArray = Array.from(myMap.values());
console.log(valuesArray); // Output: ['value1', 'value2', 'value3']
Explanation:
- Array.from() takes an iterable object, in this case, map.keys() or map.values().
- It constructs a new array based on the elements retrieved from the Map object.
- The resulting arrays contain the keys or values extracted from the Map.
The Array.from() method provides a straightforward way to convert Map keys and values into arrays, offering flexibility and ease of use. In the next segments, we’ll explore additional methods for achieving the same conversion.
Method 2: Using forEach() Method
The forEach() method in JavaScript allows iteration through the elements of an array, and we can utilize it to convert Map keys and values into separate arrays.
Syntax of forEach():
let keysArray = [];
let valuesArray = [];
map.forEach((value, key) => {
keysArray.push(key);
valuesArray.push(value);
});
Example:
Consider the same Map we used previously:
let myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
Now, let’s convert the Map keys and values into arrays using forEach():
let keysArray = [];
let valuesArray = [];
myMap.forEach((value, key) => {
keysArray.push(key);
valuesArray.push(value);
});
console.log(keysArray); // Output: ['key1', 'key2', 'key3']
console.log(valuesArray); // Output: ['value1', 'value2', 'value3']
Explanation:
- We initialize empty array keysArray and valuesArray to store the keys and values, respectively.
- The forEach() method iterates through each key-value pair in the Map.
- For each iteration, it pushes the key into the keysArray and the value into the valuesArray.
- Finally, we get separate arrays containing the keys and values from the Map.
Let’s proceed with exploring the third method to convert Map keys and values into arrays.
Method 3: Using for…of Loop
The for...of loop
in JavaScript provides a concise way to iterate over iterable objects, including Map objects. We can utilize this loop to extract keys and values from a Map and create arrays.
Syntax for the loop:
let keysArray = [];
let valuesArray = [];
for (let [key, value] of map) {
keysArray.push(key);
valuesArray.push(value);
}
Example:
Using the same Map as before:
let myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
Let’s convert the Map keys and values into arrays using a for...of
loop:
let keysArray = [];
let valuesArray = [];
for (let [key, value] of myMap) {
keysArray.push(key);
valuesArray.push(value);
}
console.log(keysArray); // Output: ['key1', 'key2', 'key3']
console.log(valuesArray); // Output: ['value1', 'value2', 'value3']
Explanation:
- We declare empty arrays
keysArray
andvaluesArray
to store the keys and values. - The
for...of
loop iterates through the entries of the Map, providing both the key and value in each iteration. - During each iteration, it pushes the key into
keysArray
and the value intovaluesArray
. - As a result, we obtain separate arrays containing the keys and values extracted from the Map.
Method 4: Using the Spread Operator
The spread operator (...
) in JavaScript is a powerful tool for expanding elements, and it can be employed to convert Map keys and values into arrays by spreading them into new arrays.
Syntax for spread operator:
let keysArray = [...map.keys()];
let valuesArray = [...map.values()];
Example:
Considering the same Map:
let myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
myMap.set('key3', 'value3');
Let’s use the spread operator to convert the Map keys and values into arrays:
let keysArray = [...myMap.keys()];
let valuesArray = [...myMap.values()];
console.log(keysArray); // Output: ['key1', 'key2', 'key3']
console.log(valuesArray); // Output: ['value1', 'value2', 'value3']
Explanation:
- We use the spread operator (
...
) along withmap.keys()
andmap.values()
to directly convert the keys and values into arrays. - The spread operator spreads each element of the iterable object (keys or values) into a new array.
- This approach provides a concise and expressive way to transform Map keys and values into separate arrays.
Conclusion:
In this tutorial, we covered 4 different methods to Convert Map Keys and Values to an Array in JavaScript.
We explored Array.from()
, forEach()
,for...of
Loop & the Spread
Operator to achieve the desired result.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply