JavaScript presents an array of possibilities when it comes to dynamic webpage interactions. One such engaging feature is the ability to change the background color of an element upon a user’s click. In this guide, we’ll delve into the process step by step, exploring the code and methods involved to change the background color on click using JavaScript.
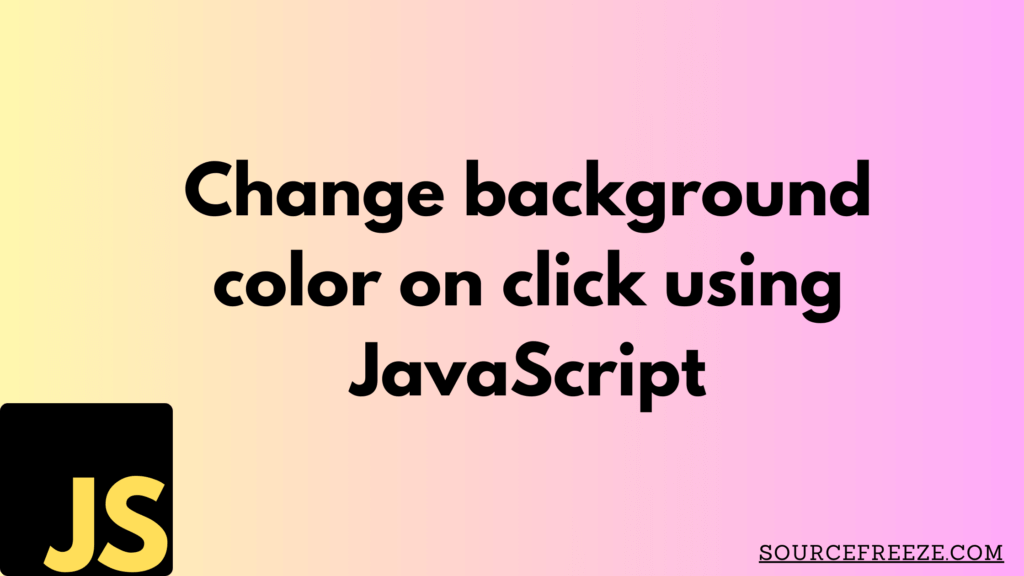
Our focus here revolves around altering the background color based on a user-triggered event, providing an interactive experience.
Let’s dive into the practical implementation.
Setting up the HTML Structure:
To begin, we’ll need an HTML file to work with. We’ll create a basic structure with a button that, when clicked, will change the background color of the webpage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Change Background Color on Click</title>
<style>
body {
transition: background-color 0.5s ease;
text-align: center;
font-family: Arial, sans-serif;
}
.change-btn {
padding: 10px 20px;
margin-top: 20px;
cursor: pointer;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
}
</style>
</head>
<body>
<h2>Changing Background Color on Click</h2>
<button class="change-btn">Change Background Color</button>
<script>
// JavaScript code will go here
</script>
</body>
</html>
Now, let’s proceed with the JavaScript part, wherein we’ll define the functionality to change the background color upon clicking the button.
Adding the JavaScript code to change background color
Inside the <script>
tag in our HTML file, we’ll write JavaScript code that will detect the button click and change the background color accordingly.
<script>
// Selecting the button element
const changeBtn = document.querySelector('.change-btn');
// Function to generate a random color
function getRandomColor() {
const letters = '0123456789ABCDEF';
let color = '#';
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
// Event listener for button click
changeBtn.addEventListener('click', function() {
// Get a random color
const newColor = getRandomColor();
// Change the background color of the body
document.body.style.backgroundColor = newColor;
});
</script>
Explanation:
We select the button element using
document.querySelector('.change-btn')
.The
getRandomColor()
function generates a random hex color code to be used as the new background color.The event listener is added to the button using
addEventListener()
, which triggers a function when the button is clicked.On each click, a new random color is generated and applied to the
backgroundColor
of thebody
.
More methods:
Changing Element Color on Click:
To change the color of an element, such as a heading or a paragraph, we can modify our JavaScript code as follows:
// Selecting the element to be changed
const elementToChange = document.querySelector('.element-to-change');
// Event listener for button click
changeBtn.addEventListener('click', function() {
// Get a random color
const newColor = getRandomColor();
// Change the color of the selected element
elementToChange.style.color = newColor;
});
Changing Button Color:
Alternatively, let’s modify the code to change the color of the button itself:
// Event listener for button click
changeBtn.addEventListener('click', function() {
// Get a random color
const newColor = getRandomColor();
// Change the button's background color
changeBtn.style.backgroundColor = newColor;
});
This adjustment will alter the background color of the button upon each click, providing a dynamic visual effect.
Conclusion
In this blog, we’ve explored how JavaScript enables dynamic webpage interactions by changing background colors on button clicks. We’ve seen how a few lines of code can create engaging experiences and learned to adapt this technique for diverse elements.
Leave a Reply