In this comprehensive guide, we’ll see the efficient JavaScript methods for capitalizing the initial letter of strings. We’ll explore practical techniques using charAt, toUpperCase, and other essential string manipulation functions to capitalize the first letter.
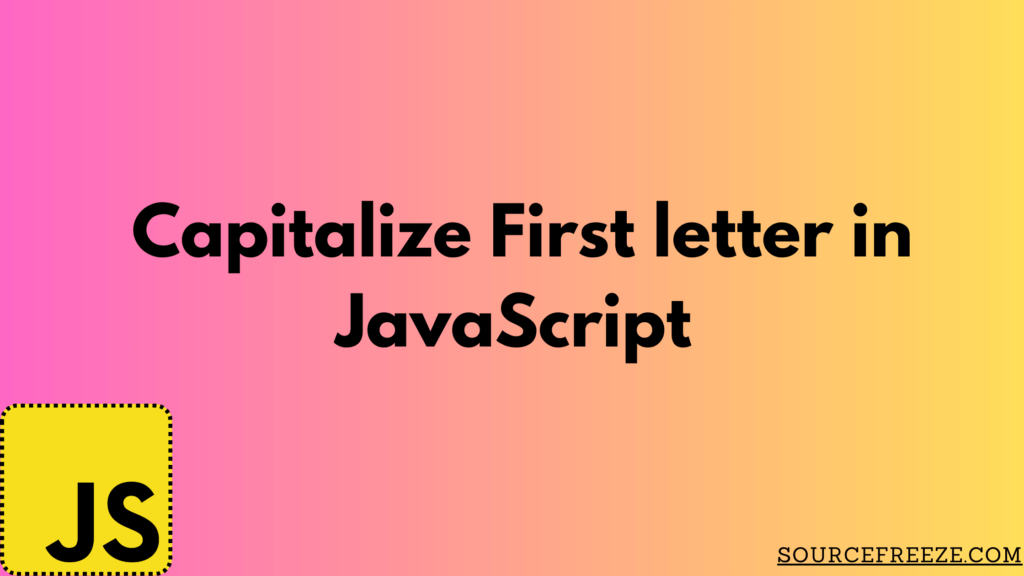
We’ll take a look at 3 methods in this blog:
1. Using charAt Function
2. Using the slice and toUpperCase Methods
3. Capitalizing First Letter of Each Word
Let’s get right into the first one:
Using charAt Function:
The charAt
function is utilized to extract the first character of a string. To capitalize the first letter, we can combine this method with other string manipulation functions.
Here’s a step-by-step guide on how to capitalize the first letter using charAt:
- Retrieve the first character: We’ll use the
charAt
function to get the first character of the string. - Convert the first character to uppercase: Once we’ve isolated the first character, we’ll use the
toUpperCase
method to convert it to uppercase. - Concatenate with the rest of the string: Finally, we’ll concatenate the capitalized first letter with the rest of the string, excluding the first character.
Let’s illustrate this with an example:
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
let text = "hello, world!";
let capitalizedText = capitalizeFirstLetter(text);
console.log(capitalizedText); // Output: "Hello, world!"
This function capitalizeFirstLetter takes a string input, capitalizes its first letter, and returns the modified string.
2. Using the slice and toUpperCase Methods:
This approach involves using the slice
method to isolate the first letter and the toUpperCase
method to capitalize it.
Here’s a step-by-step breakdown:
- Extract the first letter: Use the slice method to retrieve the first character of the string.
- Convert the first letter to uppercase: Apply the toUpperCase method to capitalize the isolated first character.
- Combine with the remaining string: Concatenate the capitalized first letter with the rest of the string, excluding the first character.
Here’s an example implementation:
function capitalizeFirstLetter(string) {
return string.slice(0, 1).toUpperCase() + string.slice(1);
}
let text = "hello, world!";
let capitalizedText = capitalizeFirstLetter(text);
console.log(capitalizedText); // Output: "Hello, world!"
In this function capitalizeFirstLetter, the slice method extracts the first character, the toUpperCase method capitalizes it, and the rest of the string is concatenated to form the modified string.
Capitalizing the first letter of each word in a string is a common requirement. One approach to achieving this is by utilizing the split
, map
, and join
methods along with string manipulation functions.
Capitalizing First Letter of Each Word:
Here’s a step-by-step guide:
- Split the string into an array of words: Use the
split
method to divide the string into an array of individual words. - Map through the array: Use the
map
method to iterate through each word in the array. - Capitalize the first letter: For each word, capitalize its first character using the
toUpperCase
method. - Join the modified words: Finally, use the
join
method to combine the capitalized words back into a single string.
Let’s illustrate this approach with an example:
function capitalizeEachWord(string) {
return string.split(' ').map(word => word.charAt(0).toUpperCase() + word.slice(1)).join(' ');
}
let text = "capitalize the first letter of each word";
let capitalizedText = capitalizeEachWord(text);
console.log(capitalizedText); // Output: "Capitalize The First Letter Of Each Word"
In the function capitalizeEachWord, the string is first split into an array of words using the space (‘ ‘) as a delimiter. Then, the map function iterates through each word, capitalizes the first letter of each word, and finally, the join method combines these modified words into a single string.
Conclusion:
In conclusion, JavaScript offers various methods to manipulate strings and capitalize their first letters. We explored three effective approaches:
- Using charAt function: This method extracts the first character of the string and capitalizes it using toUpperCase.
- Utilizing slice and toUpperCase methods: Here, the slice method isolates the first character, which is then capitalized using toUpperCase.
- Capitalizing each word: By splitting the string into an array of words, capitalizing the initial letter of each word, and joining them back together, we can capitalize every word’s first letter.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply