Append text to a textarea in JavaScript is a fundamental task, It involves manipulating the textarea’s value property to either append or verify if specific text exists within it. let us see step by step tutorial about how to append the text to textarea in javascript.
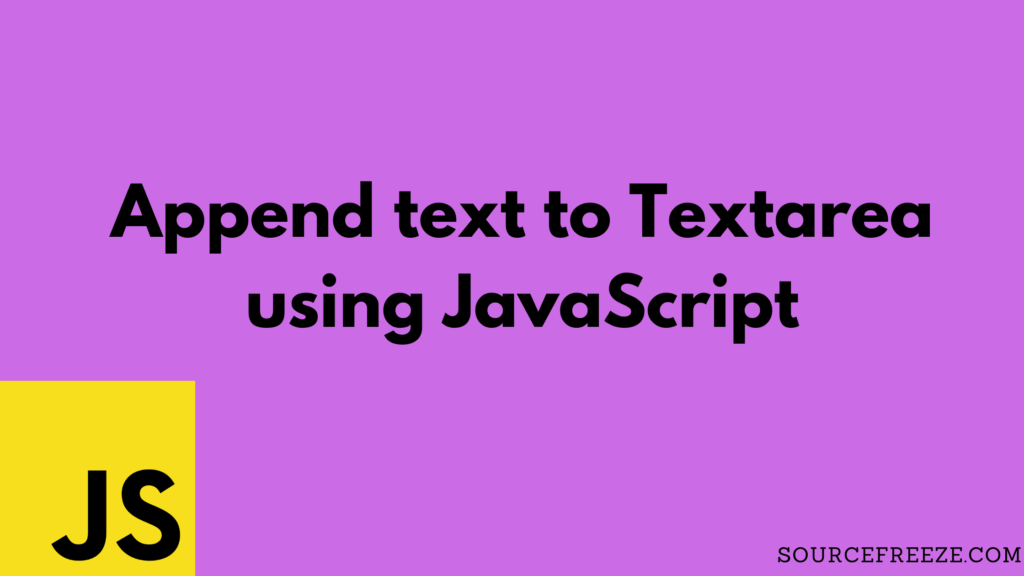
Here are the methods we’ll be exploring in this article:
- Appending Text to a Textarea
- Append Text on Button Click
- Checking if the Text is Already Contained
- Prepending Text
- Checking if the Textarea Ends with Text
Appending Text to a Textarea:
Appending text to a textarea in JavaScript is a common task. Here’s how we do it step by step:
Step 1: Access the Textarea
First, we need to access the textarea element from the HTML using JavaScript. We do this by selecting the textarea element using its id.
<textarea id="message" rows="5" cols="30"></textarea>
<button id="btn">Append text</button>
Step 2: JavaScript to Append Text
const textarea = document.getElementById('message');
This line gets the textarea element by its id (‘message’) and stores it in the textarea variable, allowing us to work with it in our code.
Step 3: Appending Text:
To append text to the textarea, we can use the value property of the textarea element. This property holds the text content of the textarea.
textarea.value += 'Appended text';
Here, we’ve added the text ‘Appended text’ to whatever text is already present in the textarea. This line works by retrieving the current value of the textarea and adding ‘Appended text’ to the end of that existing value.
This technique is useful when you want to dynamically add text to a textarea, such as when a button is clicked or when certain conditions are met which we’ll look into next…
Append Text on Button Click
Step 1: Access Elements
We start with the same HTML structure:
<textarea id="message" rows="5" cols="30"></textarea>
<button id="btn">Append text</button>
Step 2: JavaScript Event Handling
const textarea = document.getElementById('message');
const btn = document.getElementById('btn');
btn.addEventListener('click', function handleClick() {
textarea.value += 'Appended text';
});
Here, we’ve selected the textarea and the button using getElementById. Then, we added an event listener to the button using addEventListener.
When the button is clicked, the event listener triggers the handleClick function. Inside this function, we append the text ‘Appended text’ to the textarea.
By understanding this method, you can create interactive elements on your web pages that respond to user actions.
Checking if the Text is Already Contained
Step 1: Access Elements
We’ll use the same HTML setup with a textarea and a button:
<textarea id="message" rows="5" cols="30"></textarea>
<button id="btn">Append text</button>
Step 2: JavaScript for Text Validation
const textarea = document.getElementById('message');
const btn = document.getElementById('btn');
btn.addEventListener('click', function handleClick() {
const text = 'Text to append';
if (!textarea.value.includes(text)) {
textarea.value += text;
}
});
This way, when you click the button, it’ll only add the phrase ‘Text to append’ to the text area if it’s not already there. This helps avoid repeating the same information in the text area whenever the button is clicked.
Prepending Text:
Appending text is standard, but prepending requires a different approach. To add text at the beginning of the textarea, we can reverse the appending logic.
const textarea = document.getElementById('message');
const btn = document.getElementById('btn');
btn.addEventListener('click', function handleClick() {
const text = 'Text to prepend';
textarea.value = text + textarea.value;
});
With this method, the new text is placed at the beginning of the textarea content by concatenating it with the existing value.
Checking if the Value of the Textarea Ends with Text
Step 1: HTML Setup
We maintain the same HTML structure, comprising a textarea and a button:
<textarea id="message" rows="5" cols="30"></textarea>
<button id="btn">Append text</button>
Step 2: JavaScript Implementation
const textarea = document.getElementById('message');
const btn = document.getElementById('btn');
btn.addEventListener('click', function handleClick() {
const text = 'Text to append';
if (!textarea.value.endsWith(text)) {
textarea.value += text;
}
});
When we click the button, we’ll check whether the phrase ‘Text to append’ is already in the textarea. If it’s not there, we’ll add the phrase. This method helps prevent repeating the same information in the textarea every time we click the button.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply