In this blog, we’ll learn about JavaScript’s capability to modify URLs without the need for page reloads. Have you ever noticed how websites update the URL in your browser without fully refreshing the page? We’ll explore the powerful tools provided by JavaScript—such as the History and Location APIs—that allow us to tweak URLs dynamically. By the end, you’ll have a clear understanding of how to modify url without reloading the page using javascript.
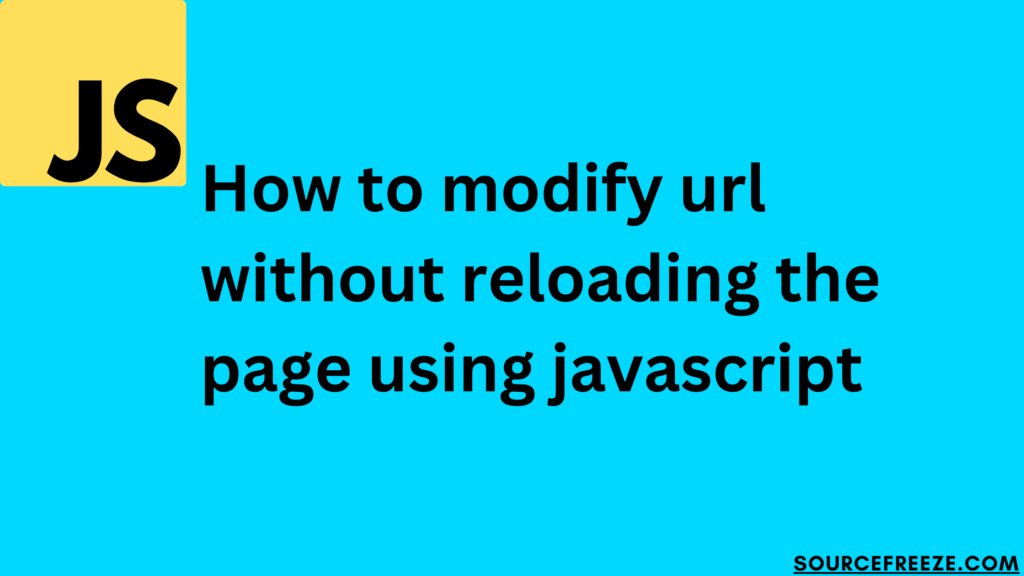
Using History API:
The History API in JavaScript provides methods to manipulate the browser’s history stack, allowing us to modify the URL without causing a full page refresh. This is particularly useful when creating smooth, dynamic user experiences in web applications.
Let’s consider a scenario where you have a simple HTML page with navigation links that, when clicked, change the URL without reloading the entire page. We’ll create an example where clicking different links will change the URL accordingly.
<!DOCTYPE html>
<html>
<head>
<title>URL Modification Example</title>
</head>
<body>
<h2>History API Example</h2>
<nav>
<ul>
<li><a href="#" onclick="changeURL('page1')">Page 1</a></li>
<li><a href="#" onclick="changeURL('page2')">Page 2</a></li>
<li><a href="#" onclick="changeURL('page3')">Page 3</a></li>
</ul>
</nav>
<div id="content">
<!-- Content will be dynamically loaded here -->
</div>
<script>
function changeURL(page) {
const newURL = `/${page}`;
history.pushState(null, null, newURL);
loadContent(page);
}
function loadContent(page) {
// Here, you'd fetch content based on 'page'
// For demonstration, let's just show the page name
document.getElementById('content').innerText = `Displaying ${page}`;
}
</script>
</body>
</html>
In this example, we have a basic HTML structure containing a heading, navigation links, and a content section. The nav
contains links to different pages (Page 1, Page 2, Page 3), each calling the changeURL()
function when clicked.
The changeURL()
function takes the page name as an argument, constructs a new URL based on that name, and uses history.pushState()
to modify the URL without reloading the page. Additionally, it calls the loadContent()
function to load content dynamically (this part could involve AJAX requests to fetch actual content).
For demonstration purposes, the loadContent()
function simply displays the name of the page in the content section.
Here’s the page as soon as it loads:
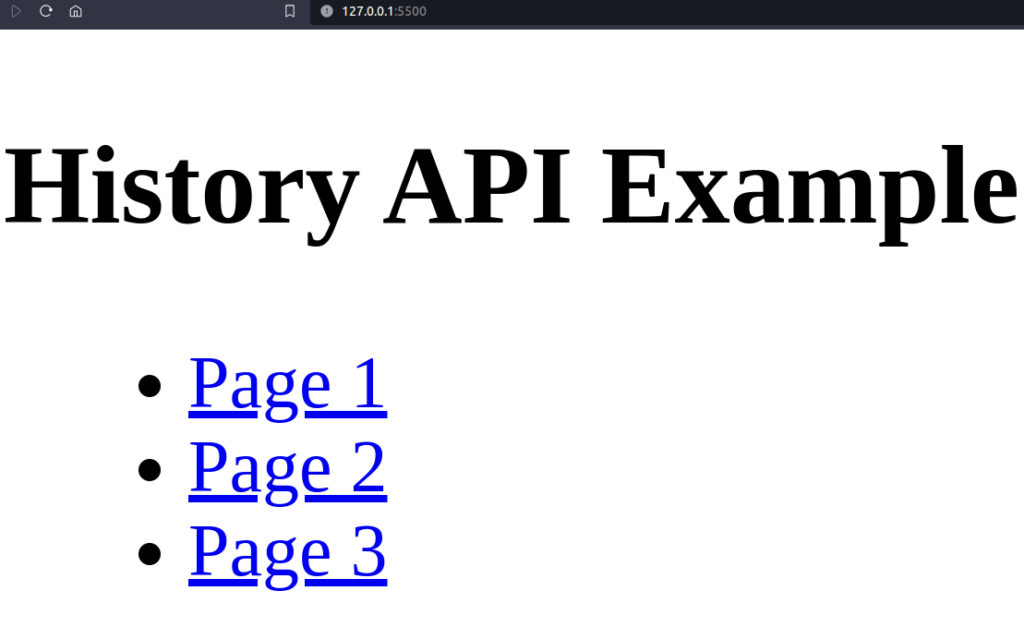
When we click ‘page 1’ the URL changes:
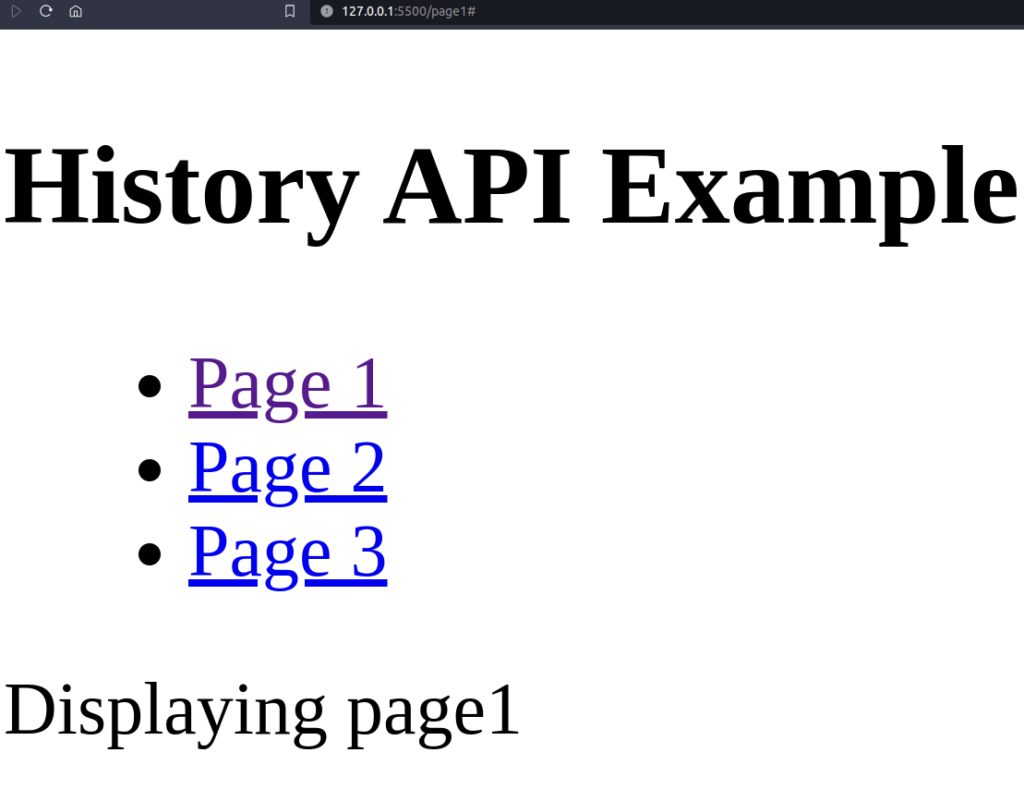
Same happens when we click ‘page 3’, the URL changes:
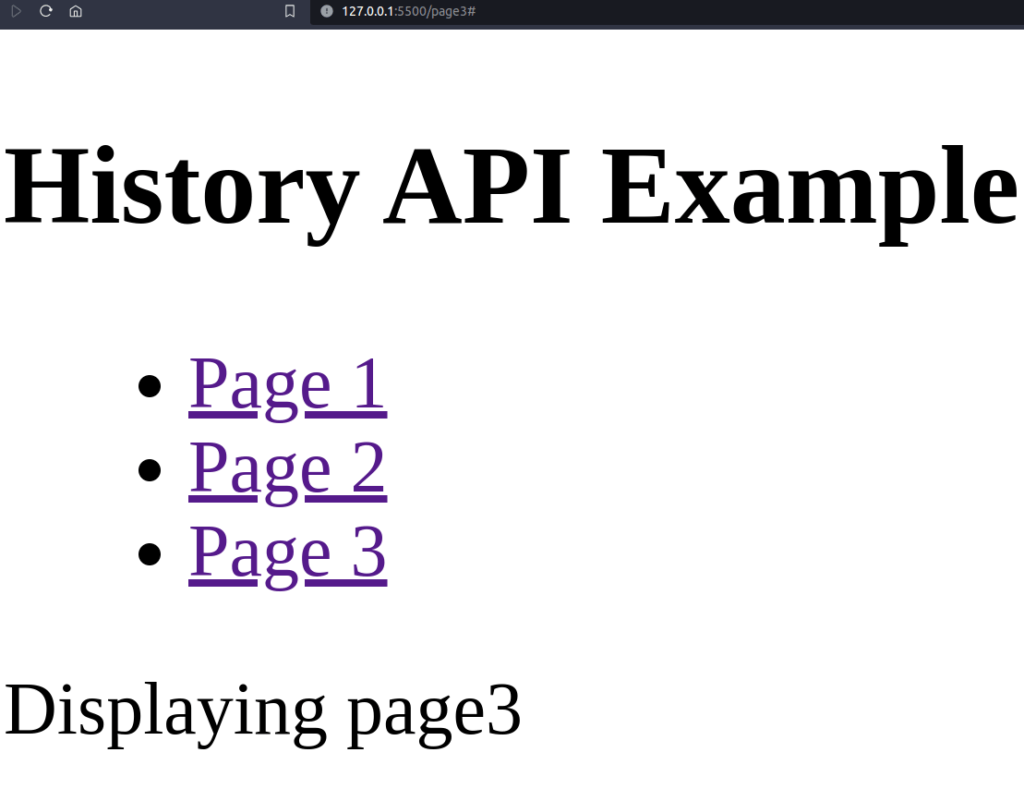
Using replaceState() Method:
Similar to pushState()
, the replaceState()
method enables us to change the URL displayed in the address bar without actually reloading the page. The difference lies in how it treats the browser’s history stack.
Here’s an extension of our previous example to showcase the replaceState()
method:
function replaceURL(page) {
const newURL = `/${page}`;
history.replaceState(null, null, newURL);
loadContent(page);
}
While pushState() adds a new entry to the history stack, replaceState() replaces the current entry with the new state. It alters the current URL without creating an additional history entry. This method can be particularly useful in certain scenarios, especially when you want to update the URL without creating a new history item.
In our example, clicking on a link calling replaceURL() would change the URL without adding a new entry to the history stack. This might be preferred in situations where you don’t want users to navigate back to the previous URL but still want to modify the displayed URL.
Let’s explore how the Location API can be used in conjunction with HTML and JavaScript to manipulate URLs without reloading the page.
Using Location API
The Location API in JavaScript provides access to the current URL and allows us to modify it. It includes properties and methods that help us interact with the URL of the current webpage.
Let’s consider a simple HTML structure where a button click changes the URL:
<!DOCTYPE html>
<html>
<head>
<title>Location API Example</title>
</head>
<body>
<h2>Location API Example</h2>
<button onclick="changeURL()">Change URL</button>
<script>
function changeURL() {
const newURL = '/newpage';
window.history.replaceState(null, null, newURL);
showCurrentURL();
}
function showCurrentURL() {
// Displaying the current URL
const currentURL = window.location.href;
alert(`Current URL: ${currentURL}`);
}
</script>
</body>
</html>
This HTML file contains a simple structure with an <h2>
heading and a button. When the button is clicked, it triggers the changeURL()
function.
Inside the changeURL()
function, we use window.history.replaceState()
to modify the URL to ‘/newpage’ without triggering a page reload. Additionally, it calls the showCurrentURL()
function.
The showCurrentURL()
function retrieves the current URL using window.location.href
and displays it in an alert for demonstration purposes. In a real application, you might use this information differently, such as updating specific content based on the modified URL.
Here’s the page in action:
Conclusion
In conclusion, JavaScript empowers developers to manipulate URLs effortlessly, enhancing user interactions on web applications. Through the exploration of the History and Location APIs, we’ve uncovered the ability to modify URLs without the page undergoing a complete reload.
Leave a Reply