When working with Next.js, controlling whether your application renders content on the server-side or client-side can significantly impact its performance and behaviour. Server-side rendering (SSR) can sometimes be unnecessary or less suitable for certain projects, and fortunately, Next.js provides ways to disable SSR when needed. In this blog, we’ll learn how to disable server side rendering in Nextjs
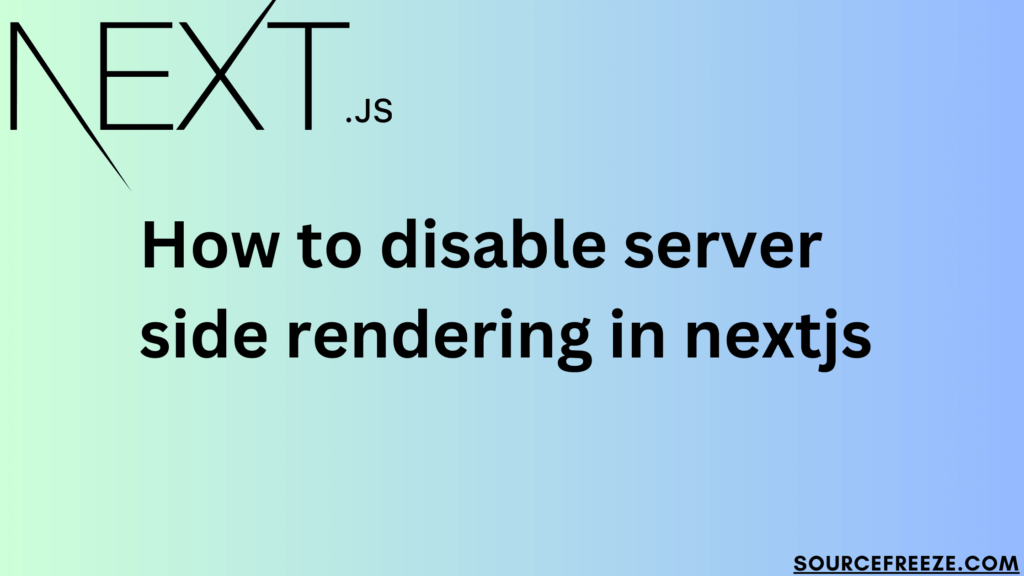
Understanding Rendering Methods in Web Development: CSR, SSR, and SSG
In the world of web development, how your web application renders content can significantly impact its performance, SEO, and user experience. Three primary rendering methods—Client-Side Rendering (CSR), Server-Side Rendering (SSR), and Static Site Generation (SSG)—play crucial roles in shaping the behavior and efficiency of modern web applications.
Client-Side Rendering (CSR):
CSR involves loading a web page’s framework and content entirely on the client’s side, typically using JavaScript. When a user requests a page, the server sends a basic HTML file, and then the client-side JavaScript framework takes over, fetching data from APIs and rendering the page content dynamically. This method enhances interactivity but might lead to slower initial loading times, especially on slower devices or networks.
Server-Side Rendering (SSR):
SSR generates the HTML for a web page on the server before sending it to the client. When a user requests a page, the server processes the request, fetches data, and generates an HTML page with populated content. This pre-rendered HTML is then sent to the browser, providing faster initial loading times and better SEO due to the presence of content in the HTML.
Static Site Generation (SSG):
SSG involves generating HTML files during the build time of the application, rather than on each request. This method pre-renders the entire website as static HTML files, which are then served to users. SSG offers the best performance as the content is already available and doesn’t require server processing for each request. However, it might not be suitable for highly dynamic content.
Let’s learn one approach using react-no-ssr todisable server-side rendering in Next.js:
Using react-no-ssr
react-no-ssr
is a package that allows components to be excluded from SSR. It ensures that certain parts of your application are only rendered on the client-side, bypassing SSR. Here’s how you can use it:
First, install the react-no-ssr package via npm or yarn:
npm install react-no-ssr
# or
yarn add react-no-ssr
Let’s say you have a component that you want to render only on the client-side. Import the NoSSR component from react-no-ssr:
import dynamic from 'next/dynamic';
import NoSSR from 'react-no-ssr';
const DynamicComponent = dynamic(() => import('../components/YourComponent'), {
ssr: false,
});
const YourPage = () => {
return (
<div>
{/* Components inside NoSSR will be rendered only on the client-side */}
<NoSSR>
<DynamicComponent />
</NoSSR>
{/* Other components will follow SSR rules */}
<YourOtherComponents />
</div>
);
};
export default YourPage;
The dynamic function from next/dynamic is used to dynamically load the component. Setting ssr to false ensures it’s excluded from SSR. The DynamicComponent will only be rendered on the client-side, while other components will follow the SSR rules of Next.js.
Using next/dynamic
next/dynamic is a Next.js function that allows for dynamic importing of components. It enables loading components asynchronously, which can be particularly useful when you want to control server-side rendering or optimize the initial loading of a page.
We’ll ensure if we have Next.js installed in our project. next/dynamic is a built-in Next.js feature, so no separate installation is required.
Let’s say you have a component named YourComponent that you want to render only on the client-side:
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/YourComponent'), {
ssr: false, // Set ssr to false to exclude from server-side rendering
});
const YourPage = () => {
return (
<div>
{/* Render the dynamically imported component */}
<DynamicComponent />
{/* Other components */}
</div>
);
};
export default YourPage;
The dynamic function from next/dynamic operates by asynchronously importing components at runtime rather than during the initial server render. When using dynamic, it takes two arguments: a function that imports the component and an options object that can include properties like ssr.
For instance, consider importing a component named YourComponent
. By employing dynamic
, this import happens on the client-side when the component is required, ensuring that it’s not bundled with the server-rendered HTML. The key here is the ssr: false
property within the options object passed to dynamic
, explicitly instructing Next.js to exclude this component from server-side rendering. Consequently, when the page loads, YourComponent
will be fetched and rendered exclusively on the client-side, optimizing performance by reducing initial load times. This fine-grained control over SSR helps in managing the balance between rendering components on the server for SEO and initial page loads while offloading others for better client-side interactivity.
NextJS has also added an official way to make this work, checkout there official docs on lazy loading here
Using a String (‘use client’)
In Next.js, a specific string at the top of a component file can be used as a workaround to trigger client-side rendering for that component. Here’s how you can do it:
'use client'
import React from 'react';
const YourComponent = () => {
return (
<div>
{/* Your component content */}
</div>
);
};
export default YourComponent;
Adding the string 'use client
‘ at the top of the component file acts as a signal to Next.js that this component should be rendered on the client-side.This technique exploits Next.js behavior to exclude certain components from server-side rendering.
Note: While this technique works, it’s considered more of a workaround and might not be officially documented or supported. It’s important to test thoroughly and ensure it aligns with the application’s requirements.
Conclusion
In this blog, we’ve explored methods to control how our Next.js apps load. We’ve uncovered next/dynamic, a tool letting us bring in components exactly when needed. Plus, we explored react-no-ssr, a way to say “hey, don’t server-render this one!” And also learnt about the simple but effective “use client” hint, making components show up just on the client-side.
Leave a Reply