JavaScript enables us to perform various actions on a webpage, and simulating the pressing of the Enter key is one of them. Whether it’s for form submissions or triggering specific functionalities, understanding how to programmatically press Enter can streamline user interactions. In this guide, we’ll explore how to press the Enter key programmatically in JavaScript:
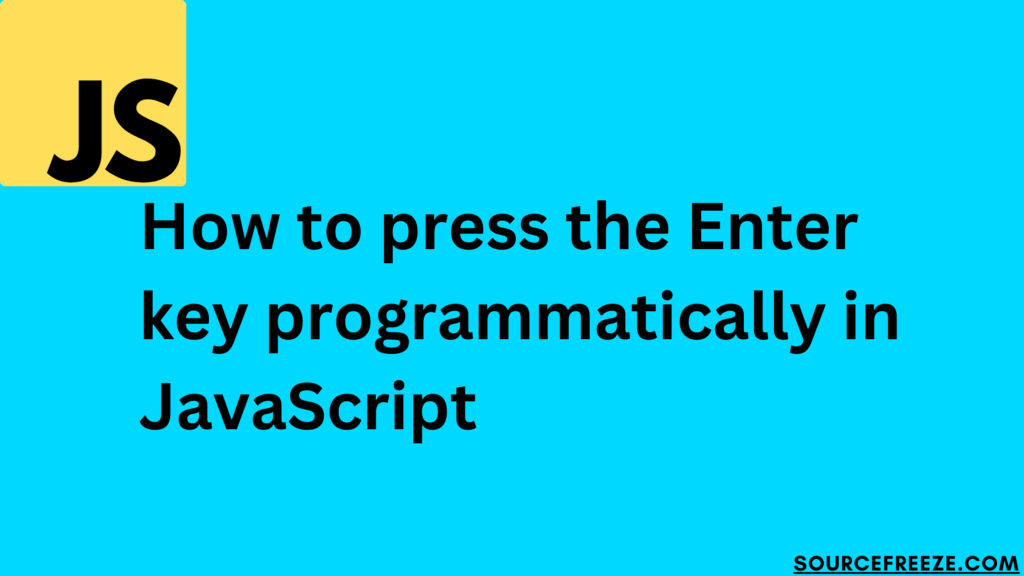
Simulate Enter key presses
To simulate pressing the Enter key programmatically in JavaScript, we can use the dispatchEvent
method along with creating a KeyboardEvent
. Here’s a simple example using HTML and JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Press Enter Key Demo</title>
</head>
<body>
<input type="text" id="textInput" placeholder="Press Enter!">
<button onclick="simulateEnter()">Simulate Enter</button>
<script>
function simulateEnter() {
// Retrieve the input element
const inputField = document.getElementById('textInput');
// Create a new keyboard event for 'Enter' key
const enterEvent = new KeyboardEvent('keydown', {
key: 'Enter',
code: 'Enter',
keyCode: 13,
which: 13,
});
// Dispatch the 'Enter' event on the input element
inputField.dispatchEvent(enterEvent);
}
</script>
</body>
</html>
This HTML snippet includes an input field and a button. Upon clicking the button, the simulateEnter JavaScript function triggers a simulated Enter key press by creating a new KeyboardEvent object. The event is dispatched on the input field, mimicking an actual Enter key press.
Event Listener on Input Field
We can listen for the Enter key press directly on an input field and execute a function when the Enter key is pressed by doing this:
<input type="text" id="textInput" placeholder="Press Enter" onkeydown="handleEnter(event)">
<script>
function handleEnter(event) {
if (event.key === 'Enter') {
// Perform desired action upon Enter key press
// For example: YourFunctionName();
}
}
</script>
By attaching the onkeydown
attribute to the input field, the handleEnter
function checks if the pressed key is ‘Enter’ and executes a specified function.
Using JavaScript’s addEventListener
Utilizing JavaScript’s addEventListener
allows us to listen for the Enter key press more dynamically:
<input type="text" id="textInput" placeholder="Press Enter!">
<button id="enterButton">Simulate Enter</button>
<script>
const inputField = document.getElementById('textInput');
const enterButton = document.getElementById('enterButton');
enterButton.addEventListener('click', simulateEnter);
function simulateEnter() {
// Create a new keyboard event for 'Enter' key
const enterEvent = new KeyboardEvent('keydown', {
key: 'Enter',
code: 'Enter',
keyCode: 13,
which: 13,
});
// Dispatch the 'Enter' event on the input element
inputField.dispatchEvent(enterEvent);
}
</script>
Binding Enter Key to Button Click
<input type="text" id="textInput" placeholder="Press Enter to click the button">
<button id="submitButton">Submit</button>
<script>
const inputField = document.getElementById('textInput');
const submitButton = document.getElementById('submitButton');
inputField.addEventListener('keydown', function(event) {
if (event.key === 'Enter') {
// Simulate button click on Enter key press
submitButton.click();
}
});
submitButton.addEventListener('click', function() {
// Your function to execute on button click
// For example: submitForm();
console.log('Button Clicked!');
});
</script>
In this example, when the Enter key is pressed inside the input field, it triggers a simulated click event on the submit button. After which the function associated with the button click event, submitForm()
in this case, gets executed.
Here’s how the output in the console will look like upon clicking 3 times:

Conclusion
In this blog, we’ve explored various methods to simulate the pressing of the Enter key programmatically in JavaScript. By leveraging simple yet powerful techniques, we’ve learned how to trigger actions, validate forms, and enhance user interactions seamlessly.
Through practical examples and straightforward explanations, we’ve discovered how to:
- Simulate Enter key presses.
- Dynamically execute functions upon Enter key events.
- Bind Enter key functionality to trigger button clicks.
Leave a Reply