In JavaScript, dealing with strings and arrays is fundamental to manipulating data. When faced with space separated strings, the need often arises to convert them into manageable arrays for further processing. In this guide, we will explore various methods to convert space separated strings to array in javascript
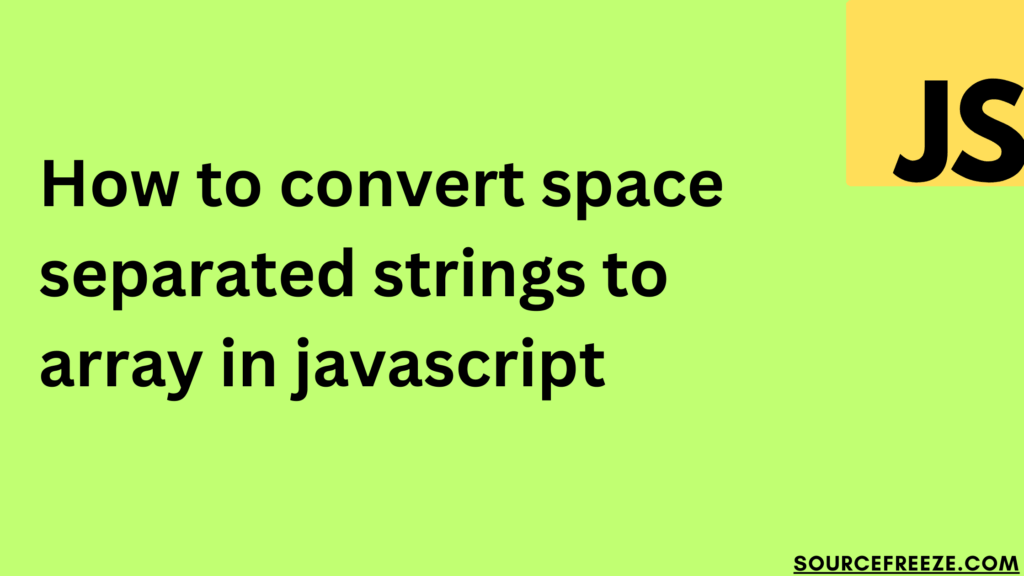
Using split() Function
The split()
method in JavaScript is a fundamental tool used to divide a string into an array of substrings based on a specified separator. This method empowers us to break down a string into smaller parts, making it easier to work with.
Basic Usage of split():
// Sample space-separated string
const spaceSeparatedString = "apple orange banana";
// Using split() to convert string to array
const stringToArray = spaceSeparatedString.split(" ");
console.log(stringToArray); // Output: ["apple", "orange", "banana"]
Here, the split() method is applied to spaceSeparatedString, splitting it into substrings whenever a space is encountered. These substrings are then stored as elements in the resulting array stringToArray.
The separator passed into the split() method determines where the string will be divided. For instance:
const sentence = "This is a sample sentence";
// Splitting by spaces
const splitBySpace = sentence.split(" ");
console.log(splitBySpace); // Output: ["This", "is", "a", "sample", "sentence"]
// Splitting by letters 'a'
const splitByLetterA = sentence.split("a");
console.log(splitByLetterA); // Output: ["This is ", " s", "mple sentence"]
When splitting by spaces, each word becomes an element in the resulting array. However, splitting by the letter ‘a’ separates the sentence wherever ‘a’ occurs, resulting in elements split at those points.
Utilizing Regular Expressions with split()
Regular expressions offer a more flexible approach for splitting strings based on complex patterns rather than just fixed characters. This empowers us to split strings using more intricate criteria.
Basic Use of Regular Expressions
// Sample string with varied separators
const complexString = "apple,orange;banana kiwi.apple";
// Using split() with regular expression to handle multiple separators
const usingRegex = complexString.split(/[ ,;.]+/);
console.log(usingRegex); // Output: ["apple", "orange", "banana", "kiwi", "apple"]
In this example, the split() method employs the regular expression [ ,;.]+ as the separator. This regex matches spaces, commas, semicolons, and periods. Consequently, the string complexString is split at any occurrence of these characters, resulting in an array of individual fruit names.
Trimming Elements in Split Arrays
Understanding the Initial String
// Sample string with leading and trailing spaces
const stringWithSpaces = " apple orange banana ";
The stringWithSpaces variable holds a string containing extra spaces at the beginning and end, surrounding the words “apple,” “orange,” and “banana.”
Splitting the String
// Splitting by spaces
const splitArray = stringWithSpaces.split(" ");
The split() method divides stringWithSpaces into an array of substrings, using spaces as the separator. However, due to the extra spaces in the string, the resulting array (splitArray) will contain empty elements at the beginning and end.
Trimming Each Element
// Trimming each element
const trimmedArray = splitArray.map((element) => element.trim());
The map() method iterates through each element in splitArray. For each element, the trim() method is applied. This method removes any leading or trailing spaces from the individual elements.
Conclusion:
Throughout this guide, we’ve learned various techniques for manipulating strings and arrays in JavaScript:
- Using
split()
Function: We explored how thesplit()
method efficiently divides strings into arrays based on specified separators, facilitating the transformation of space-separated strings into manageable arrays.
- Utilizing Regular Expressions with
split()
: By integrating regular expressions with thesplit()
method, we learned how to split strings based on complex patterns, enabling versatile handling of varied separators.
- Trimming Elements in Split Arrays: We detailed the process of refining arrays obtained after splitting strings, specifically focusing on the
trim()
method to eliminate leading and trailing spaces from individual elements.
Leave a Reply