In JavaScript, string manipulation stands as a fundamental skill, empowering developers to use the language’s capabilities effectively. Throughout this comprehensive guide, we’ll explore this process, dissecting JavaScript code snippets and learning their functionality to split a string on Capital Letters using JavaScript.
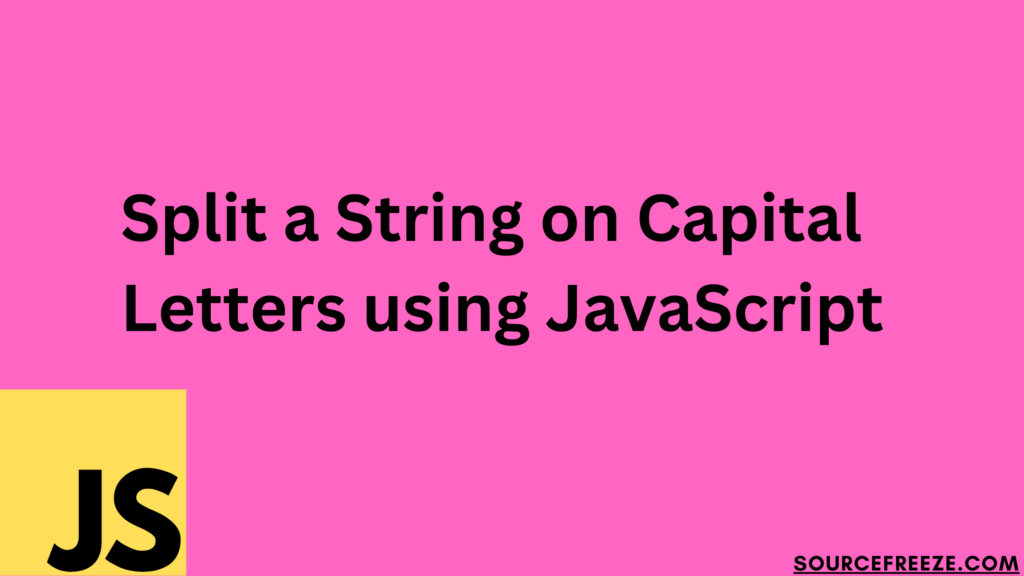
First, let’s discuss the methodology behind this operation.
Understanding the split() Method in JavaScript
The split()
method, used for string manipulation in JavaScript, allows us to divide a string into an array of substrings based on a specified separator.
Consider a string with a sequence of words in camelCase or PascalCase, where each word begins with a capital letter. Utilizing the split()
method in conjunction with a regular expression tailored to identify capital letters, we can effectively segment the string at these points, extracting meaningful components.
Let’s illustrate this process with a code snippet:
const text = "SplittingStringsOnCapitalLetters";
const result = text.split(/(?=[A-Z])/);
console.log(result);
In this example, the split() method employs a regular expression (?=[A-Z]). Indicating that the split should occur before any capital letter. Running this code would generate an array containing individual words derived from the initial string, separated at the capital letters:
Output
["Splitting", "Strings", "On", "Capital", "Letters"]
When working with strings that contain blank spaces or whitespace characters, managing these elements becomes crucial. JavaScript provides the trim()
method, which proves invaluable in handling leading and trailing whitespace within strings.
Handling Strings Starting with Whitespace
Identifying the Issue
Consider a scenario where the input string begins with whitespace:
const textWithSpace = " StartingWithSpaceSplitOnCaps";
const resultWithoutTrim = textWithSpace.split(/(?=[A-Z])/);
console.log(resultWithoutTrim);
When attempting to split this string based on capital letters without applying trim()
, the outcome might not align with our expectations due to the leading whitespace. The resulting array might contain an additional empty string or a segment not correctly formed:
["", "Starting", "With", "Space", "Split", "On", "Caps"]
The initial empty string in the resulting array emerges from the leading whitespace, leading to an undesired element in the segmented array.
Managing Whitespace with trim() Method
The trim()
method, an essential tool in string manipulation, facilitates the removal of whitespace characters from both ends of a string. These whitespace characters include spaces, tabs, and newline characters, among others.
Let’s incorporate trim() into our previous code snippet to handle potential whitespace around the string before splitting it based on capital letters:
const textWithSpace = " StartingWithSpaceSplitOnCaps";
const trimmedText = textWithSpace.trim();
const resultWithTrim = trimmedText.split(/(?=[A-Z])/);
console.log(resultWithTrim);
Applying trim() to textWithSpace eliminates the leading whitespace before executing the split() operation based on capital letters. Consequently, the resulting array reflects the segmented words accurately:
["Starting", "With", "Space", "Split", "On", "Caps"]
By incorporating trim()
before splitting the string, we ensure that leading whitespace doesn’t interfere with the intended segmentation based on capital letters.
Conclusion:
There you go! Now you’ve got a good handle on handling words in JavaScript! We’ve seen how split() helps with word division and how trim() tidies up spaces. With these simple tricks, working with words becomes much easier in JavaScript.
Leave a Reply