In JavaScript, comparing dates is crucial for various applications. Whether it’s for scheduling, validation, or conditional operations, understanding how to compare dates with the current date is fundamental. We’ll explore several methods to compare date with current date in JavaScript.
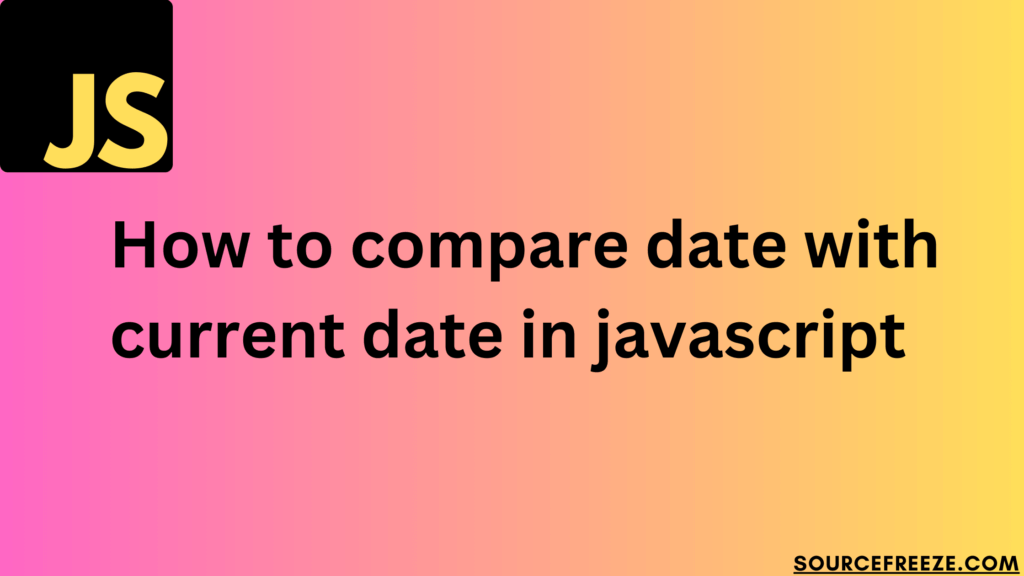
Using the Date Object
The Date object in JavaScript is pivotal for working with dates. By creating instances of Date, we can perform comparisons easily.
// Create a date object for the current date
const currentDate = new Date();
// Create another date object for comparison
const otherDate = new Date('2025-09-03');
// Compare the two dates
if (currentDate > otherDate) {
console.log('The current date is after the specified date.');
} else if (currentDate < otherDate) {
console.log('The current date is before the specified date.');
} else {
console.log('Both dates are equal.');
}
This code snippet utilizes JavaScript’s Date object to create two instances: currentDate
, representing the current date and time, and otherDate
, set to September 3, 2025. It employs conditional statements to compare these dates. If currentDate
is later than otherDate
, it will log “The current date is after the specified date.” Conversely, if currentDate
is earlier than otherDate
, it logs “The current date is before the specified date.” In the scenario where both dates are identical, the output will be “Both dates are equal.” Here we get:
The current date is before the specified date.
Using getTime() Method
Another approach involves utilizing the getTime()
method, which returns the number of milliseconds since January 1, 1970. This enables direct numerical comparisons between dates.
// Create a date object for the current date
const currentDate = new Date();
// Create another date object for comparison
const otherDate = new Date('2025-09-03');
// Get milliseconds for current date
const currentTime = currentDate.getTime();
// Get milliseconds for another date
const otherTime = otherDate.getTime();
// Compare the milliseconds
if (currentTime > otherTime) {
console.log('The current date is after the specified date.');
} else if (currentTime < otherTime) {
console.log('The current date is before the specified date.');
} else {
console.log('Both dates are equal.');
}
This code snippet starts by creating two Date objects: currentDate
, representing the current date and time, and otherDate
, set to September 3, 2025. Additionally, it calculates the number of milliseconds since January 1, 1970, for both currentDate
and otherDate
using the getTime()
method, storing these values in currentTime
and otherTime
, respectively.
The subsequent conditional statements compare these calculated millisecond values. If currentTime
exceeds otherTime
, it logs “The current date is after the specified date.” Conversely, if currentTime
is less than otherTime
, it outputs “The current date is before the specified date.” In the event where both millisecond values are identical, it logs “Both dates are equal.”
We’ll get the same output as before:
The current date is before the specified date.
Conclusion:
In this blog, we explored two primary methods for comparing dates in JavaScript. The first method involved direct Date object comparison, employing standard operators to evaluate the relationship between dates. The second method utilized milliseconds obtained through the getTime() method, allowing for a numerical comparison between the dates’ underlying values.
Leave a Reply