In this guide, we’ll delve into the methods to hide and show HTML elements by ID using JavaScript, focusing specifically on targeting elements by their IDs. This technique is essential for interactive web development and enhances user experience.
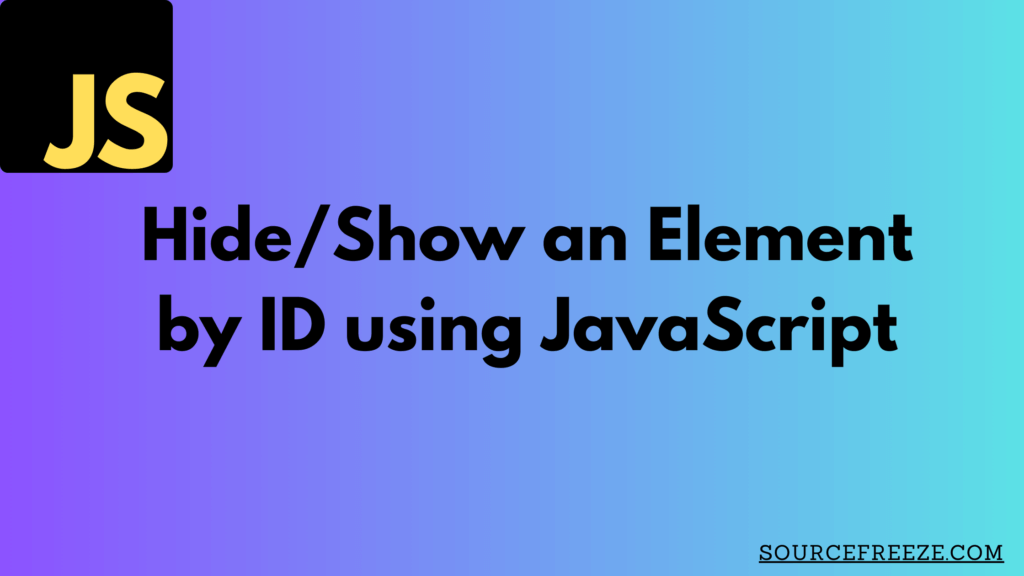
JavaScript offers several methods to manipulate the visibility of elements based on their IDs. Let’s explore two primary methods in detail:
Method 1: Hide/Show Element by getElementById and CSS Display Property
The getElementById
method is a fundamental JavaScript function used to retrieve an HTML element based on its unique ID. Paired with the manipulation of the CSS display
property, this method enables the hiding and showing of elements.
Implementation:
// JavaScript Code
const element = document.getElementById('elementID');
// To Hide:
element.style.display = 'none';
// To Show:
element.style.display = 'block'; // or 'inline', 'flex', depending on the element's default display type
Explanation:
The getElementById function retrieves the element with the specified ID from the DOM (Document Object Model). Subsequently, changing the display property allows us to toggle the visibility of the element. Setting display to ‘none’ hides the element, while setting it to its default value (e.g., ‘block’) shows the element again.
Method 2: Hide/Show Element using classList to Toggle Classes
Another approach involves manipulating CSS classes through the classList
property. This method utilizes pre-defined CSS classes to control the visibility of elements.
Implementation:
// JavaScript Code
const element = document.getElementById('elementID');
// To Hide:
element.classList.add('hidden');
// To Show:
element.classList.remove('hidden');
Explanation:
Here, we’re toggling a pre-defined CSS class named ‘hidden’ using classList
. This ‘hidden’ class includes styling rules to hide the element by adjusting its visibility or display properties. Adding or removing this class dynamically alters the element’s visibility.
In the upcoming segment, we’ll explore additional techniques to manipulate element visibility using JavaScript, elaborating on further methods and their nuances.
Method 3: Hide/Show Element using visibility Property
The visibility
property provides another way to control the visibility of elements, offering more nuanced control compared to the display
property.
Implementation:
// JavaScript Code
const element = document.getElementById('elementID');
// To Hide:
element.style.visibility = 'hidden';
// To Show:
element.style.visibility = 'visible';
Explanation:
Unlike display
, changing the visibility
property to 'hidden'
hides the element while still occupying space in the layout. Setting it to 'visible'
restores the visibility without affecting the layout structure.
Method 4: Hide/Show Element Using hidden Attribute
HTML5 introduced the hidden
attribute, offering a simple way to hide elements directly within the markup.
Implementation:
<!-- HTML Markup -->
<div id="elementID" hidden>
This element is hidden by default.
</div>
// JavaScript Code
const element = document.getElementById('elementID');
// To Show:
element.removeAttribute('hidden');
// To Hide:
element.setAttribute('hidden', true);
The JavaScript manipulation of this attribute involves using setAttribute('hidden', true)
to hide the element or removeAttribute('hidden')
to reveal it. This attribute operates independently of CSS, making it a convenient and effective method for simple element visibility control.
This method can be especially useful for elements that need to be initially hidden and selectively shown based on user interactions or specific events.
Conclusion:
In summary, the methods covered to show and hide HTML elements by ID using JavaScript provide various options catering to different use cases for hide/shoe elements.
getElementById
with CSS Display Property: This method alters thedisplay
property, toggling between hiding and showing the element while affecting its layout.- Using
classList
to Toggle Classes: Manipulating CSS classes allows for dynamic visibility control without directly modifying style properties. visibility
Property: Thevisibility
property offers nuanced control by hiding elements while maintaining their layout space.- Using
hidden
Attribute: HTML5’shidden
attribute provides a straightforward means to hide elements directly within the markup, independent of CSS styling.
Leave a Reply