When we talk about get elements by aria-label
using JavaScript, there are several methods at our disposal. Let’s see into three primary methods and explore the first one in detail but first What is an aria-label
?
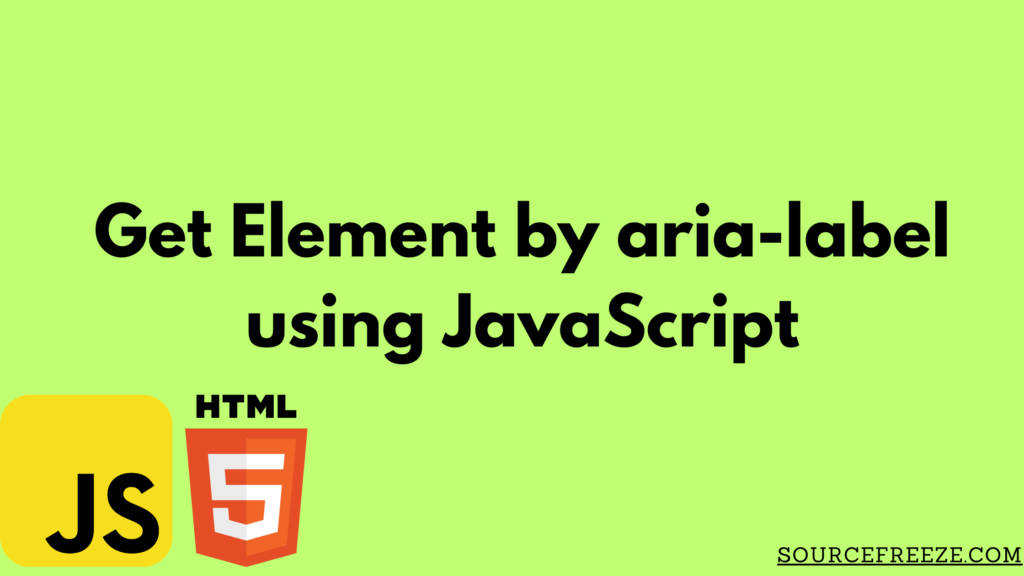
What is aria-label?
In the world of websites and apps, elements like buttons, images, or links often need labels to tell us what they do. But sometimes, these elements don’t have visible text labels. That’s where “aria-label” comes in.
Think of “aria-label” as a special tag we can give to things on a webpage to describe what they are or what they do. It’s like a hidden label that helps computers and assistive technologies, like screen readers, understand what an element is all about.
For instance, imagine a button with an icon instead of words. The “aria-label” acts like a secret message attached to that button, telling anyone who can’t see the icon what it’s there for.
Now let’s explore how to get Element by aria-label using JavaScript using the following methods:
- Using
querySelector
for an Exact Match - Partial Match of
aria-label
Value - Narrowing Down with Specific Element Types
Using querySelector
for an Exact Match
To begin with, one effective way to target an element with a precise aria-label
is by using the querySelector
method. This method fetches the first element in the document that matches the provided selector.
Let’s consider an example scenario:
<body>
<button aria-label="Dismiss">X</button>
<button aria-label="Submit">Submit</button>
</body>
If we wish to retrieve the button element with the aria-label
attribute set to “Dismiss,” here’s how we accomplish it:
const dismissButton = document.querySelector('[aria-label="Dismiss"]');
console.log(dismissButton); // ๐๏ธ button element with aria-label="Dismiss"
This code snippet uses the attribute selector [aria-label="Dismiss"]
within querySelector
to pinpoint the button element precisely.
By employing this method, we ensure we fetch the exact element matching the specified aria-label
attribute value.
Partial Match of aria-label
Value
Sometimes, we might need to find elements based on a partial match of their aria-label
attribute. JavaScript provides selectors that cater to these scenarios:
- Starts with (^=)
- Ends with ($=)
- Contains (*=)
Suppose we have the following HTML elements:
<div aria-label="Notification: Error">Error Message</div>
<button aria-label="Close Notification">X</button>
<button aria-label="Open Menu">Menu</button>
Starts with (^=)
Let’s say we want to target elements whose aria-label
starts with “Notif.” We use the ^=
selector to accomplish this:
const notificationElement = document.querySelector('[aria-label^="Notif"]');
console.log(notificationElement); // ๐๏ธ div with aria-label="Notification: Error"
By using [aria-label^="Notif"]
, we locate elements where the aria-label
attribute starts with “Notif.”
Ends with ($=) and Contains (*=)
Similarly, if we aim to find elements where the aria-label
attribute ends with or contains specific strings, we use the $=
and *=
selectors, respectively.
Let’s move on to the third method:
Narrowing Down with Specific Element Types
In addition to targeting elements by their aria-label
attributes, we can further refine our selection by specifying the element type.
For instance, consider the following HTML snippet:
<button aria-label="Close Notification">X</button>
<div aria-label="Open Menu">Menu</div>
<button aria-label="Submit">Submit</button>
Suppose we’re interested in specifically selecting button elements based on their aria-label
attributes.
Narrowing Down Buttons by aria-label
To achieve this, we use a selector that combines the button element type with the aria-label
attribute condition:
const closeButton = document.querySelector('button[aria-label="Close Notification"]');
console.log(closeButton); // ๐๏ธ button with aria-label="Close Notification"
By employing this selector (button[aria-label="Close Notification"]
), we precisely target the button element with the specified aria-label
.
This method enables us to not only focus on aria-label
attributes but also narrow down the elements by their specific types, ensuring a more refined selection process
Conclusion
get elements by their aria-label
attributes using javascript provide a powerful way to access specific components, especially in scenarios where elements might lack distinctive identifiers.
Throughout this discussion, we’ve uncovered three fundamental methods:
- Exact Match using
querySelector
: Precisely fetches elements based on an exactaria-label
attribute value. - Partial Matching(^=, $=, *=): Enables searching for elements with
aria-label
attributes that start with, end with, or contain specific strings. - Narrowing Down with Specific Element Types: Allows refining element selection by combining
aria-label
conditions with specific element types.
By leveraging these methods, we can efficiently navigate and interact with elements in the Document Object Model (DOM), enhancing accessibility and functionality within web applications.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply