There are several ways to remove all classes from an element in JavaScript. We will see more details in this guide first we have three methods below to remove all classes from an element that will see by one.
classList
removeAttribute
className
.
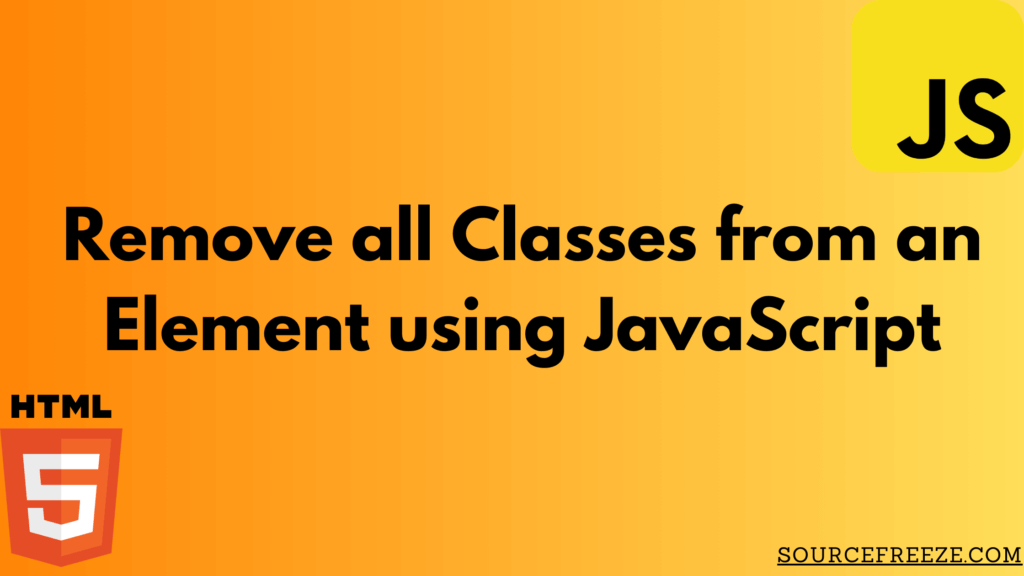
1. Using classList Method to remove classes:
The classList
property provides methods to add, remove, or toggle classes on an element. To remove a class, we’ll specifically use the remove()
method.
const element = document.getElementById('yourElementId');
element.classList.remove('classNameToRemove');
Explanation:
- Step 1: Select the target element using
getElementById
or another selection method. - Step 2: Access the element’s
classList
property. - Step 3: Use the
remove()
method ofclassList
, passing the class name we want to remove as an argument.
This method is straightforward and efficient. However, keep in mind that it’s only available in modern browsers.
2. Using removeAttribute Method to modify classes:
The removeAttribute
method enables us to remove attributes from an HTML element, including the class
attribute.
const element = document.getElementById('yourElementId');
element.removeAttribute('class');
Explanation:
- Step 1: Identify the target element using
getElementById
or similar. - Step 2: Apply the
removeAttribute
method, specifying'class'
as the attribute we want to remove.
This method is direct and works well for scenarios where you want to remove all classes associated with an element. However, it completely removes the class attribute, so use it judiciously based on your requirements.
3. Using className Property to remove or set desired classes:
The className
property provides a straightforward way to modify or remove classes from an element.
Removing All Classes:
const element = document.getElementById('yourElementId');
element.className = '';
Explanation:
- Step 1: Identify the target element using
getElementById
or similar. - Step 2: Assign an empty string
''
to theclassName
property of the element.
By setting className
to an empty string, all classes associated with the element are effectively removed.
Assign a Single Class and Remove Others:
const element = document.getElementById('yourElementId');
element.className = 'desiredClass';
Explanation:
- Step 1: Locate the element using
getElementById
or a similar method. - Step 2: Assign the
className
property to the desired class name that you want to set.
This method seems simple, but it replaces all existing classes with the specified ones.
Conclusion
In this blog, we explored three distinct methods for remove all classes from an element in JavaScript. We discussed the implementation of these methods using classList
, removeAttribute
, and the className
property.
classList
: Offers specific methods likeremove()
to remove individual classes.removeAttribute
: Directly removes the entireclass
attribute from the element.className
Property: Provides flexibility by either removing all classes or assigning a single class while removing others.
Each method has its merits and specific use cases, allowing developers to choose the most suitable approach based on their requirements.
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply