Maps, introduced in ES6, are versatile data structures that store key-value pairs. Sorting a Map in JavaScript can be quite useful when you need your data in a particular order. Let’s dive into this together and explore how to sort a map in JavaScript in this tutorial using a step-by-step approach.
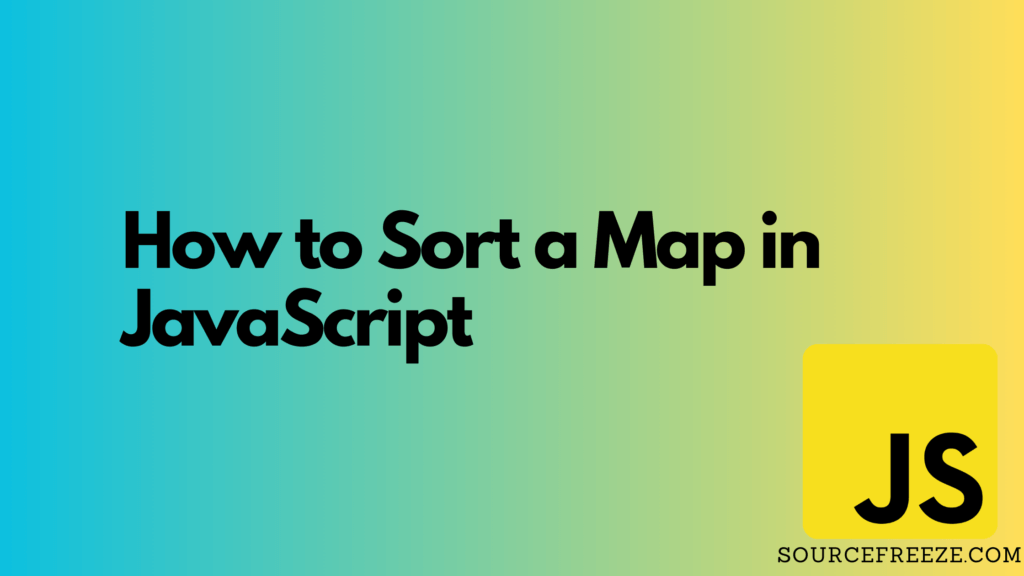
We will be exploring two methods in this article to sort a map in javascript.
Method 1: Sorting with Object.entries() and Array.sort():
Imagine our Map is full of fruits and their prices, but they’re all jumbled up. We can use Object.entries() to turn the Map into a list of lists, like this:
const fruits = new Map([
['apple', 10],
['banana', 15],
['orange', 20],
]);
const messyList = Object.entries(fruits);
console.log(messyList); // Output: [['apple', 10], ['banana', 15], ['orange', 20]]
Now, we use the formula (a, b) => a[1] – b[1] to sort the list. This formula simply compares the prices of two fruits and decides which one comes first:
- If the first fruit is cheaper, it comes before the second.
- If the second fruit is cheaper, it comes before the first.
- If they have the same price, their order stays the same.
const sortedList = messyList.sort((a, b) => a[1] - b[1]);
console.log(sortedList); // Output: [['apple', 10], ['banana', 15], ['orange', 20]]
Rebuilding the Organized Map:
Finally, we turn the sorted list back into an organized Map:
const organizedFruits = new Map(sortedList);
console.log(organizedFruits); // Output: Map {apple => 10, banana => 15, orange => 20}
This method is simple and easy to understand, even for beginners. It uses plain numbers instead of complicated logic, making it easier to remember and apply.
Remember:
- We can sort a Maps by key or value. Just change the a[1] and b[1] in the formula to the index of the element you want to sort by.
- This method is great for sorting small to medium-sized Maps. For very large Maps, other methods might be more efficient to sort a map in javascript.
Method 2: Using Custom Sorting Logic with Array.from() and Map() Constructor
Step 1: Create a Sample Map
let myMap = new Map();
myMap.set('John', 35);
myMap.set('Alice', 29);
myMap.set('Bob', 42);
Step 2: Convert Map Entries to Array, Apply Custom Sorting Logic
This time, we’ll use a custom sorting logic to sort the Map entries:
let sortedArray = Array.from(myMap)
.sort(([keyA, valueA], [keyB, valueB]) => {
// Custom sorting logic based on values (age in this case)
return valueA - valueB;
});
Step 3: Reconstruct a Sorted Map
Reconstruct a Map from the sorted array of entries:
let sortedMap = new Map(sortedArray);
Step 4: Display the Sorted Map
Loop through the sorted map and display the key-value pairs:
for (let [key, value] of sortedMap) {
console.log(`${key}: ${value}`);
}
Output:
Alice: 29
John: 35
Bob: 42
Conclusion:
By understanding these techniques, we can effectively sort maps in JavaScript based on keys or apply custom sorting based on values. Feel free to utilize these methods as per your sorting requirements!
Thanks for stopping by! Check out more from Source Freeze here!
Leave a Reply