here in this tutorial, we can see how to get a filename from the URL in javascript.
How to Get a filename from URL in JavaScript
to get a filename from URL in javascript call the following method URL substring then the URL of the last index of /
plus 1 we will get the exact filename.
Usually, the file name will be in the last part of the URL, sourcefreeze.com/filename.png here filename.png is the file name first to get the URL we can use window.location.pathname
then try to extract the filename using url.substring
method by providing url.lastIndexOf('/')+1
.
Please refer to the exact code below to get the filename, here first we are checking URL is valid not if valid then we are getting the correct filename else to return the empty string.
function getFileName(url) {
if (url) {
let filename = url.substring(url.lastIndexOf('/') + 1);
return filename;
}
return "";
}
How to get the filename from URL without extension
to get a filename from URL in javascript without extension call the following method URL substring then the URL of the last index of /
plus 1 then URL last index of .
will remove the extension of the file so we will get the exact filename without the extension.
In some cases, we need to get the filename from the URL without an extension for example sourcefreeze.com/filename.png is a URL we need the filename only without .png, please find the code to get the file name without an extension below.
we are using the same approach to get the file name but just adding the url.lastIndexof(".")
to remove the png or file extension.
let url = "http://sourcefreeze.com/filename.png";
let filename = url.substring(url.lastIndexOf("/") + 1, url.lastIndexOf("."));
How to get the file name from URL using Regex
To get the file name in the javascript using regex, we can use the javascript regex exec method. it will return the array of values which contains the filename, index, and input group details in the array of 0 positions we will get the exact file name.
const fileName = /[^/]*$/.exec('https://sourcefreeze.com/wp-content/uploads/2022/01/source_freeze.png')
console.log(fileName[0]) // source_freeze.png
console.log(fileName) // will return the filename, index, input group details
in the first array of 0, we will get the exact file name plus group, index, and input details correspondingly.
How to get the file name from URL without extension using Regex:
The below code is used to get the file name from the URL without extension using the regex method, here we just getting dividing the name /
then dividing with .
after that get the first part of the array so we will get the exact file name.
let url = "https://sourcefreeze.com/wp-content/uploads/2022/01/source_freeze.png";
let filename = url.match(/([^\/]+)(?=\.\w+$)/)[0];
console.log(filename);
Please let us know if any questions in the comments.
Also, refer to our previous tutorial how to convert array to comma separated strings in javascript
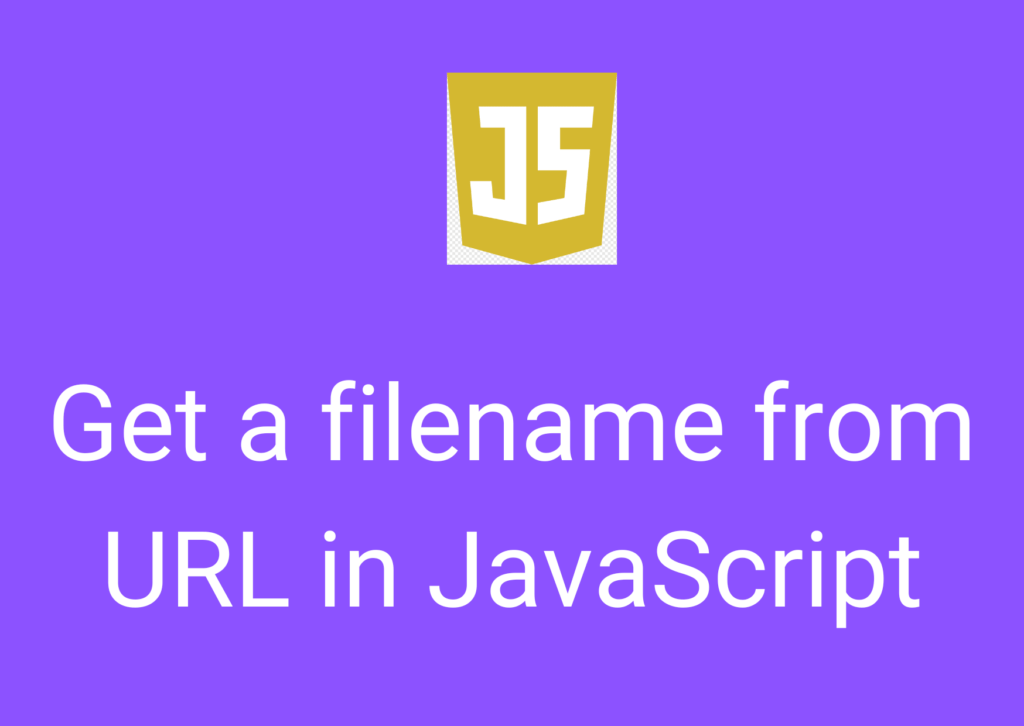
Leave a Reply